Chapter 2: Python and Essential Libraries
2.5 Scikit-learn for Machine Learning
Scikit-learn is an open-source library for machine learning in Python that is extensively used in the field. It provides a plethora of supervised and unsupervised learning algorithms to help users build predictive models. The library is designed to be user-friendly and consistent, with a simple interface in Python. It is built on top of NumPy, SciPy, and Matplotlib, which are other popular Python libraries.
In this section, we will cover the basics of Scikit-learn, including data preprocessing, creating a model, training the model, making predictions, and evaluating the model. Preprocessing involves cleaning and transforming the data to make it suitable for machine learning algorithms.
Creating a model involves selecting an appropriate algorithm and setting its parameters. Training the model involves feeding the data to the algorithm and adjusting its parameters to achieve the desired outcome.
Making predictions involves using the trained model to predict the outcome of new data. Evaluating the model involves measuring its performance on a test dataset and fine-tuning it further if necessary. By following these steps, users can gain a better understanding of Scikit-learn and its applications in the field of machine learning.
2.5.1 Installation
Before we start, make sure you have Scikit-learn installed. If you haven't installed it yet, you can do so using pip:
pip install scikit-learn
2.5.2 Importing Scikit-learn
To use Scikit-learn in your Python program, you first need to import it:
from sklearn import preprocessing, model_selection, linear_model, metrics
2.5.3 Data Preprocessing
Scikit-learn provides a variety of utilities for data preprocessing, which can help refine and optimize the data before analysis. One particularly useful tool is the StandardScaler
. This scaler works by standardizing the features of the data, which involves removing the mean and scaling the data to unit variance.
By doing this, the data is transformed to a normal distribution, which can be more easily analyzed using various machine learning algorithms. In addition to the StandardScaler
, Scikit-learn also provides other data preprocessing tools, such as the MinMaxScaler
and the RobustScaler
. These scalers are useful for different situations, such as scaling data to a specific range or handling outliers.
By utilizing these preprocessing tools, you can ensure that your data is optimized and ready for analysis, leading to more accurate and informative results.
Example:
from sklearn import preprocessing
import numpy as np
# Create a StandardScaler
scaler = preprocessing.StandardScaler()
# Fit the StandardScaler to the data
data = [[0, 0], [0, 0], [1, 1], [1, 1]]
scaler.fit(data)
# Transform the data
scaled_data = scaler.transform(data)
print(scaled_data)
2.5.4 Creating a Model
Scikit-learn is a useful library for machine learning enthusiasts. It provides a wide range of machine learning models that can be utilized for different tasks. These models can be easily imported and used in your projects.
There are many models to choose from, such as linear regression, decision trees, random forests, and more. In fact, linear regression models can be created quite easily with Scikit-learn, and can be customized to better suit your specific needs and requirements. So, whether you're a beginner or an experienced machine learning practitioner, Scikit-learn is definitely worth checking out.
Example:
Scikit-learn provides a variety of machine learning models that you can use. For example, you can create a linear regression model like this:
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
2.5.5 Training the Model
Once you have a model, you can train it on your data using the fit
method. Training the model involves passing it your training data multiple times in order to improve its accuracy. During each pass, the model makes predictions on the training data and compares them to the actual values.
It then uses this comparison to adjust the parameters of the model in order to better fit the data. The fit
method also allows you to specify a validation set, which the model can use to evaluate its performance on data that it has not seen during training.
By iterating over the training data multiple times and making adjustments along the way, the model can learn to make increasingly accurate predictions on new data.
Example:
You can train the model on your data using the fit
method:
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
# Train the model
X = [[0, 0], [1, 1]]
y = [0, 1]
model.fit(X, y)
2.5.6 Making Predictions
Once the model is trained, you can use it to make predictions on new data. This can be an incredibly valuable tool in a variety of fields, from finance to healthcare to marketing. Once you have the model up and running, you can use it to generate insights that can help you make better decisions and achieve better outcomes.
The more data you feed into the model, the more accurate it will become, as it is able to learn from experience and adjust its predictions accordingly. So don't be afraid to experiment and try new things--the possibilities are endless with a well-trained predictive model.
Example:
After the model is trained, you can use it to make predictions on new data:
# Make predictions
X_new = [[2, 2]]
y_new = model.predict(X_new)
print(y_new)
2.5.7 Evaluating the Model
Scikit-learn is a powerful Python library that offers a wide array of functions to evaluate the performance of your machine learning models. In addition to the mean squared error, which is commonly used to assess the accuracy of a model, Scikit-learn provides other evaluation metrics such as R-squared, precision, recall, and F1 score.
Understanding these metrics and how to use them properly is crucial for building effective machine learning models that can handle real-world problems. Furthermore, Scikit-learn also provides tools for data preprocessing, feature selection, model selection, and model optimization, which are essential steps in the machine learning pipeline.
With Scikit-learn, you can streamline your machine learning workflow and make the most of your data.
Example:
Scikit-learn provides several functions to evaluate the performance of a model, such as the mean squared error:
from sklearn import metrics
# Calculate the mean squared error of the predictions
y_true = [1]
y_pred = model.predict(X_new)
mse = metrics.mean_squared_error(y_true, y_pred)
print(mse)
2.5.8 Advanced Scikit-learn Features
Cross-Validation
Cross-validation is a powerful statistical method that is used to estimate the skill of machine learning models. It is a commonly used technique in the field of applied machine learning, and is particularly useful when comparing and selecting models for a given predictive modeling problem. One of the main advantages of cross-validation is that it is easy to understand and implement, even for those who are not experts in the field of machine learning.
The results obtained from cross-validation tend to have lower bias than other methods, making it a highly reliable technique for evaluating the performance of machine learning models. Overall, cross-validation is an indispensable tool for anyone working in the field of machine learning, and is sure to become even more important as the field continues to evolve and expand in the coming years.
Example:
from sklearn.model_selection import cross_val_score
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
# Perform 5-fold cross-validation
scores = cross_val_score(model, X, y, cv=5)
# Print cross-validation scores
print(scores)
Hyperparameter Tuning
Machine learning models are parameterized so that their behavior can be tuned for a given problem. These parameters can be modified to achieve better accuracy or to optimize other metrics that are important for a given application.
While some parameters have clear and intuitive interpretations, others can be more subtle and require a deeper understanding of the underlying model. This means that finding the best combination of parameters can be treated as a search problem that requires careful consideration of the trade-offs between different choices.
Scikit-learn provides two methods for automatic hyperparameter tuning: Grid Search and Randomized Search. Grid Search exhaustively searches the hyperparameter space for a given set of parameters, while Randomized Search samples randomly from the hyperparameter space.
Both methods can be computationally expensive, especially for large parameter spaces, but they can help automate the process of finding the best set of hyperparameters for a given problem. Additionally, other approaches such as Bayesian optimization can be used to guide the search, but they require additional expertise and computational resources.
Example:
from sklearn.model_selection import GridSearchCV
from sklearn import linear_model
# Define the parameter grid
param_grid = {
'fit_intercept': [True, False],
'normalize': [True, False]
}
# Create a linear regression model
model = linear_model.LinearRegression()
# Create a GridSearchCV object
grid_search = GridSearchCV(model, param_grid, cv=5)
# Perform grid search
grid_search.fit(X, y)
# Print the best parameters
print(grid_search.best_params_)
This concludes our introduction to Scikit-learn. While this section only scratches the surface of what Scikit-learn can do, it should give you a good foundation to build upon.
If you want gain more deep understanding of Scikit-learn we recommend our following book:
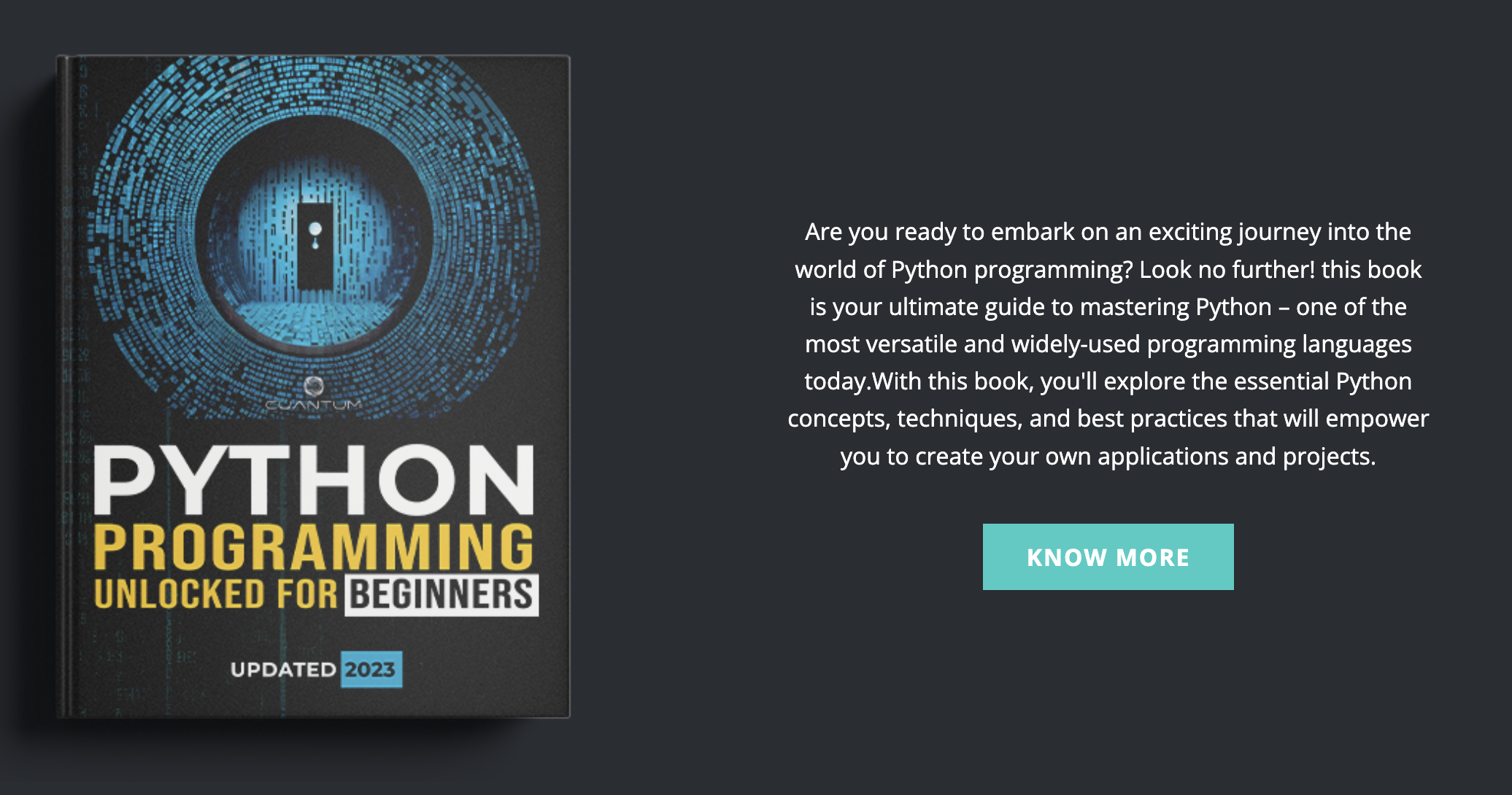
Chapter 2 Conclusion
What an exciting journey we've had in this chapter! We've embarked on an exploration of the Python libraries that are the lifeblood of most machine learning projects. Our adventure began with a brisk walk through Python, where we brushed up on its syntax, data types, control structures, and functions. This was a welcome refresher for our experienced readers and a friendly introduction for those just starting out.
Our next stop was the land of NumPy, a library that gifts us with the power of large multi-dimensional arrays and matrices, along with a treasure trove of mathematical functions to operate on these arrays. We discovered the art of creating arrays, the science of indexing, and the magic of performing mathematical operations on arrays.
Our journey then led us to the realm of Pandas, a library that presents us with robust, expressive, and flexible data structures that make data manipulation and analysis a breeze. We delved into the creation of DataFrames, the selection of data, the cleansing of data, and the performance of basic data analysis.
We also ventured into the territories of Matplotlib and Seaborn, two of the main libraries used for painting the canvas of data visualization in Python. We learned to craft various types of plots, such as line plots, scatter plots, and histograms, akin to artists creating masterpieces. We also dabbled in creating subplots and customizing plot styles, adding our unique touch to our creations.
Finally, we arrived at the gates of Scikit-learn, one of the most popular libraries for machine learning in Python. We covered the essentials of crafting a model, training the model, making predictions, and evaluating the model. We also discussed advanced techniques like cross-validation and hyperparameter tuning, akin to master artisans honing their craft.
By the end of this chapter, you should feel a sense of accomplishment. You've gained a solid understanding of these Python libraries and their role in the grand scheme of machine learning. These libraries form the bedrock upon which we will construct our knowledge in the following chapters. In the next chapter, we will dive into the process of data preprocessing, a crucial step in any machine learning project. So, let's keep the momentum going and continue our adventure!
2.5 Scikit-learn for Machine Learning
Scikit-learn is an open-source library for machine learning in Python that is extensively used in the field. It provides a plethora of supervised and unsupervised learning algorithms to help users build predictive models. The library is designed to be user-friendly and consistent, with a simple interface in Python. It is built on top of NumPy, SciPy, and Matplotlib, which are other popular Python libraries.
In this section, we will cover the basics of Scikit-learn, including data preprocessing, creating a model, training the model, making predictions, and evaluating the model. Preprocessing involves cleaning and transforming the data to make it suitable for machine learning algorithms.
Creating a model involves selecting an appropriate algorithm and setting its parameters. Training the model involves feeding the data to the algorithm and adjusting its parameters to achieve the desired outcome.
Making predictions involves using the trained model to predict the outcome of new data. Evaluating the model involves measuring its performance on a test dataset and fine-tuning it further if necessary. By following these steps, users can gain a better understanding of Scikit-learn and its applications in the field of machine learning.
2.5.1 Installation
Before we start, make sure you have Scikit-learn installed. If you haven't installed it yet, you can do so using pip:
pip install scikit-learn
2.5.2 Importing Scikit-learn
To use Scikit-learn in your Python program, you first need to import it:
from sklearn import preprocessing, model_selection, linear_model, metrics
2.5.3 Data Preprocessing
Scikit-learn provides a variety of utilities for data preprocessing, which can help refine and optimize the data before analysis. One particularly useful tool is the StandardScaler
. This scaler works by standardizing the features of the data, which involves removing the mean and scaling the data to unit variance.
By doing this, the data is transformed to a normal distribution, which can be more easily analyzed using various machine learning algorithms. In addition to the StandardScaler
, Scikit-learn also provides other data preprocessing tools, such as the MinMaxScaler
and the RobustScaler
. These scalers are useful for different situations, such as scaling data to a specific range or handling outliers.
By utilizing these preprocessing tools, you can ensure that your data is optimized and ready for analysis, leading to more accurate and informative results.
Example:
from sklearn import preprocessing
import numpy as np
# Create a StandardScaler
scaler = preprocessing.StandardScaler()
# Fit the StandardScaler to the data
data = [[0, 0], [0, 0], [1, 1], [1, 1]]
scaler.fit(data)
# Transform the data
scaled_data = scaler.transform(data)
print(scaled_data)
2.5.4 Creating a Model
Scikit-learn is a useful library for machine learning enthusiasts. It provides a wide range of machine learning models that can be utilized for different tasks. These models can be easily imported and used in your projects.
There are many models to choose from, such as linear regression, decision trees, random forests, and more. In fact, linear regression models can be created quite easily with Scikit-learn, and can be customized to better suit your specific needs and requirements. So, whether you're a beginner or an experienced machine learning practitioner, Scikit-learn is definitely worth checking out.
Example:
Scikit-learn provides a variety of machine learning models that you can use. For example, you can create a linear regression model like this:
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
2.5.5 Training the Model
Once you have a model, you can train it on your data using the fit
method. Training the model involves passing it your training data multiple times in order to improve its accuracy. During each pass, the model makes predictions on the training data and compares them to the actual values.
It then uses this comparison to adjust the parameters of the model in order to better fit the data. The fit
method also allows you to specify a validation set, which the model can use to evaluate its performance on data that it has not seen during training.
By iterating over the training data multiple times and making adjustments along the way, the model can learn to make increasingly accurate predictions on new data.
Example:
You can train the model on your data using the fit
method:
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
# Train the model
X = [[0, 0], [1, 1]]
y = [0, 1]
model.fit(X, y)
2.5.6 Making Predictions
Once the model is trained, you can use it to make predictions on new data. This can be an incredibly valuable tool in a variety of fields, from finance to healthcare to marketing. Once you have the model up and running, you can use it to generate insights that can help you make better decisions and achieve better outcomes.
The more data you feed into the model, the more accurate it will become, as it is able to learn from experience and adjust its predictions accordingly. So don't be afraid to experiment and try new things--the possibilities are endless with a well-trained predictive model.
Example:
After the model is trained, you can use it to make predictions on new data:
# Make predictions
X_new = [[2, 2]]
y_new = model.predict(X_new)
print(y_new)
2.5.7 Evaluating the Model
Scikit-learn is a powerful Python library that offers a wide array of functions to evaluate the performance of your machine learning models. In addition to the mean squared error, which is commonly used to assess the accuracy of a model, Scikit-learn provides other evaluation metrics such as R-squared, precision, recall, and F1 score.
Understanding these metrics and how to use them properly is crucial for building effective machine learning models that can handle real-world problems. Furthermore, Scikit-learn also provides tools for data preprocessing, feature selection, model selection, and model optimization, which are essential steps in the machine learning pipeline.
With Scikit-learn, you can streamline your machine learning workflow and make the most of your data.
Example:
Scikit-learn provides several functions to evaluate the performance of a model, such as the mean squared error:
from sklearn import metrics
# Calculate the mean squared error of the predictions
y_true = [1]
y_pred = model.predict(X_new)
mse = metrics.mean_squared_error(y_true, y_pred)
print(mse)
2.5.8 Advanced Scikit-learn Features
Cross-Validation
Cross-validation is a powerful statistical method that is used to estimate the skill of machine learning models. It is a commonly used technique in the field of applied machine learning, and is particularly useful when comparing and selecting models for a given predictive modeling problem. One of the main advantages of cross-validation is that it is easy to understand and implement, even for those who are not experts in the field of machine learning.
The results obtained from cross-validation tend to have lower bias than other methods, making it a highly reliable technique for evaluating the performance of machine learning models. Overall, cross-validation is an indispensable tool for anyone working in the field of machine learning, and is sure to become even more important as the field continues to evolve and expand in the coming years.
Example:
from sklearn.model_selection import cross_val_score
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
# Perform 5-fold cross-validation
scores = cross_val_score(model, X, y, cv=5)
# Print cross-validation scores
print(scores)
Hyperparameter Tuning
Machine learning models are parameterized so that their behavior can be tuned for a given problem. These parameters can be modified to achieve better accuracy or to optimize other metrics that are important for a given application.
While some parameters have clear and intuitive interpretations, others can be more subtle and require a deeper understanding of the underlying model. This means that finding the best combination of parameters can be treated as a search problem that requires careful consideration of the trade-offs between different choices.
Scikit-learn provides two methods for automatic hyperparameter tuning: Grid Search and Randomized Search. Grid Search exhaustively searches the hyperparameter space for a given set of parameters, while Randomized Search samples randomly from the hyperparameter space.
Both methods can be computationally expensive, especially for large parameter spaces, but they can help automate the process of finding the best set of hyperparameters for a given problem. Additionally, other approaches such as Bayesian optimization can be used to guide the search, but they require additional expertise and computational resources.
Example:
from sklearn.model_selection import GridSearchCV
from sklearn import linear_model
# Define the parameter grid
param_grid = {
'fit_intercept': [True, False],
'normalize': [True, False]
}
# Create a linear regression model
model = linear_model.LinearRegression()
# Create a GridSearchCV object
grid_search = GridSearchCV(model, param_grid, cv=5)
# Perform grid search
grid_search.fit(X, y)
# Print the best parameters
print(grid_search.best_params_)
This concludes our introduction to Scikit-learn. While this section only scratches the surface of what Scikit-learn can do, it should give you a good foundation to build upon.
If you want gain more deep understanding of Scikit-learn we recommend our following book:
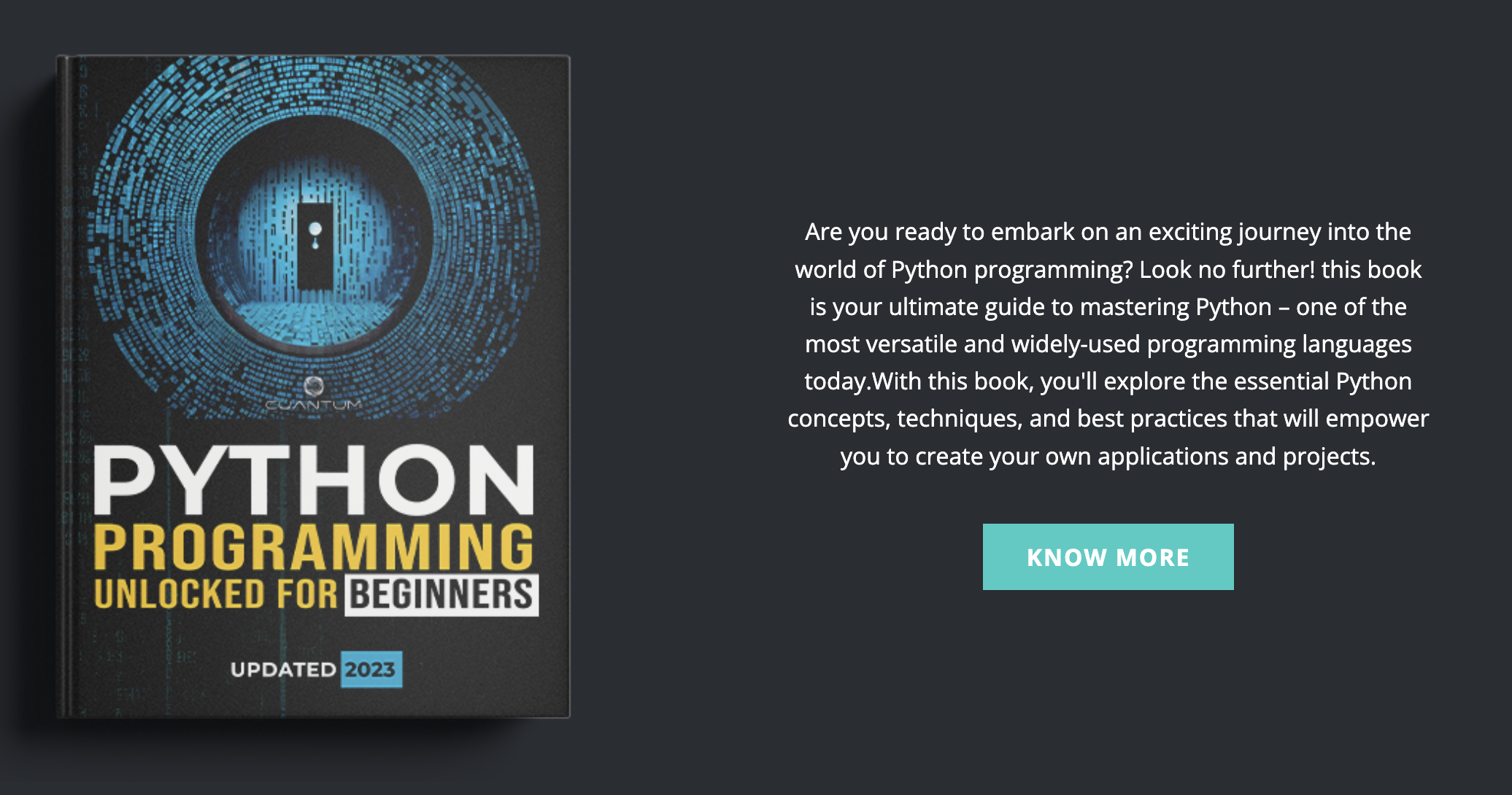
Chapter 2 Conclusion
What an exciting journey we've had in this chapter! We've embarked on an exploration of the Python libraries that are the lifeblood of most machine learning projects. Our adventure began with a brisk walk through Python, where we brushed up on its syntax, data types, control structures, and functions. This was a welcome refresher for our experienced readers and a friendly introduction for those just starting out.
Our next stop was the land of NumPy, a library that gifts us with the power of large multi-dimensional arrays and matrices, along with a treasure trove of mathematical functions to operate on these arrays. We discovered the art of creating arrays, the science of indexing, and the magic of performing mathematical operations on arrays.
Our journey then led us to the realm of Pandas, a library that presents us with robust, expressive, and flexible data structures that make data manipulation and analysis a breeze. We delved into the creation of DataFrames, the selection of data, the cleansing of data, and the performance of basic data analysis.
We also ventured into the territories of Matplotlib and Seaborn, two of the main libraries used for painting the canvas of data visualization in Python. We learned to craft various types of plots, such as line plots, scatter plots, and histograms, akin to artists creating masterpieces. We also dabbled in creating subplots and customizing plot styles, adding our unique touch to our creations.
Finally, we arrived at the gates of Scikit-learn, one of the most popular libraries for machine learning in Python. We covered the essentials of crafting a model, training the model, making predictions, and evaluating the model. We also discussed advanced techniques like cross-validation and hyperparameter tuning, akin to master artisans honing their craft.
By the end of this chapter, you should feel a sense of accomplishment. You've gained a solid understanding of these Python libraries and their role in the grand scheme of machine learning. These libraries form the bedrock upon which we will construct our knowledge in the following chapters. In the next chapter, we will dive into the process of data preprocessing, a crucial step in any machine learning project. So, let's keep the momentum going and continue our adventure!
2.5 Scikit-learn for Machine Learning
Scikit-learn is an open-source library for machine learning in Python that is extensively used in the field. It provides a plethora of supervised and unsupervised learning algorithms to help users build predictive models. The library is designed to be user-friendly and consistent, with a simple interface in Python. It is built on top of NumPy, SciPy, and Matplotlib, which are other popular Python libraries.
In this section, we will cover the basics of Scikit-learn, including data preprocessing, creating a model, training the model, making predictions, and evaluating the model. Preprocessing involves cleaning and transforming the data to make it suitable for machine learning algorithms.
Creating a model involves selecting an appropriate algorithm and setting its parameters. Training the model involves feeding the data to the algorithm and adjusting its parameters to achieve the desired outcome.
Making predictions involves using the trained model to predict the outcome of new data. Evaluating the model involves measuring its performance on a test dataset and fine-tuning it further if necessary. By following these steps, users can gain a better understanding of Scikit-learn and its applications in the field of machine learning.
2.5.1 Installation
Before we start, make sure you have Scikit-learn installed. If you haven't installed it yet, you can do so using pip:
pip install scikit-learn
2.5.2 Importing Scikit-learn
To use Scikit-learn in your Python program, you first need to import it:
from sklearn import preprocessing, model_selection, linear_model, metrics
2.5.3 Data Preprocessing
Scikit-learn provides a variety of utilities for data preprocessing, which can help refine and optimize the data before analysis. One particularly useful tool is the StandardScaler
. This scaler works by standardizing the features of the data, which involves removing the mean and scaling the data to unit variance.
By doing this, the data is transformed to a normal distribution, which can be more easily analyzed using various machine learning algorithms. In addition to the StandardScaler
, Scikit-learn also provides other data preprocessing tools, such as the MinMaxScaler
and the RobustScaler
. These scalers are useful for different situations, such as scaling data to a specific range or handling outliers.
By utilizing these preprocessing tools, you can ensure that your data is optimized and ready for analysis, leading to more accurate and informative results.
Example:
from sklearn import preprocessing
import numpy as np
# Create a StandardScaler
scaler = preprocessing.StandardScaler()
# Fit the StandardScaler to the data
data = [[0, 0], [0, 0], [1, 1], [1, 1]]
scaler.fit(data)
# Transform the data
scaled_data = scaler.transform(data)
print(scaled_data)
2.5.4 Creating a Model
Scikit-learn is a useful library for machine learning enthusiasts. It provides a wide range of machine learning models that can be utilized for different tasks. These models can be easily imported and used in your projects.
There are many models to choose from, such as linear regression, decision trees, random forests, and more. In fact, linear regression models can be created quite easily with Scikit-learn, and can be customized to better suit your specific needs and requirements. So, whether you're a beginner or an experienced machine learning practitioner, Scikit-learn is definitely worth checking out.
Example:
Scikit-learn provides a variety of machine learning models that you can use. For example, you can create a linear regression model like this:
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
2.5.5 Training the Model
Once you have a model, you can train it on your data using the fit
method. Training the model involves passing it your training data multiple times in order to improve its accuracy. During each pass, the model makes predictions on the training data and compares them to the actual values.
It then uses this comparison to adjust the parameters of the model in order to better fit the data. The fit
method also allows you to specify a validation set, which the model can use to evaluate its performance on data that it has not seen during training.
By iterating over the training data multiple times and making adjustments along the way, the model can learn to make increasingly accurate predictions on new data.
Example:
You can train the model on your data using the fit
method:
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
# Train the model
X = [[0, 0], [1, 1]]
y = [0, 1]
model.fit(X, y)
2.5.6 Making Predictions
Once the model is trained, you can use it to make predictions on new data. This can be an incredibly valuable tool in a variety of fields, from finance to healthcare to marketing. Once you have the model up and running, you can use it to generate insights that can help you make better decisions and achieve better outcomes.
The more data you feed into the model, the more accurate it will become, as it is able to learn from experience and adjust its predictions accordingly. So don't be afraid to experiment and try new things--the possibilities are endless with a well-trained predictive model.
Example:
After the model is trained, you can use it to make predictions on new data:
# Make predictions
X_new = [[2, 2]]
y_new = model.predict(X_new)
print(y_new)
2.5.7 Evaluating the Model
Scikit-learn is a powerful Python library that offers a wide array of functions to evaluate the performance of your machine learning models. In addition to the mean squared error, which is commonly used to assess the accuracy of a model, Scikit-learn provides other evaluation metrics such as R-squared, precision, recall, and F1 score.
Understanding these metrics and how to use them properly is crucial for building effective machine learning models that can handle real-world problems. Furthermore, Scikit-learn also provides tools for data preprocessing, feature selection, model selection, and model optimization, which are essential steps in the machine learning pipeline.
With Scikit-learn, you can streamline your machine learning workflow and make the most of your data.
Example:
Scikit-learn provides several functions to evaluate the performance of a model, such as the mean squared error:
from sklearn import metrics
# Calculate the mean squared error of the predictions
y_true = [1]
y_pred = model.predict(X_new)
mse = metrics.mean_squared_error(y_true, y_pred)
print(mse)
2.5.8 Advanced Scikit-learn Features
Cross-Validation
Cross-validation is a powerful statistical method that is used to estimate the skill of machine learning models. It is a commonly used technique in the field of applied machine learning, and is particularly useful when comparing and selecting models for a given predictive modeling problem. One of the main advantages of cross-validation is that it is easy to understand and implement, even for those who are not experts in the field of machine learning.
The results obtained from cross-validation tend to have lower bias than other methods, making it a highly reliable technique for evaluating the performance of machine learning models. Overall, cross-validation is an indispensable tool for anyone working in the field of machine learning, and is sure to become even more important as the field continues to evolve and expand in the coming years.
Example:
from sklearn.model_selection import cross_val_score
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
# Perform 5-fold cross-validation
scores = cross_val_score(model, X, y, cv=5)
# Print cross-validation scores
print(scores)
Hyperparameter Tuning
Machine learning models are parameterized so that their behavior can be tuned for a given problem. These parameters can be modified to achieve better accuracy or to optimize other metrics that are important for a given application.
While some parameters have clear and intuitive interpretations, others can be more subtle and require a deeper understanding of the underlying model. This means that finding the best combination of parameters can be treated as a search problem that requires careful consideration of the trade-offs between different choices.
Scikit-learn provides two methods for automatic hyperparameter tuning: Grid Search and Randomized Search. Grid Search exhaustively searches the hyperparameter space for a given set of parameters, while Randomized Search samples randomly from the hyperparameter space.
Both methods can be computationally expensive, especially for large parameter spaces, but they can help automate the process of finding the best set of hyperparameters for a given problem. Additionally, other approaches such as Bayesian optimization can be used to guide the search, but they require additional expertise and computational resources.
Example:
from sklearn.model_selection import GridSearchCV
from sklearn import linear_model
# Define the parameter grid
param_grid = {
'fit_intercept': [True, False],
'normalize': [True, False]
}
# Create a linear regression model
model = linear_model.LinearRegression()
# Create a GridSearchCV object
grid_search = GridSearchCV(model, param_grid, cv=5)
# Perform grid search
grid_search.fit(X, y)
# Print the best parameters
print(grid_search.best_params_)
This concludes our introduction to Scikit-learn. While this section only scratches the surface of what Scikit-learn can do, it should give you a good foundation to build upon.
If you want gain more deep understanding of Scikit-learn we recommend our following book:
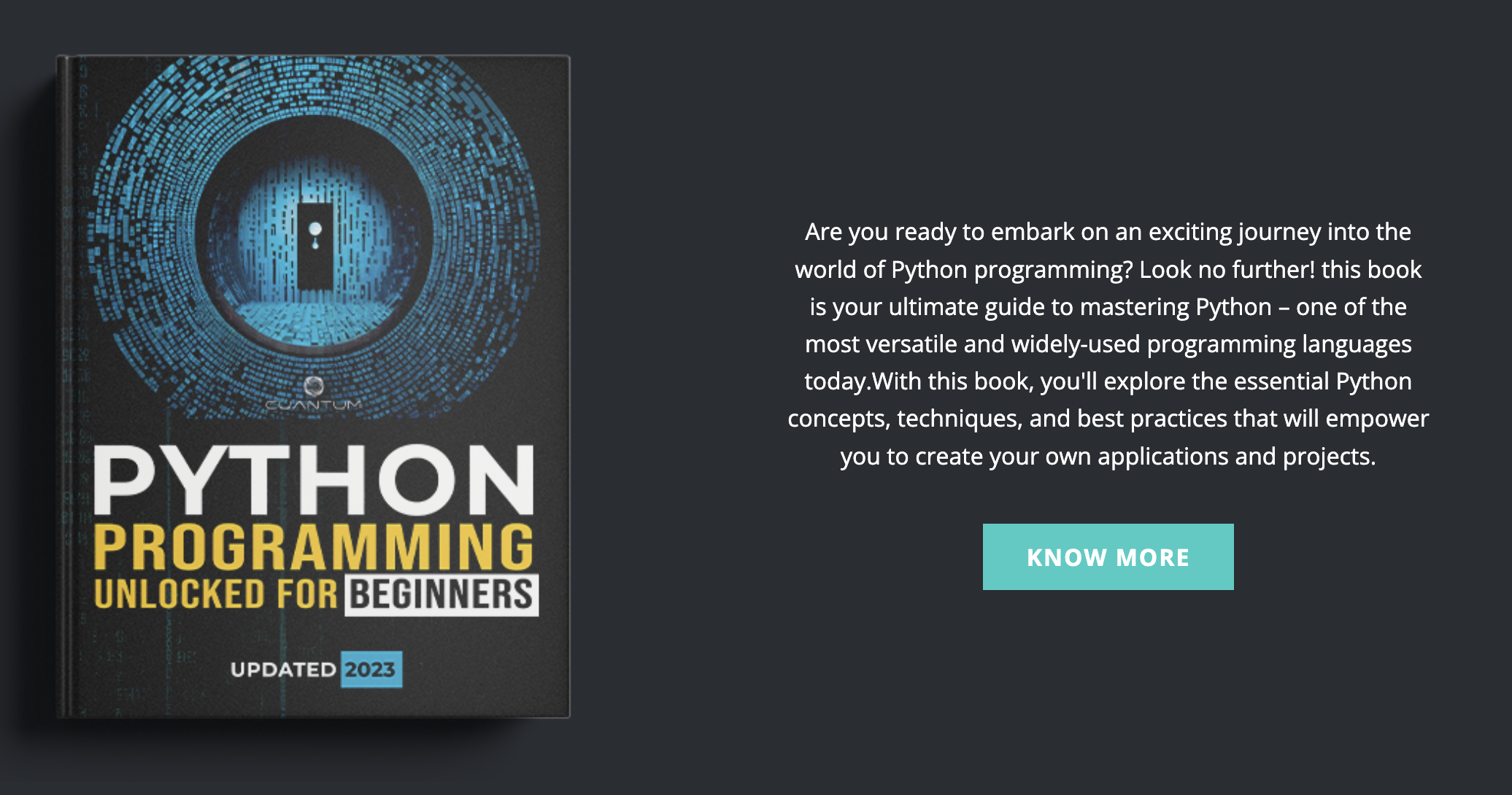
Chapter 2 Conclusion
What an exciting journey we've had in this chapter! We've embarked on an exploration of the Python libraries that are the lifeblood of most machine learning projects. Our adventure began with a brisk walk through Python, where we brushed up on its syntax, data types, control structures, and functions. This was a welcome refresher for our experienced readers and a friendly introduction for those just starting out.
Our next stop was the land of NumPy, a library that gifts us with the power of large multi-dimensional arrays and matrices, along with a treasure trove of mathematical functions to operate on these arrays. We discovered the art of creating arrays, the science of indexing, and the magic of performing mathematical operations on arrays.
Our journey then led us to the realm of Pandas, a library that presents us with robust, expressive, and flexible data structures that make data manipulation and analysis a breeze. We delved into the creation of DataFrames, the selection of data, the cleansing of data, and the performance of basic data analysis.
We also ventured into the territories of Matplotlib and Seaborn, two of the main libraries used for painting the canvas of data visualization in Python. We learned to craft various types of plots, such as line plots, scatter plots, and histograms, akin to artists creating masterpieces. We also dabbled in creating subplots and customizing plot styles, adding our unique touch to our creations.
Finally, we arrived at the gates of Scikit-learn, one of the most popular libraries for machine learning in Python. We covered the essentials of crafting a model, training the model, making predictions, and evaluating the model. We also discussed advanced techniques like cross-validation and hyperparameter tuning, akin to master artisans honing their craft.
By the end of this chapter, you should feel a sense of accomplishment. You've gained a solid understanding of these Python libraries and their role in the grand scheme of machine learning. These libraries form the bedrock upon which we will construct our knowledge in the following chapters. In the next chapter, we will dive into the process of data preprocessing, a crucial step in any machine learning project. So, let's keep the momentum going and continue our adventure!
2.5 Scikit-learn for Machine Learning
Scikit-learn is an open-source library for machine learning in Python that is extensively used in the field. It provides a plethora of supervised and unsupervised learning algorithms to help users build predictive models. The library is designed to be user-friendly and consistent, with a simple interface in Python. It is built on top of NumPy, SciPy, and Matplotlib, which are other popular Python libraries.
In this section, we will cover the basics of Scikit-learn, including data preprocessing, creating a model, training the model, making predictions, and evaluating the model. Preprocessing involves cleaning and transforming the data to make it suitable for machine learning algorithms.
Creating a model involves selecting an appropriate algorithm and setting its parameters. Training the model involves feeding the data to the algorithm and adjusting its parameters to achieve the desired outcome.
Making predictions involves using the trained model to predict the outcome of new data. Evaluating the model involves measuring its performance on a test dataset and fine-tuning it further if necessary. By following these steps, users can gain a better understanding of Scikit-learn and its applications in the field of machine learning.
2.5.1 Installation
Before we start, make sure you have Scikit-learn installed. If you haven't installed it yet, you can do so using pip:
pip install scikit-learn
2.5.2 Importing Scikit-learn
To use Scikit-learn in your Python program, you first need to import it:
from sklearn import preprocessing, model_selection, linear_model, metrics
2.5.3 Data Preprocessing
Scikit-learn provides a variety of utilities for data preprocessing, which can help refine and optimize the data before analysis. One particularly useful tool is the StandardScaler
. This scaler works by standardizing the features of the data, which involves removing the mean and scaling the data to unit variance.
By doing this, the data is transformed to a normal distribution, which can be more easily analyzed using various machine learning algorithms. In addition to the StandardScaler
, Scikit-learn also provides other data preprocessing tools, such as the MinMaxScaler
and the RobustScaler
. These scalers are useful for different situations, such as scaling data to a specific range or handling outliers.
By utilizing these preprocessing tools, you can ensure that your data is optimized and ready for analysis, leading to more accurate and informative results.
Example:
from sklearn import preprocessing
import numpy as np
# Create a StandardScaler
scaler = preprocessing.StandardScaler()
# Fit the StandardScaler to the data
data = [[0, 0], [0, 0], [1, 1], [1, 1]]
scaler.fit(data)
# Transform the data
scaled_data = scaler.transform(data)
print(scaled_data)
2.5.4 Creating a Model
Scikit-learn is a useful library for machine learning enthusiasts. It provides a wide range of machine learning models that can be utilized for different tasks. These models can be easily imported and used in your projects.
There are many models to choose from, such as linear regression, decision trees, random forests, and more. In fact, linear regression models can be created quite easily with Scikit-learn, and can be customized to better suit your specific needs and requirements. So, whether you're a beginner or an experienced machine learning practitioner, Scikit-learn is definitely worth checking out.
Example:
Scikit-learn provides a variety of machine learning models that you can use. For example, you can create a linear regression model like this:
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
2.5.5 Training the Model
Once you have a model, you can train it on your data using the fit
method. Training the model involves passing it your training data multiple times in order to improve its accuracy. During each pass, the model makes predictions on the training data and compares them to the actual values.
It then uses this comparison to adjust the parameters of the model in order to better fit the data. The fit
method also allows you to specify a validation set, which the model can use to evaluate its performance on data that it has not seen during training.
By iterating over the training data multiple times and making adjustments along the way, the model can learn to make increasingly accurate predictions on new data.
Example:
You can train the model on your data using the fit
method:
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
# Train the model
X = [[0, 0], [1, 1]]
y = [0, 1]
model.fit(X, y)
2.5.6 Making Predictions
Once the model is trained, you can use it to make predictions on new data. This can be an incredibly valuable tool in a variety of fields, from finance to healthcare to marketing. Once you have the model up and running, you can use it to generate insights that can help you make better decisions and achieve better outcomes.
The more data you feed into the model, the more accurate it will become, as it is able to learn from experience and adjust its predictions accordingly. So don't be afraid to experiment and try new things--the possibilities are endless with a well-trained predictive model.
Example:
After the model is trained, you can use it to make predictions on new data:
# Make predictions
X_new = [[2, 2]]
y_new = model.predict(X_new)
print(y_new)
2.5.7 Evaluating the Model
Scikit-learn is a powerful Python library that offers a wide array of functions to evaluate the performance of your machine learning models. In addition to the mean squared error, which is commonly used to assess the accuracy of a model, Scikit-learn provides other evaluation metrics such as R-squared, precision, recall, and F1 score.
Understanding these metrics and how to use them properly is crucial for building effective machine learning models that can handle real-world problems. Furthermore, Scikit-learn also provides tools for data preprocessing, feature selection, model selection, and model optimization, which are essential steps in the machine learning pipeline.
With Scikit-learn, you can streamline your machine learning workflow and make the most of your data.
Example:
Scikit-learn provides several functions to evaluate the performance of a model, such as the mean squared error:
from sklearn import metrics
# Calculate the mean squared error of the predictions
y_true = [1]
y_pred = model.predict(X_new)
mse = metrics.mean_squared_error(y_true, y_pred)
print(mse)
2.5.8 Advanced Scikit-learn Features
Cross-Validation
Cross-validation is a powerful statistical method that is used to estimate the skill of machine learning models. It is a commonly used technique in the field of applied machine learning, and is particularly useful when comparing and selecting models for a given predictive modeling problem. One of the main advantages of cross-validation is that it is easy to understand and implement, even for those who are not experts in the field of machine learning.
The results obtained from cross-validation tend to have lower bias than other methods, making it a highly reliable technique for evaluating the performance of machine learning models. Overall, cross-validation is an indispensable tool for anyone working in the field of machine learning, and is sure to become even more important as the field continues to evolve and expand in the coming years.
Example:
from sklearn.model_selection import cross_val_score
from sklearn import linear_model
# Create a linear regression model
model = linear_model.LinearRegression()
# Perform 5-fold cross-validation
scores = cross_val_score(model, X, y, cv=5)
# Print cross-validation scores
print(scores)
Hyperparameter Tuning
Machine learning models are parameterized so that their behavior can be tuned for a given problem. These parameters can be modified to achieve better accuracy or to optimize other metrics that are important for a given application.
While some parameters have clear and intuitive interpretations, others can be more subtle and require a deeper understanding of the underlying model. This means that finding the best combination of parameters can be treated as a search problem that requires careful consideration of the trade-offs between different choices.
Scikit-learn provides two methods for automatic hyperparameter tuning: Grid Search and Randomized Search. Grid Search exhaustively searches the hyperparameter space for a given set of parameters, while Randomized Search samples randomly from the hyperparameter space.
Both methods can be computationally expensive, especially for large parameter spaces, but they can help automate the process of finding the best set of hyperparameters for a given problem. Additionally, other approaches such as Bayesian optimization can be used to guide the search, but they require additional expertise and computational resources.
Example:
from sklearn.model_selection import GridSearchCV
from sklearn import linear_model
# Define the parameter grid
param_grid = {
'fit_intercept': [True, False],
'normalize': [True, False]
}
# Create a linear regression model
model = linear_model.LinearRegression()
# Create a GridSearchCV object
grid_search = GridSearchCV(model, param_grid, cv=5)
# Perform grid search
grid_search.fit(X, y)
# Print the best parameters
print(grid_search.best_params_)
This concludes our introduction to Scikit-learn. While this section only scratches the surface of what Scikit-learn can do, it should give you a good foundation to build upon.
If you want gain more deep understanding of Scikit-learn we recommend our following book:
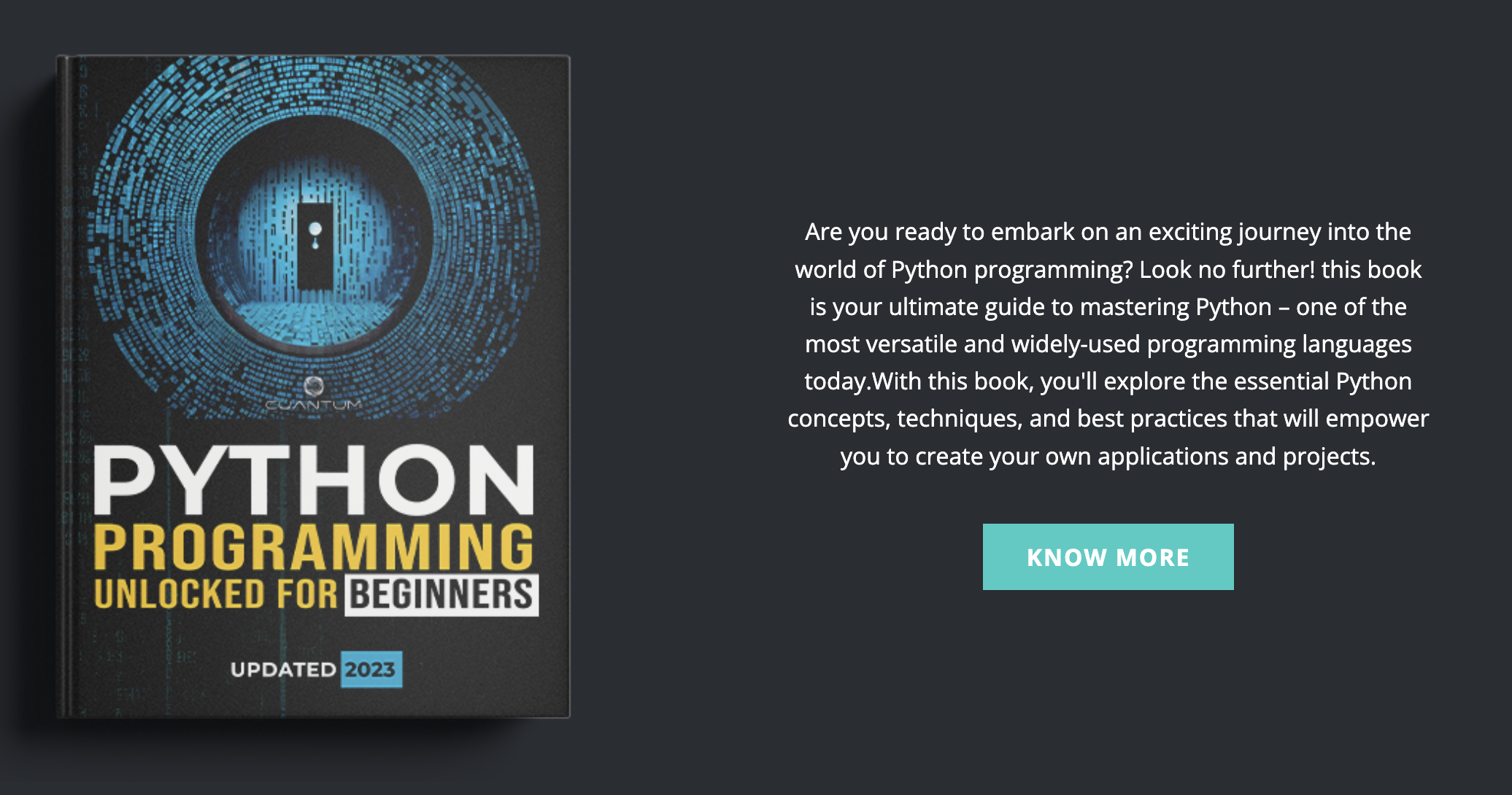
Chapter 2 Conclusion
What an exciting journey we've had in this chapter! We've embarked on an exploration of the Python libraries that are the lifeblood of most machine learning projects. Our adventure began with a brisk walk through Python, where we brushed up on its syntax, data types, control structures, and functions. This was a welcome refresher for our experienced readers and a friendly introduction for those just starting out.
Our next stop was the land of NumPy, a library that gifts us with the power of large multi-dimensional arrays and matrices, along with a treasure trove of mathematical functions to operate on these arrays. We discovered the art of creating arrays, the science of indexing, and the magic of performing mathematical operations on arrays.
Our journey then led us to the realm of Pandas, a library that presents us with robust, expressive, and flexible data structures that make data manipulation and analysis a breeze. We delved into the creation of DataFrames, the selection of data, the cleansing of data, and the performance of basic data analysis.
We also ventured into the territories of Matplotlib and Seaborn, two of the main libraries used for painting the canvas of data visualization in Python. We learned to craft various types of plots, such as line plots, scatter plots, and histograms, akin to artists creating masterpieces. We also dabbled in creating subplots and customizing plot styles, adding our unique touch to our creations.
Finally, we arrived at the gates of Scikit-learn, one of the most popular libraries for machine learning in Python. We covered the essentials of crafting a model, training the model, making predictions, and evaluating the model. We also discussed advanced techniques like cross-validation and hyperparameter tuning, akin to master artisans honing their craft.
By the end of this chapter, you should feel a sense of accomplishment. You've gained a solid understanding of these Python libraries and their role in the grand scheme of machine learning. These libraries form the bedrock upon which we will construct our knowledge in the following chapters. In the next chapter, we will dive into the process of data preprocessing, a crucial step in any machine learning project. So, let's keep the momentum going and continue our adventure!