Chapter 2: Python and Essential Libraries
2.1 Python Crash Course
Welcome to Chapter 2! Here, we will take a deeper dive into the world of Python and its essential libraries that are widely used in the field of Machine Learning. As you may already know, Python has become the go-to language for many data scientists and machine learning engineers due to its simplicity and extensive libraries. Python's popularity is largely due to the fact that it is an open-source programming language that is easy to learn and use.
Throughout this chapter, we will cover a range of topics, from the basics of Python programming to the most essential libraries used in Machine Learning. We will start by discussing Python syntax and data types, including variables, loops, conditions, and functions. Next, we will explore some of the most essential libraries used in Machine Learning, such as NumPy, Pandas, and Matplotlib, and how they can be used to process, analyze, and visualize data.
We will also discuss the basics of Machine Learning, including supervised and unsupervised learning, and how to implement them using Python. By the end of this chapter, you will have a solid understanding of Python and its key libraries, setting a strong foundation for the rest of the book.
Let's start with a crash course on Python.
Python is a powerful and versatile high-level programming language that is widely used in various fields, including web development, data analysis, artificial intelligence, and more. Its simplicity and readability make it a great language for beginners, but it can also handle complex tasks and large-scale projects.
In this section, we will delve into the basics of Python, discussing data types, control flow, functions, and classes in detail. We will also explore some of the practical applications of Python in various industries, including finance, healthcare, and education. By the end of this section, you will have a solid understanding of the fundamentals of Python and be able to build simple programs on your own.
It's important to note that this section is not meant to be a comprehensive guide to Python, but rather a quick overview to get you started. If you're already familiar with Python, feel free to skim through this section or move on to the next one, where we will explore more advanced topics in Python programming.
2.1.1 Data Types
Python has several built-in data types, including integers, floats, strings, lists, tuples, sets, and dictionaries. Here's a quick overview:
Integers and Floats
Integers are a type of number that represent whole quantities, while floats, or floating point numbers, are a type of number that represent decimal quantities. Both types of numbers can be used in basic arithmetic operations, such as addition, subtraction, multiplication, and division.
Integers can be used in modulo operations, which find the remainder of a division operation. Floats can also be used in more complex mathematical operations, such as trigonometric functions and logarithms. It is important to note that when performing arithmetic operations with both integers and floats, the result will be a float.
Example:
# Integers
x = 10
y = 2
print(x + y) # Addition
print(x - y) # Subtraction
print(x * y) # Multiplication
print(x / y) # Division
# Floats
a = 1.5
b = 0.5
print(a + b)
print(a - b)
print(a * b)
print(a / b)
Code Purpose:
This code snippet demonstrates performing basic arithmetic operations on integer and floating-point numbers in Python. It showcases the behavior of the addition, subtraction, multiplication, and division operators for both data types.
Step-by-Step Breakdown:
- Integer Arithmetic:
- The code assigns the integer value
10
to the variablex
and2
to the variabley
. - It then performs the following operations using the arithmetic operators:
print(x + y)
: This calculates the sum ofx
andy
(which is 12) and prints the result.print(x - y)
: This calculates the difference ofx
andy
(which is 8) and prints the result.print(x * y)
: This calculates the product ofx
andy
(which is 20) and prints the result.print(x / y)
: This performs integer division ofx
byy
. Since both operands are integers, Python performs integer division by default, resulting in5.0
. Note that the result is a float, even though the operands are integers.
- The code assigns the integer value
- Float Arithmetic:
- The code assigns the floating-point value
1.5
to the variablea
and0.5
to the variableb
. - Similar to integer arithmetic, it performs operations using the same operators and prints the results:
print(a + b)
: This calculates the sum ofa
andb
(which is 2.0) and prints the result.print(a - b)
: This calculates the difference ofa
andb
(which is 1.0) and prints the result.print(a * b)
: This calculates the product ofa
andb
(which is 0.75) and prints the result.print(a / b)
: This performs floating-point division ofa
byb
. Since at least one operand is a float, Python performs division with floating-point precision, resulting in3.0
.
- The code assigns the floating-point value
Key Points:
- Python supports arithmetic operations on both integers (whole numbers) and floats (decimal numbers).
- The behavior of the division operator (
/
) differs for integers and floats.- For integers, it performs integer division, discarding any remainder (resulting in a float if necessary).
- For floats or mixed operand types (one integer and one float), it performs floating-point division, preserving decimal precision.
Strings
Strings are sequences of characters that are used to represent text in programming. They can be created by enclosing characters in single quotes ('') or double quotes (""). Once created, strings can be manipulated in various ways, such as by appending new characters to them or by extracting specific characters from them.
Strings can be formatted to include values that change dynamically, such as dates or user input. This allows for more dynamic and interactive programs that can respond to user input in real time. Overall, strings are a fundamental concept in programming that allow for the representation and manipulation of text-based data.
Example:
s = 'Hello, world!'
print(s)
Lists
Lists are an essential part of programming as they allow ordered collections of items. Lists are mutable, allowing you to make modifications to their content. One example of a list could be a to-do list that you might use to keep track of tasks you need to complete. You could add or remove items from this list as you complete tasks or think of new ones.
In addition to to-do lists, lists can be used for many other purposes, such as storing employee names and phone numbers, a list of your favorite books, or even a list of countries you'd like to visit someday. Lists can also be nested within each other to create more complex structures. For example, a list of to-do lists could be used to categorize your tasks by subject or priority.
Lists are a versatile and integral part of programming that can be used in a variety of ways to help organize and manage data.
Example:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Add an item to the list
fruits.append('date')
# Remove an item from the list
fruits.remove('banana')
# Access an item in the list
print(fruits[0]) # 'apple'
Code Purpose:
This code snippet demonstrates how to create a list in Python and perform common list manipulation operations, including adding, removing, and accessing elements.
Step-by-Step Breakdown:
- Creating a List:
- The line
fruits = ['apple', 'banana', 'cherry']
creates a list namedfruits
. Lists are used to store ordered collections of items in Python. In this case, the listfruits
contains three string elements: "apple", "banana", and "cherry".
- The line
- Adding an Item to the List (Append):
- The line
fruits.append('date')
adds the string element "date" to the end of thefruits
list using the.append
method. Lists are mutable, meaning their contents can be changed after creation. The.append
method is a convenient way to add new items to the end of a list.
- The line
- Removing an Item from the List (Remove):
- The line
fruits.remove('banana')
removes the first occurrence of the string element "banana" from thefruits
list using the.remove
method. It's important to note that the.remove
method removes the element based on its value, not its position in the list. If the element is not found, it will raise aValueError
.
- The line
- Accessing an Item in the List:
- The line
print(fruits[0])
prints the first element of thefruits
list. Lists are indexed starting from 0, sofruits[0]
refers to the element at index 0, which is "apple" in this case.
- The line
Key Points:
- Lists are a fundamental data structure in Python for storing collections of items.
- You can create lists using square brackets
[]
and enclosing elements separated by commas. - The
.append
method provides a way to add items to the end of a list. - The
.remove
method removes the first occurrence of a specified element from the list. - Lists are ordered, and you can access elements by their index within square brackets.
Tuples
Tuples and lists are both data structures in Python. While they share similarities, such as the ability to store multiple values in a single variable, there are also key differences. One of the main differences is that tuples are immutable, meaning that once a tuple is created, you cannot change its content. In contrast, lists are mutable, which means you can add, remove or modify elements after the list is created.
Despite their immutability, tuples are still useful in a number of scenarios. For example, they are commonly used to store related values together, such as the coordinates of a point in space. Tuples can also be used as keys in dictionaries, since they are hashable. Tuples can be used to return multiple values from a function, making them a useful tool for handling complex data.
While tuples may seem limited due to their immutability, they offer a number of advantages and use cases that make them a valuable tool in any Python programmer's toolkit.
Example:
# Create a tuple
coordinates = (10.0, 20.0)
# Access an item in the tuple
print(coordinates[0]) # 10.0
Code Purpose:
This code snippet demonstrates how to create a tuple in Python and access elements by their index.
Step-by-Step Breakdown:
- Creating a Tuple:
- The line
coordinates = (10.0, 20.0)
creates a tuple namedcoordinates
. Tuples are another fundamental data structure in Python used to store ordered collections of elements. However, unlike lists, tuples are immutable, meaning their contents cannot be changed after creation. In this case, the tuplecoordinates
contains two elements:10.0
(a float) and20.0
(a float).
- The line
- Accessing an Item in the Tuple:
- The line
print(coordinates[0])
prints the first element of thecoordinates
tuple. Similar to lists, tuples are indexed starting from 0. So,coordinates[0]
refers to the element at index 0, which is10.0
in this example.
- The line
Key Points:
- Tuples are ordered collections of elements like lists, but they are immutable (unchangeable after creation).
- Tuples are created using parentheses
()
, enclosing elements separated by commas. - You can access elements in tuples by their index within square brackets, similar to lists.
Sets
Sets are unordered collections of unique items. They are useful in various programming scenarios, such as counting unique items in a list or testing for membership. Sets can also be combined using mathematical operations such as union, intersection, and difference. In Python, sets are represented using curly braces and items are separated by commas.
One important characteristic of sets is that they do not allow duplicates. This means that if an item is added to a set that already exists, it will not change the set. Furthermore, sets are mutable, which means that items can be added or removed from the set after it has been created. Overall, sets are a versatile and important tool in programming.
Example:
# Create a set
fruits = {'apple', 'banana', 'cherry', 'apple'}
# Add an item to the set
fruits.add('date')
# Remove an item from the set
fruits.remove('banana')
# Check if an item is in the set
print('apple' in fruits) # True
Code Purpose:
This code snippet demonstrates how to create a set in Python and perform common set operations, including adding, removing, and checking for element membership.
Step-by-Step Breakdown:
- Creating a Set:
- The line
fruits = {'apple', 'banana', 'cherry', 'apple'}
creates a set namedfruits
. Sets are used to store collections of unique elements in Python. Unlike lists, sets do not allow duplicate elements. In this case, the setfruits
is created with four elements: "apple", "banana", "cherry", and "apple". However, since sets don't allow duplicates, the second occurrence of "apple" is ignored.
- The line
- Adding an Item to the Set (Add):
- The line
fruits.add('date')
adds the string element "date" to thefruits
set using the.add
method. Sets are mutable, meaning their contents can be changed after creation. The.add
method is a way to add new, unique elements to a set.
- The line
- Removing an Item from the Set (Remove):
- The line
fruits.remove('banana')
removes the element "banana" from thefruits
set using the.remove
method. Similar to lists, it removes the first occurrence of the specified element. It's important to note that.remove
will raise aKeyError
if the element is not found in the set.
- The line
- Checking for Membership:
- The line
print('apple' in fruits)
checks if the element "apple" exists in thefruits
set using thein
operator. Thein
operator returnsTrue
if the element is found in the set, andFalse
otherwise. In this case, it printsTrue
because "apple" is indeed in the set.
- The line
Key Points:
- Sets are collections of unique elements, ideal for storing unordered collections where duplicates are not allowed.
- You create sets using curly braces
{}
and enclosing elements separated by commas. - The
.add
method adds unique elements to a set. - The
.remove
method removes an element from the set, raising an error if not found. - The
in
operator checks if an element exists within a set.
Dictionaries
Dictionaries are data structures that contain key-value pairs, allowing for efficient lookups and storage of information. These pairs can represent any type of data, such as strings, numbers, or even other dictionaries. In addition to lookup and storage, dictionaries can also be used for data manipulation and analysis.
With the flexibility and versatility of dictionaries, they have become a staple in many programming languages, used in applications ranging from database management to natural language processing.
Example:
# Create a dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Access a value in the dictionary
print(person['name']) # 'Alice'
# Change a value in the dictionary
person['age'] = 26
# Add a new key-value pair to the dictionary
person['job'] = 'Engineer'
# Remove a key-value pair from the dictionary
del person['city']
# Print the modified dictionary
print(person)
Code Purpose:
This code snippet demonstrates how to create a dictionary in Python, access and modify its elements, and perform common operations like adding and removing key-value pairs.
Step-by-Step Breakdown:
- Creating a Dictionary:
- The line
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
creates a dictionary namedperson
. Dictionaries are fundamental data structures in Python for storing collections of data in a key-value format. In this case, theperson
dictionary stores three key-value pairs:- Key: 'name', Value: 'Alice' (associates the name "Alice" with the key 'name')
- Key: 'age', Value: 25 (associates the age 25 with the key 'age')
- Key: 'city', Value: 'New York' (associates the city "New York" with the key 'city')
- The line
- Accessing a Value in the Dictionary:
- The line
print(person['name'])
retrieves the value associated with the key 'name' from theperson
dictionary and prints it. Since 'name' is a key, it retrieves the corresponding value, which is "Alice" in this case.
- The line
- Changing a Value in the Dictionary:
- The line
person['age'] = 26
modifies the value associated with the key 'age' in theperson
dictionary. Dictionaries are mutable, meaning you can change their contents after creation. Here, we update the age to 26.
- The line
- Adding a New Key-Value Pair:
- The line
person['job'] = 'Engineer'
adds a new key-value pair to theperson
dictionary. The key is 'job', and the value is 'Engineer'. This extends the dictionary with new information.
- The line
- Removing a Key-Value Pair:
- The line
del person['city']
removes the key-value pair with the key 'city' from theperson
dictionary. Thedel
keyword is used for deletion.
- The line
- Printing the Modified Dictionary:
- The line
print(person)
prints the contents of the modifiedperson
dictionary. This will display the updated dictionary without the 'city' key-value pair and the changed age.
- The line
Key Points:
- Dictionaries are collections of key-value pairs, providing a flexible way to store and access data.
- You create dictionaries using curly braces
{}
with keys and values separated by colons (:
). Keys must be unique and immutable (e.g., strings, numbers). - Accessing elements is done using the key within square brackets
[]
. - You can modify existing values or add new key-value pairs using assignment.
- The
del
keyword removes key-value pairs from the dictionary.
2.1.2 Control Flow
Control flow is a crucial concept in programming. It refers to the order in which the code of a program is executed. In Python, there are several mechanisms that can be used to control the flow of a program.
One of these is the if
statement, which allows the program to make decisions based on certain conditions. Another important mechanism is the for
loop, which is used to iterate over a sequence of values or elements. Similarly, the while
loop can be used to execute a block of code repeatedly as long as a certain condition is met.
In addition to these mechanisms, Python also provides try
/except
blocks, which are used for error handling. These blocks are particularly useful when a program encounters an error or an exception that it cannot handle, as they allow the program to gracefully recover from the error and continue executing its code.
It is important for programmers to have a solid understanding of control flow and the different mechanisms that can be used to control it in Python. By using these mechanisms effectively, programmers can create programs that are both efficient and robust, and that can handle a wide range of different scenarios and situations.
If Statements
If
statements are used for conditional execution, allowing a program to make decisions based on certain conditions. This is a powerful tool that gives developers more control over the flow of their code by allowing them to specify what should happen under certain circumstances.
For example, an if
statement could be used to check if a user has entered the correct password, and if so, grant them access to a restricted area of a website. Alternatively, it could be used to check if a user has entered an invalid input, and prompt them to try again.
By using if
statements, developers can create more dynamic and versatile programs that can respond to a wider range of user inputs and conditions.
Example:
x = 10
if x > 0:
print('x is positive')
elif x < 0:
print('x is negative')
else:
print('x is zero')
For Loops
For
loops are an essential tool in programming. They are used for iterating over a sequence (like a list or a string), making it possible to perform operations on each element of the sequence. This is incredibly useful in a wide variety of contexts.
For example, imagine you have a list of items and you want to perform the same calculation on each item. A for
loop makes this task simple and efficient. By iterating over the list, the loop performs the calculation on each item in turn, saving you from having to write the same code over and over again.
In addition to lists and strings, for
loops can also be used with other types of sequences, such as dictionaries and sets, making them an incredibly versatile tool for any programmer to have in their toolkit.
Example:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
While Loops
While
loops are used for repeated execution as long as a condition is true. This means that if the condition is false from the outset, the loop will never execute. It is important to ensure that the condition will eventually become false, otherwise the loop will continue indefinitely.
In practice, while
loops can be useful for situations where you do not know how many iterations will be required. For example, if you are writing a program to calculate the square root of a number, you may not know how many iterations will be required until you get the desired level of precision.
Another use of while
loops is to repeatedly ask the user for input until they provide valid input. This can be useful to ensure that the program does not crash or behave unexpectedly due to invalid user input.
Overall, while
loops are a powerful programming construct that can be used to solve a wide variety of problems. By using them effectively, you can write code that is more efficient, easier to read, and easier to maintain.
Example:
x = 0
while x < 5:
print(x)
x += 1
2.1.3 Try/Except Blocks
Try
/except
blocks are a key part of Python programming for error handling. These blocks allow the programmer to anticipate and handle errors that may occur during the execution of a Python script. By using try/except blocks, a programmer can create a more robust and error-resistant program that can handle unexpected input or other errors without crashing. In fact, try/except blocks are so commonly used in Python programming that they are often considered a fundamental aspect of the language's syntax and functionality.
Example:
try:
x = 1 / 0 # This will raise a ZeroDivisionError
except ZeroDivisionError:
print('You cannot divide by zero!')
2.1.4 Functions
Functions are a key concept in programming that allow for the creation of reusable pieces of code. When defining a function in Python, the def
keyword is used. Functions can contain any number of statements, including loops, conditional statements, and other functions.
Additionally, functions can have parameters and return values, which allows for greater flexibility in how they are used. By breaking down a problem into smaller functions, code can be made more readable and easier to maintain.
Functions are an essential tool for any programmer looking to improve the efficiency and effectiveness of their code.
Example:
def greet(name):
return f'Hello, {name}!'
print(greet('Alice')) # 'Hello, Alice!'
2.1.5 Classes
Object-oriented programming is centered around the concept of classes. A class is essentially a blueprint that describes the properties, methods, and behaviors of objects that are created from it.
These objects can be thought of as instances of the class, each with their own unique set of values for the properties defined by the class. When you create an object from a class, you are essentially instantiating it, meaning that you are creating a new instance of the class with its own set of values.
Once an object has been created, you can then call its methods to perform various tasks. By defining these methods within the class, you can ensure that the same functionality is available to all instances of the class.
Classes provide a powerful mechanism for organizing and managing complex code, allowing you to create reusable, modular code that can be shared across multiple projects.
Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f'Hello, my name is {self.name} and I am {self.age} years old.'
alice = Person('Alice', 25)
print(alice.greet()) # 'Hello, my name is Alice and I am 25 years old.'
2.1.6 Built-in Functions and Modules
Python offers an extensive range of built-in functions and modules that provide additional functionality to the language. These modules are designed to be used in a variety of applications, from data analysis to web development and beyond.
For example, the math module provides access to mathematical functions such as trigonometry, while the os module enables interaction with the operating system. Python's standard library includes modules for working with regular expressions, file I/O, and network communication, to name just a few.
With such a wide array of tools at your disposal, Python is a powerful language that can handle a vast range of tasks and applications.
Here are some of the most commonly used ones:
Built-in Functions
Python has many built-in functions that perform a variety of tasks. Here are a few examples:
print()
: Prints the specified message to the screen.len()
: Returns the number of items in an object.type()
: Returns the type of an object.str()
,int()
,float()
: Convert an object to a string, integer, or float, respectively.input()
: Reads a line from input (keyboard), converts it to a string, and returns it.
Here's how you can use these functions:
print(len('Hello, world!')) # 13
print(type(10)) # <class 'int'>
print(int('10')) # 10
name = input('What is your name? ')
Built-in Modules
Python also comes with a set of built-in modules that you can import into your program to use. Here are a few examples:
math
: Provides mathematical functions.random
: Provides functions for generating random numbers.datetime
: Provides functions for manipulating dates and times.os
: Provides functions for interacting with the operating system.
Here's how you can use these modules:
import math
print(math.sqrt(16)) # 4.0
import random
print(random.randint(1, 10)) # a random integer between 1 and 10
import datetime
print(datetime.datetime.now()) # current date and time
import os
print(os.getcwd()) # current working directory
This concludes our Python crash course. While this section only scratches the surface of what Python can do, it should give you a good foundation to build upon. In the next sections, we will explore some of the key Python libraries used in Machine Learning.
If you want to gain a better and deeper understanding of Python basics, this book may be of interest to you:
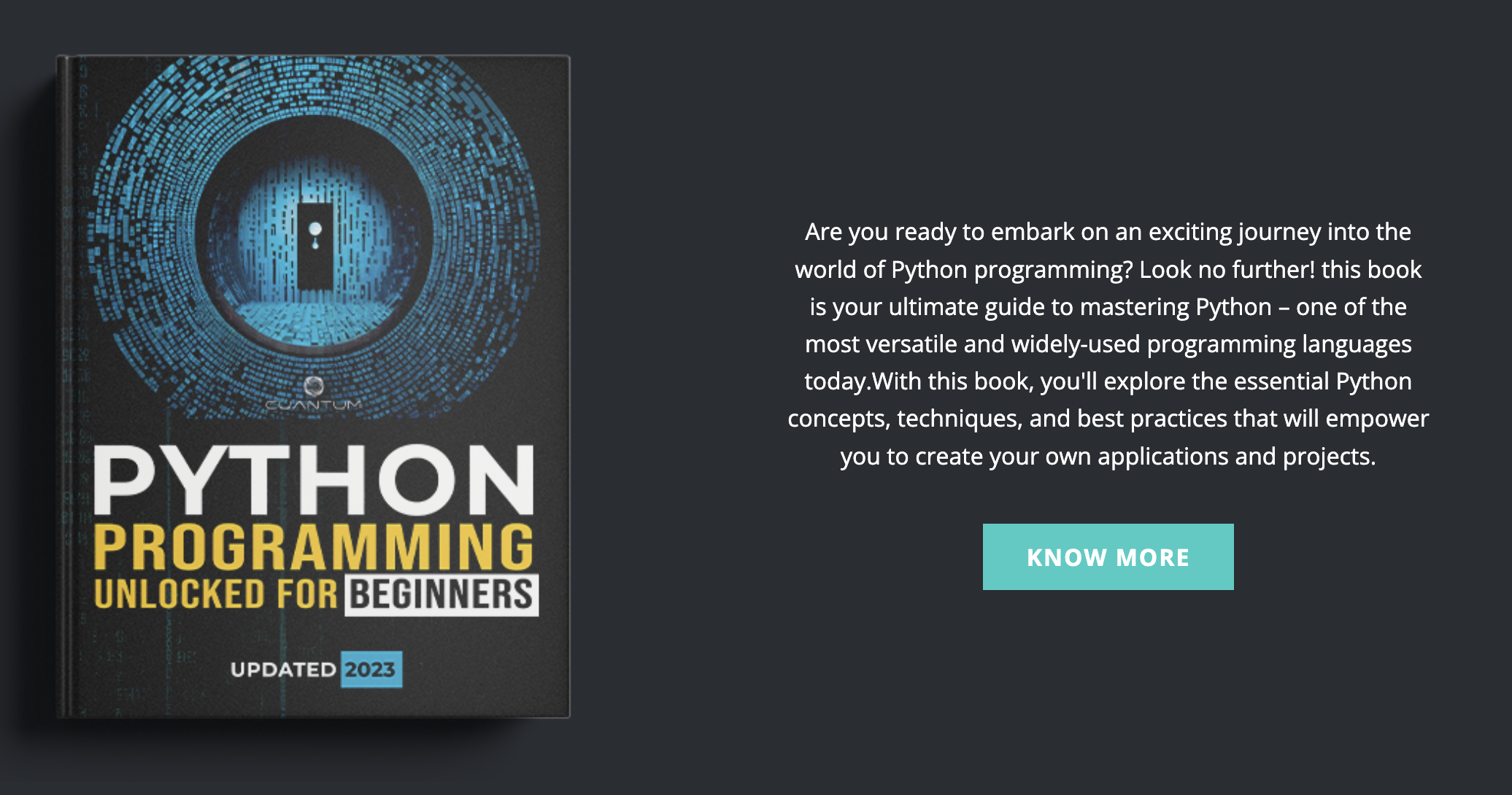
2.1 Python Crash Course
Welcome to Chapter 2! Here, we will take a deeper dive into the world of Python and its essential libraries that are widely used in the field of Machine Learning. As you may already know, Python has become the go-to language for many data scientists and machine learning engineers due to its simplicity and extensive libraries. Python's popularity is largely due to the fact that it is an open-source programming language that is easy to learn and use.
Throughout this chapter, we will cover a range of topics, from the basics of Python programming to the most essential libraries used in Machine Learning. We will start by discussing Python syntax and data types, including variables, loops, conditions, and functions. Next, we will explore some of the most essential libraries used in Machine Learning, such as NumPy, Pandas, and Matplotlib, and how they can be used to process, analyze, and visualize data.
We will also discuss the basics of Machine Learning, including supervised and unsupervised learning, and how to implement them using Python. By the end of this chapter, you will have a solid understanding of Python and its key libraries, setting a strong foundation for the rest of the book.
Let's start with a crash course on Python.
Python is a powerful and versatile high-level programming language that is widely used in various fields, including web development, data analysis, artificial intelligence, and more. Its simplicity and readability make it a great language for beginners, but it can also handle complex tasks and large-scale projects.
In this section, we will delve into the basics of Python, discussing data types, control flow, functions, and classes in detail. We will also explore some of the practical applications of Python in various industries, including finance, healthcare, and education. By the end of this section, you will have a solid understanding of the fundamentals of Python and be able to build simple programs on your own.
It's important to note that this section is not meant to be a comprehensive guide to Python, but rather a quick overview to get you started. If you're already familiar with Python, feel free to skim through this section or move on to the next one, where we will explore more advanced topics in Python programming.
2.1.1 Data Types
Python has several built-in data types, including integers, floats, strings, lists, tuples, sets, and dictionaries. Here's a quick overview:
Integers and Floats
Integers are a type of number that represent whole quantities, while floats, or floating point numbers, are a type of number that represent decimal quantities. Both types of numbers can be used in basic arithmetic operations, such as addition, subtraction, multiplication, and division.
Integers can be used in modulo operations, which find the remainder of a division operation. Floats can also be used in more complex mathematical operations, such as trigonometric functions and logarithms. It is important to note that when performing arithmetic operations with both integers and floats, the result will be a float.
Example:
# Integers
x = 10
y = 2
print(x + y) # Addition
print(x - y) # Subtraction
print(x * y) # Multiplication
print(x / y) # Division
# Floats
a = 1.5
b = 0.5
print(a + b)
print(a - b)
print(a * b)
print(a / b)
Code Purpose:
This code snippet demonstrates performing basic arithmetic operations on integer and floating-point numbers in Python. It showcases the behavior of the addition, subtraction, multiplication, and division operators for both data types.
Step-by-Step Breakdown:
- Integer Arithmetic:
- The code assigns the integer value
10
to the variablex
and2
to the variabley
. - It then performs the following operations using the arithmetic operators:
print(x + y)
: This calculates the sum ofx
andy
(which is 12) and prints the result.print(x - y)
: This calculates the difference ofx
andy
(which is 8) and prints the result.print(x * y)
: This calculates the product ofx
andy
(which is 20) and prints the result.print(x / y)
: This performs integer division ofx
byy
. Since both operands are integers, Python performs integer division by default, resulting in5.0
. Note that the result is a float, even though the operands are integers.
- The code assigns the integer value
- Float Arithmetic:
- The code assigns the floating-point value
1.5
to the variablea
and0.5
to the variableb
. - Similar to integer arithmetic, it performs operations using the same operators and prints the results:
print(a + b)
: This calculates the sum ofa
andb
(which is 2.0) and prints the result.print(a - b)
: This calculates the difference ofa
andb
(which is 1.0) and prints the result.print(a * b)
: This calculates the product ofa
andb
(which is 0.75) and prints the result.print(a / b)
: This performs floating-point division ofa
byb
. Since at least one operand is a float, Python performs division with floating-point precision, resulting in3.0
.
- The code assigns the floating-point value
Key Points:
- Python supports arithmetic operations on both integers (whole numbers) and floats (decimal numbers).
- The behavior of the division operator (
/
) differs for integers and floats.- For integers, it performs integer division, discarding any remainder (resulting in a float if necessary).
- For floats or mixed operand types (one integer and one float), it performs floating-point division, preserving decimal precision.
Strings
Strings are sequences of characters that are used to represent text in programming. They can be created by enclosing characters in single quotes ('') or double quotes (""). Once created, strings can be manipulated in various ways, such as by appending new characters to them or by extracting specific characters from them.
Strings can be formatted to include values that change dynamically, such as dates or user input. This allows for more dynamic and interactive programs that can respond to user input in real time. Overall, strings are a fundamental concept in programming that allow for the representation and manipulation of text-based data.
Example:
s = 'Hello, world!'
print(s)
Lists
Lists are an essential part of programming as they allow ordered collections of items. Lists are mutable, allowing you to make modifications to their content. One example of a list could be a to-do list that you might use to keep track of tasks you need to complete. You could add or remove items from this list as you complete tasks or think of new ones.
In addition to to-do lists, lists can be used for many other purposes, such as storing employee names and phone numbers, a list of your favorite books, or even a list of countries you'd like to visit someday. Lists can also be nested within each other to create more complex structures. For example, a list of to-do lists could be used to categorize your tasks by subject or priority.
Lists are a versatile and integral part of programming that can be used in a variety of ways to help organize and manage data.
Example:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Add an item to the list
fruits.append('date')
# Remove an item from the list
fruits.remove('banana')
# Access an item in the list
print(fruits[0]) # 'apple'
Code Purpose:
This code snippet demonstrates how to create a list in Python and perform common list manipulation operations, including adding, removing, and accessing elements.
Step-by-Step Breakdown:
- Creating a List:
- The line
fruits = ['apple', 'banana', 'cherry']
creates a list namedfruits
. Lists are used to store ordered collections of items in Python. In this case, the listfruits
contains three string elements: "apple", "banana", and "cherry".
- The line
- Adding an Item to the List (Append):
- The line
fruits.append('date')
adds the string element "date" to the end of thefruits
list using the.append
method. Lists are mutable, meaning their contents can be changed after creation. The.append
method is a convenient way to add new items to the end of a list.
- The line
- Removing an Item from the List (Remove):
- The line
fruits.remove('banana')
removes the first occurrence of the string element "banana" from thefruits
list using the.remove
method. It's important to note that the.remove
method removes the element based on its value, not its position in the list. If the element is not found, it will raise aValueError
.
- The line
- Accessing an Item in the List:
- The line
print(fruits[0])
prints the first element of thefruits
list. Lists are indexed starting from 0, sofruits[0]
refers to the element at index 0, which is "apple" in this case.
- The line
Key Points:
- Lists are a fundamental data structure in Python for storing collections of items.
- You can create lists using square brackets
[]
and enclosing elements separated by commas. - The
.append
method provides a way to add items to the end of a list. - The
.remove
method removes the first occurrence of a specified element from the list. - Lists are ordered, and you can access elements by their index within square brackets.
Tuples
Tuples and lists are both data structures in Python. While they share similarities, such as the ability to store multiple values in a single variable, there are also key differences. One of the main differences is that tuples are immutable, meaning that once a tuple is created, you cannot change its content. In contrast, lists are mutable, which means you can add, remove or modify elements after the list is created.
Despite their immutability, tuples are still useful in a number of scenarios. For example, they are commonly used to store related values together, such as the coordinates of a point in space. Tuples can also be used as keys in dictionaries, since they are hashable. Tuples can be used to return multiple values from a function, making them a useful tool for handling complex data.
While tuples may seem limited due to their immutability, they offer a number of advantages and use cases that make them a valuable tool in any Python programmer's toolkit.
Example:
# Create a tuple
coordinates = (10.0, 20.0)
# Access an item in the tuple
print(coordinates[0]) # 10.0
Code Purpose:
This code snippet demonstrates how to create a tuple in Python and access elements by their index.
Step-by-Step Breakdown:
- Creating a Tuple:
- The line
coordinates = (10.0, 20.0)
creates a tuple namedcoordinates
. Tuples are another fundamental data structure in Python used to store ordered collections of elements. However, unlike lists, tuples are immutable, meaning their contents cannot be changed after creation. In this case, the tuplecoordinates
contains two elements:10.0
(a float) and20.0
(a float).
- The line
- Accessing an Item in the Tuple:
- The line
print(coordinates[0])
prints the first element of thecoordinates
tuple. Similar to lists, tuples are indexed starting from 0. So,coordinates[0]
refers to the element at index 0, which is10.0
in this example.
- The line
Key Points:
- Tuples are ordered collections of elements like lists, but they are immutable (unchangeable after creation).
- Tuples are created using parentheses
()
, enclosing elements separated by commas. - You can access elements in tuples by their index within square brackets, similar to lists.
Sets
Sets are unordered collections of unique items. They are useful in various programming scenarios, such as counting unique items in a list or testing for membership. Sets can also be combined using mathematical operations such as union, intersection, and difference. In Python, sets are represented using curly braces and items are separated by commas.
One important characteristic of sets is that they do not allow duplicates. This means that if an item is added to a set that already exists, it will not change the set. Furthermore, sets are mutable, which means that items can be added or removed from the set after it has been created. Overall, sets are a versatile and important tool in programming.
Example:
# Create a set
fruits = {'apple', 'banana', 'cherry', 'apple'}
# Add an item to the set
fruits.add('date')
# Remove an item from the set
fruits.remove('banana')
# Check if an item is in the set
print('apple' in fruits) # True
Code Purpose:
This code snippet demonstrates how to create a set in Python and perform common set operations, including adding, removing, and checking for element membership.
Step-by-Step Breakdown:
- Creating a Set:
- The line
fruits = {'apple', 'banana', 'cherry', 'apple'}
creates a set namedfruits
. Sets are used to store collections of unique elements in Python. Unlike lists, sets do not allow duplicate elements. In this case, the setfruits
is created with four elements: "apple", "banana", "cherry", and "apple". However, since sets don't allow duplicates, the second occurrence of "apple" is ignored.
- The line
- Adding an Item to the Set (Add):
- The line
fruits.add('date')
adds the string element "date" to thefruits
set using the.add
method. Sets are mutable, meaning their contents can be changed after creation. The.add
method is a way to add new, unique elements to a set.
- The line
- Removing an Item from the Set (Remove):
- The line
fruits.remove('banana')
removes the element "banana" from thefruits
set using the.remove
method. Similar to lists, it removes the first occurrence of the specified element. It's important to note that.remove
will raise aKeyError
if the element is not found in the set.
- The line
- Checking for Membership:
- The line
print('apple' in fruits)
checks if the element "apple" exists in thefruits
set using thein
operator. Thein
operator returnsTrue
if the element is found in the set, andFalse
otherwise. In this case, it printsTrue
because "apple" is indeed in the set.
- The line
Key Points:
- Sets are collections of unique elements, ideal for storing unordered collections where duplicates are not allowed.
- You create sets using curly braces
{}
and enclosing elements separated by commas. - The
.add
method adds unique elements to a set. - The
.remove
method removes an element from the set, raising an error if not found. - The
in
operator checks if an element exists within a set.
Dictionaries
Dictionaries are data structures that contain key-value pairs, allowing for efficient lookups and storage of information. These pairs can represent any type of data, such as strings, numbers, or even other dictionaries. In addition to lookup and storage, dictionaries can also be used for data manipulation and analysis.
With the flexibility and versatility of dictionaries, they have become a staple in many programming languages, used in applications ranging from database management to natural language processing.
Example:
# Create a dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Access a value in the dictionary
print(person['name']) # 'Alice'
# Change a value in the dictionary
person['age'] = 26
# Add a new key-value pair to the dictionary
person['job'] = 'Engineer'
# Remove a key-value pair from the dictionary
del person['city']
# Print the modified dictionary
print(person)
Code Purpose:
This code snippet demonstrates how to create a dictionary in Python, access and modify its elements, and perform common operations like adding and removing key-value pairs.
Step-by-Step Breakdown:
- Creating a Dictionary:
- The line
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
creates a dictionary namedperson
. Dictionaries are fundamental data structures in Python for storing collections of data in a key-value format. In this case, theperson
dictionary stores three key-value pairs:- Key: 'name', Value: 'Alice' (associates the name "Alice" with the key 'name')
- Key: 'age', Value: 25 (associates the age 25 with the key 'age')
- Key: 'city', Value: 'New York' (associates the city "New York" with the key 'city')
- The line
- Accessing a Value in the Dictionary:
- The line
print(person['name'])
retrieves the value associated with the key 'name' from theperson
dictionary and prints it. Since 'name' is a key, it retrieves the corresponding value, which is "Alice" in this case.
- The line
- Changing a Value in the Dictionary:
- The line
person['age'] = 26
modifies the value associated with the key 'age' in theperson
dictionary. Dictionaries are mutable, meaning you can change their contents after creation. Here, we update the age to 26.
- The line
- Adding a New Key-Value Pair:
- The line
person['job'] = 'Engineer'
adds a new key-value pair to theperson
dictionary. The key is 'job', and the value is 'Engineer'. This extends the dictionary with new information.
- The line
- Removing a Key-Value Pair:
- The line
del person['city']
removes the key-value pair with the key 'city' from theperson
dictionary. Thedel
keyword is used for deletion.
- The line
- Printing the Modified Dictionary:
- The line
print(person)
prints the contents of the modifiedperson
dictionary. This will display the updated dictionary without the 'city' key-value pair and the changed age.
- The line
Key Points:
- Dictionaries are collections of key-value pairs, providing a flexible way to store and access data.
- You create dictionaries using curly braces
{}
with keys and values separated by colons (:
). Keys must be unique and immutable (e.g., strings, numbers). - Accessing elements is done using the key within square brackets
[]
. - You can modify existing values or add new key-value pairs using assignment.
- The
del
keyword removes key-value pairs from the dictionary.
2.1.2 Control Flow
Control flow is a crucial concept in programming. It refers to the order in which the code of a program is executed. In Python, there are several mechanisms that can be used to control the flow of a program.
One of these is the if
statement, which allows the program to make decisions based on certain conditions. Another important mechanism is the for
loop, which is used to iterate over a sequence of values or elements. Similarly, the while
loop can be used to execute a block of code repeatedly as long as a certain condition is met.
In addition to these mechanisms, Python also provides try
/except
blocks, which are used for error handling. These blocks are particularly useful when a program encounters an error or an exception that it cannot handle, as they allow the program to gracefully recover from the error and continue executing its code.
It is important for programmers to have a solid understanding of control flow and the different mechanisms that can be used to control it in Python. By using these mechanisms effectively, programmers can create programs that are both efficient and robust, and that can handle a wide range of different scenarios and situations.
If Statements
If
statements are used for conditional execution, allowing a program to make decisions based on certain conditions. This is a powerful tool that gives developers more control over the flow of their code by allowing them to specify what should happen under certain circumstances.
For example, an if
statement could be used to check if a user has entered the correct password, and if so, grant them access to a restricted area of a website. Alternatively, it could be used to check if a user has entered an invalid input, and prompt them to try again.
By using if
statements, developers can create more dynamic and versatile programs that can respond to a wider range of user inputs and conditions.
Example:
x = 10
if x > 0:
print('x is positive')
elif x < 0:
print('x is negative')
else:
print('x is zero')
For Loops
For
loops are an essential tool in programming. They are used for iterating over a sequence (like a list or a string), making it possible to perform operations on each element of the sequence. This is incredibly useful in a wide variety of contexts.
For example, imagine you have a list of items and you want to perform the same calculation on each item. A for
loop makes this task simple and efficient. By iterating over the list, the loop performs the calculation on each item in turn, saving you from having to write the same code over and over again.
In addition to lists and strings, for
loops can also be used with other types of sequences, such as dictionaries and sets, making them an incredibly versatile tool for any programmer to have in their toolkit.
Example:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
While Loops
While
loops are used for repeated execution as long as a condition is true. This means that if the condition is false from the outset, the loop will never execute. It is important to ensure that the condition will eventually become false, otherwise the loop will continue indefinitely.
In practice, while
loops can be useful for situations where you do not know how many iterations will be required. For example, if you are writing a program to calculate the square root of a number, you may not know how many iterations will be required until you get the desired level of precision.
Another use of while
loops is to repeatedly ask the user for input until they provide valid input. This can be useful to ensure that the program does not crash or behave unexpectedly due to invalid user input.
Overall, while
loops are a powerful programming construct that can be used to solve a wide variety of problems. By using them effectively, you can write code that is more efficient, easier to read, and easier to maintain.
Example:
x = 0
while x < 5:
print(x)
x += 1
2.1.3 Try/Except Blocks
Try
/except
blocks are a key part of Python programming for error handling. These blocks allow the programmer to anticipate and handle errors that may occur during the execution of a Python script. By using try/except blocks, a programmer can create a more robust and error-resistant program that can handle unexpected input or other errors without crashing. In fact, try/except blocks are so commonly used in Python programming that they are often considered a fundamental aspect of the language's syntax and functionality.
Example:
try:
x = 1 / 0 # This will raise a ZeroDivisionError
except ZeroDivisionError:
print('You cannot divide by zero!')
2.1.4 Functions
Functions are a key concept in programming that allow for the creation of reusable pieces of code. When defining a function in Python, the def
keyword is used. Functions can contain any number of statements, including loops, conditional statements, and other functions.
Additionally, functions can have parameters and return values, which allows for greater flexibility in how they are used. By breaking down a problem into smaller functions, code can be made more readable and easier to maintain.
Functions are an essential tool for any programmer looking to improve the efficiency and effectiveness of their code.
Example:
def greet(name):
return f'Hello, {name}!'
print(greet('Alice')) # 'Hello, Alice!'
2.1.5 Classes
Object-oriented programming is centered around the concept of classes. A class is essentially a blueprint that describes the properties, methods, and behaviors of objects that are created from it.
These objects can be thought of as instances of the class, each with their own unique set of values for the properties defined by the class. When you create an object from a class, you are essentially instantiating it, meaning that you are creating a new instance of the class with its own set of values.
Once an object has been created, you can then call its methods to perform various tasks. By defining these methods within the class, you can ensure that the same functionality is available to all instances of the class.
Classes provide a powerful mechanism for organizing and managing complex code, allowing you to create reusable, modular code that can be shared across multiple projects.
Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f'Hello, my name is {self.name} and I am {self.age} years old.'
alice = Person('Alice', 25)
print(alice.greet()) # 'Hello, my name is Alice and I am 25 years old.'
2.1.6 Built-in Functions and Modules
Python offers an extensive range of built-in functions and modules that provide additional functionality to the language. These modules are designed to be used in a variety of applications, from data analysis to web development and beyond.
For example, the math module provides access to mathematical functions such as trigonometry, while the os module enables interaction with the operating system. Python's standard library includes modules for working with regular expressions, file I/O, and network communication, to name just a few.
With such a wide array of tools at your disposal, Python is a powerful language that can handle a vast range of tasks and applications.
Here are some of the most commonly used ones:
Built-in Functions
Python has many built-in functions that perform a variety of tasks. Here are a few examples:
print()
: Prints the specified message to the screen.len()
: Returns the number of items in an object.type()
: Returns the type of an object.str()
,int()
,float()
: Convert an object to a string, integer, or float, respectively.input()
: Reads a line from input (keyboard), converts it to a string, and returns it.
Here's how you can use these functions:
print(len('Hello, world!')) # 13
print(type(10)) # <class 'int'>
print(int('10')) # 10
name = input('What is your name? ')
Built-in Modules
Python also comes with a set of built-in modules that you can import into your program to use. Here are a few examples:
math
: Provides mathematical functions.random
: Provides functions for generating random numbers.datetime
: Provides functions for manipulating dates and times.os
: Provides functions for interacting with the operating system.
Here's how you can use these modules:
import math
print(math.sqrt(16)) # 4.0
import random
print(random.randint(1, 10)) # a random integer between 1 and 10
import datetime
print(datetime.datetime.now()) # current date and time
import os
print(os.getcwd()) # current working directory
This concludes our Python crash course. While this section only scratches the surface of what Python can do, it should give you a good foundation to build upon. In the next sections, we will explore some of the key Python libraries used in Machine Learning.
If you want to gain a better and deeper understanding of Python basics, this book may be of interest to you:
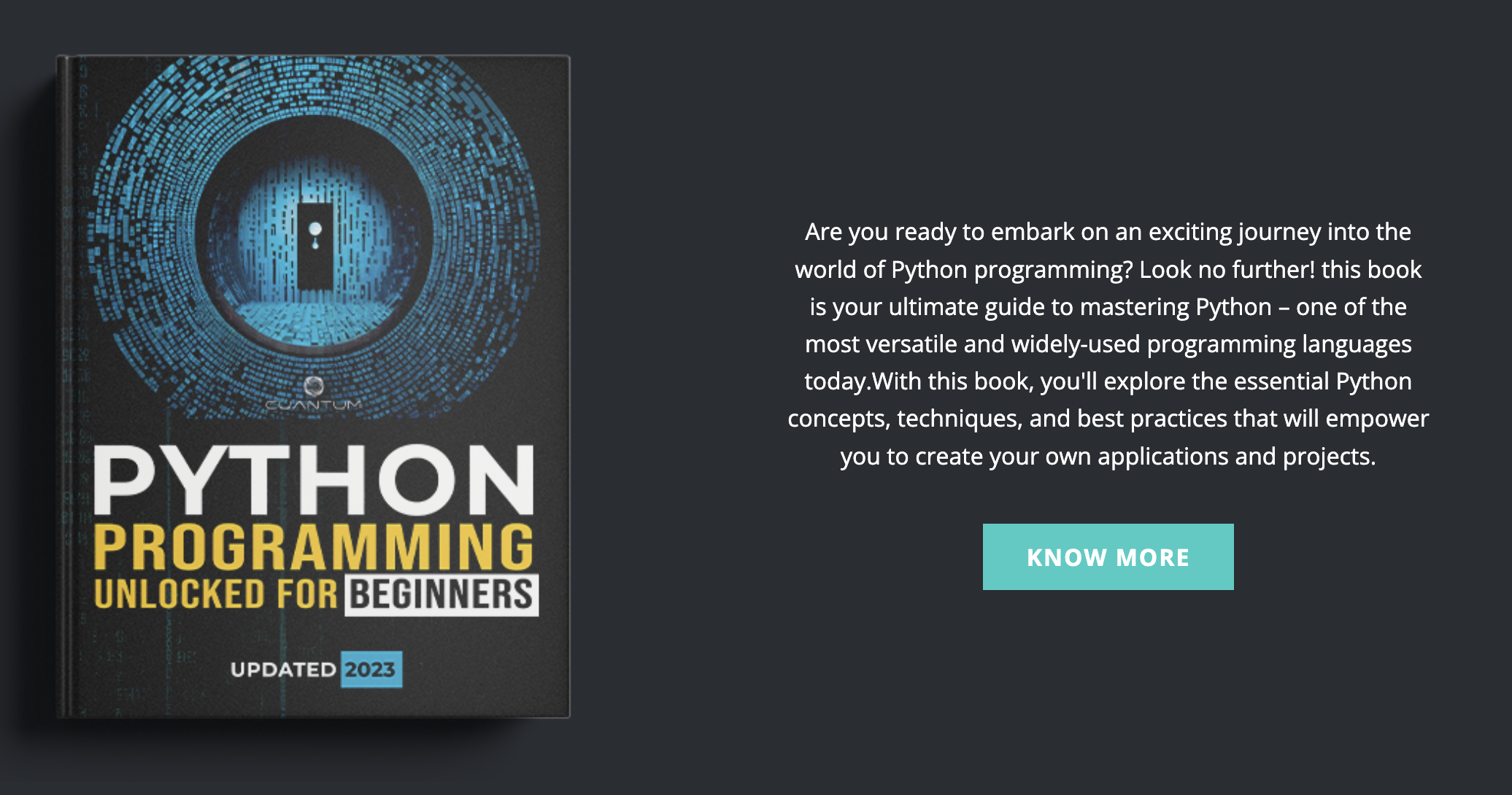
2.1 Python Crash Course
Welcome to Chapter 2! Here, we will take a deeper dive into the world of Python and its essential libraries that are widely used in the field of Machine Learning. As you may already know, Python has become the go-to language for many data scientists and machine learning engineers due to its simplicity and extensive libraries. Python's popularity is largely due to the fact that it is an open-source programming language that is easy to learn and use.
Throughout this chapter, we will cover a range of topics, from the basics of Python programming to the most essential libraries used in Machine Learning. We will start by discussing Python syntax and data types, including variables, loops, conditions, and functions. Next, we will explore some of the most essential libraries used in Machine Learning, such as NumPy, Pandas, and Matplotlib, and how they can be used to process, analyze, and visualize data.
We will also discuss the basics of Machine Learning, including supervised and unsupervised learning, and how to implement them using Python. By the end of this chapter, you will have a solid understanding of Python and its key libraries, setting a strong foundation for the rest of the book.
Let's start with a crash course on Python.
Python is a powerful and versatile high-level programming language that is widely used in various fields, including web development, data analysis, artificial intelligence, and more. Its simplicity and readability make it a great language for beginners, but it can also handle complex tasks and large-scale projects.
In this section, we will delve into the basics of Python, discussing data types, control flow, functions, and classes in detail. We will also explore some of the practical applications of Python in various industries, including finance, healthcare, and education. By the end of this section, you will have a solid understanding of the fundamentals of Python and be able to build simple programs on your own.
It's important to note that this section is not meant to be a comprehensive guide to Python, but rather a quick overview to get you started. If you're already familiar with Python, feel free to skim through this section or move on to the next one, where we will explore more advanced topics in Python programming.
2.1.1 Data Types
Python has several built-in data types, including integers, floats, strings, lists, tuples, sets, and dictionaries. Here's a quick overview:
Integers and Floats
Integers are a type of number that represent whole quantities, while floats, or floating point numbers, are a type of number that represent decimal quantities. Both types of numbers can be used in basic arithmetic operations, such as addition, subtraction, multiplication, and division.
Integers can be used in modulo operations, which find the remainder of a division operation. Floats can also be used in more complex mathematical operations, such as trigonometric functions and logarithms. It is important to note that when performing arithmetic operations with both integers and floats, the result will be a float.
Example:
# Integers
x = 10
y = 2
print(x + y) # Addition
print(x - y) # Subtraction
print(x * y) # Multiplication
print(x / y) # Division
# Floats
a = 1.5
b = 0.5
print(a + b)
print(a - b)
print(a * b)
print(a / b)
Code Purpose:
This code snippet demonstrates performing basic arithmetic operations on integer and floating-point numbers in Python. It showcases the behavior of the addition, subtraction, multiplication, and division operators for both data types.
Step-by-Step Breakdown:
- Integer Arithmetic:
- The code assigns the integer value
10
to the variablex
and2
to the variabley
. - It then performs the following operations using the arithmetic operators:
print(x + y)
: This calculates the sum ofx
andy
(which is 12) and prints the result.print(x - y)
: This calculates the difference ofx
andy
(which is 8) and prints the result.print(x * y)
: This calculates the product ofx
andy
(which is 20) and prints the result.print(x / y)
: This performs integer division ofx
byy
. Since both operands are integers, Python performs integer division by default, resulting in5.0
. Note that the result is a float, even though the operands are integers.
- The code assigns the integer value
- Float Arithmetic:
- The code assigns the floating-point value
1.5
to the variablea
and0.5
to the variableb
. - Similar to integer arithmetic, it performs operations using the same operators and prints the results:
print(a + b)
: This calculates the sum ofa
andb
(which is 2.0) and prints the result.print(a - b)
: This calculates the difference ofa
andb
(which is 1.0) and prints the result.print(a * b)
: This calculates the product ofa
andb
(which is 0.75) and prints the result.print(a / b)
: This performs floating-point division ofa
byb
. Since at least one operand is a float, Python performs division with floating-point precision, resulting in3.0
.
- The code assigns the floating-point value
Key Points:
- Python supports arithmetic operations on both integers (whole numbers) and floats (decimal numbers).
- The behavior of the division operator (
/
) differs for integers and floats.- For integers, it performs integer division, discarding any remainder (resulting in a float if necessary).
- For floats or mixed operand types (one integer and one float), it performs floating-point division, preserving decimal precision.
Strings
Strings are sequences of characters that are used to represent text in programming. They can be created by enclosing characters in single quotes ('') or double quotes (""). Once created, strings can be manipulated in various ways, such as by appending new characters to them or by extracting specific characters from them.
Strings can be formatted to include values that change dynamically, such as dates or user input. This allows for more dynamic and interactive programs that can respond to user input in real time. Overall, strings are a fundamental concept in programming that allow for the representation and manipulation of text-based data.
Example:
s = 'Hello, world!'
print(s)
Lists
Lists are an essential part of programming as they allow ordered collections of items. Lists are mutable, allowing you to make modifications to their content. One example of a list could be a to-do list that you might use to keep track of tasks you need to complete. You could add or remove items from this list as you complete tasks or think of new ones.
In addition to to-do lists, lists can be used for many other purposes, such as storing employee names and phone numbers, a list of your favorite books, or even a list of countries you'd like to visit someday. Lists can also be nested within each other to create more complex structures. For example, a list of to-do lists could be used to categorize your tasks by subject or priority.
Lists are a versatile and integral part of programming that can be used in a variety of ways to help organize and manage data.
Example:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Add an item to the list
fruits.append('date')
# Remove an item from the list
fruits.remove('banana')
# Access an item in the list
print(fruits[0]) # 'apple'
Code Purpose:
This code snippet demonstrates how to create a list in Python and perform common list manipulation operations, including adding, removing, and accessing elements.
Step-by-Step Breakdown:
- Creating a List:
- The line
fruits = ['apple', 'banana', 'cherry']
creates a list namedfruits
. Lists are used to store ordered collections of items in Python. In this case, the listfruits
contains three string elements: "apple", "banana", and "cherry".
- The line
- Adding an Item to the List (Append):
- The line
fruits.append('date')
adds the string element "date" to the end of thefruits
list using the.append
method. Lists are mutable, meaning their contents can be changed after creation. The.append
method is a convenient way to add new items to the end of a list.
- The line
- Removing an Item from the List (Remove):
- The line
fruits.remove('banana')
removes the first occurrence of the string element "banana" from thefruits
list using the.remove
method. It's important to note that the.remove
method removes the element based on its value, not its position in the list. If the element is not found, it will raise aValueError
.
- The line
- Accessing an Item in the List:
- The line
print(fruits[0])
prints the first element of thefruits
list. Lists are indexed starting from 0, sofruits[0]
refers to the element at index 0, which is "apple" in this case.
- The line
Key Points:
- Lists are a fundamental data structure in Python for storing collections of items.
- You can create lists using square brackets
[]
and enclosing elements separated by commas. - The
.append
method provides a way to add items to the end of a list. - The
.remove
method removes the first occurrence of a specified element from the list. - Lists are ordered, and you can access elements by their index within square brackets.
Tuples
Tuples and lists are both data structures in Python. While they share similarities, such as the ability to store multiple values in a single variable, there are also key differences. One of the main differences is that tuples are immutable, meaning that once a tuple is created, you cannot change its content. In contrast, lists are mutable, which means you can add, remove or modify elements after the list is created.
Despite their immutability, tuples are still useful in a number of scenarios. For example, they are commonly used to store related values together, such as the coordinates of a point in space. Tuples can also be used as keys in dictionaries, since they are hashable. Tuples can be used to return multiple values from a function, making them a useful tool for handling complex data.
While tuples may seem limited due to their immutability, they offer a number of advantages and use cases that make them a valuable tool in any Python programmer's toolkit.
Example:
# Create a tuple
coordinates = (10.0, 20.0)
# Access an item in the tuple
print(coordinates[0]) # 10.0
Code Purpose:
This code snippet demonstrates how to create a tuple in Python and access elements by their index.
Step-by-Step Breakdown:
- Creating a Tuple:
- The line
coordinates = (10.0, 20.0)
creates a tuple namedcoordinates
. Tuples are another fundamental data structure in Python used to store ordered collections of elements. However, unlike lists, tuples are immutable, meaning their contents cannot be changed after creation. In this case, the tuplecoordinates
contains two elements:10.0
(a float) and20.0
(a float).
- The line
- Accessing an Item in the Tuple:
- The line
print(coordinates[0])
prints the first element of thecoordinates
tuple. Similar to lists, tuples are indexed starting from 0. So,coordinates[0]
refers to the element at index 0, which is10.0
in this example.
- The line
Key Points:
- Tuples are ordered collections of elements like lists, but they are immutable (unchangeable after creation).
- Tuples are created using parentheses
()
, enclosing elements separated by commas. - You can access elements in tuples by their index within square brackets, similar to lists.
Sets
Sets are unordered collections of unique items. They are useful in various programming scenarios, such as counting unique items in a list or testing for membership. Sets can also be combined using mathematical operations such as union, intersection, and difference. In Python, sets are represented using curly braces and items are separated by commas.
One important characteristic of sets is that they do not allow duplicates. This means that if an item is added to a set that already exists, it will not change the set. Furthermore, sets are mutable, which means that items can be added or removed from the set after it has been created. Overall, sets are a versatile and important tool in programming.
Example:
# Create a set
fruits = {'apple', 'banana', 'cherry', 'apple'}
# Add an item to the set
fruits.add('date')
# Remove an item from the set
fruits.remove('banana')
# Check if an item is in the set
print('apple' in fruits) # True
Code Purpose:
This code snippet demonstrates how to create a set in Python and perform common set operations, including adding, removing, and checking for element membership.
Step-by-Step Breakdown:
- Creating a Set:
- The line
fruits = {'apple', 'banana', 'cherry', 'apple'}
creates a set namedfruits
. Sets are used to store collections of unique elements in Python. Unlike lists, sets do not allow duplicate elements. In this case, the setfruits
is created with four elements: "apple", "banana", "cherry", and "apple". However, since sets don't allow duplicates, the second occurrence of "apple" is ignored.
- The line
- Adding an Item to the Set (Add):
- The line
fruits.add('date')
adds the string element "date" to thefruits
set using the.add
method. Sets are mutable, meaning their contents can be changed after creation. The.add
method is a way to add new, unique elements to a set.
- The line
- Removing an Item from the Set (Remove):
- The line
fruits.remove('banana')
removes the element "banana" from thefruits
set using the.remove
method. Similar to lists, it removes the first occurrence of the specified element. It's important to note that.remove
will raise aKeyError
if the element is not found in the set.
- The line
- Checking for Membership:
- The line
print('apple' in fruits)
checks if the element "apple" exists in thefruits
set using thein
operator. Thein
operator returnsTrue
if the element is found in the set, andFalse
otherwise. In this case, it printsTrue
because "apple" is indeed in the set.
- The line
Key Points:
- Sets are collections of unique elements, ideal for storing unordered collections where duplicates are not allowed.
- You create sets using curly braces
{}
and enclosing elements separated by commas. - The
.add
method adds unique elements to a set. - The
.remove
method removes an element from the set, raising an error if not found. - The
in
operator checks if an element exists within a set.
Dictionaries
Dictionaries are data structures that contain key-value pairs, allowing for efficient lookups and storage of information. These pairs can represent any type of data, such as strings, numbers, or even other dictionaries. In addition to lookup and storage, dictionaries can also be used for data manipulation and analysis.
With the flexibility and versatility of dictionaries, they have become a staple in many programming languages, used in applications ranging from database management to natural language processing.
Example:
# Create a dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Access a value in the dictionary
print(person['name']) # 'Alice'
# Change a value in the dictionary
person['age'] = 26
# Add a new key-value pair to the dictionary
person['job'] = 'Engineer'
# Remove a key-value pair from the dictionary
del person['city']
# Print the modified dictionary
print(person)
Code Purpose:
This code snippet demonstrates how to create a dictionary in Python, access and modify its elements, and perform common operations like adding and removing key-value pairs.
Step-by-Step Breakdown:
- Creating a Dictionary:
- The line
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
creates a dictionary namedperson
. Dictionaries are fundamental data structures in Python for storing collections of data in a key-value format. In this case, theperson
dictionary stores three key-value pairs:- Key: 'name', Value: 'Alice' (associates the name "Alice" with the key 'name')
- Key: 'age', Value: 25 (associates the age 25 with the key 'age')
- Key: 'city', Value: 'New York' (associates the city "New York" with the key 'city')
- The line
- Accessing a Value in the Dictionary:
- The line
print(person['name'])
retrieves the value associated with the key 'name' from theperson
dictionary and prints it. Since 'name' is a key, it retrieves the corresponding value, which is "Alice" in this case.
- The line
- Changing a Value in the Dictionary:
- The line
person['age'] = 26
modifies the value associated with the key 'age' in theperson
dictionary. Dictionaries are mutable, meaning you can change their contents after creation. Here, we update the age to 26.
- The line
- Adding a New Key-Value Pair:
- The line
person['job'] = 'Engineer'
adds a new key-value pair to theperson
dictionary. The key is 'job', and the value is 'Engineer'. This extends the dictionary with new information.
- The line
- Removing a Key-Value Pair:
- The line
del person['city']
removes the key-value pair with the key 'city' from theperson
dictionary. Thedel
keyword is used for deletion.
- The line
- Printing the Modified Dictionary:
- The line
print(person)
prints the contents of the modifiedperson
dictionary. This will display the updated dictionary without the 'city' key-value pair and the changed age.
- The line
Key Points:
- Dictionaries are collections of key-value pairs, providing a flexible way to store and access data.
- You create dictionaries using curly braces
{}
with keys and values separated by colons (:
). Keys must be unique and immutable (e.g., strings, numbers). - Accessing elements is done using the key within square brackets
[]
. - You can modify existing values or add new key-value pairs using assignment.
- The
del
keyword removes key-value pairs from the dictionary.
2.1.2 Control Flow
Control flow is a crucial concept in programming. It refers to the order in which the code of a program is executed. In Python, there are several mechanisms that can be used to control the flow of a program.
One of these is the if
statement, which allows the program to make decisions based on certain conditions. Another important mechanism is the for
loop, which is used to iterate over a sequence of values or elements. Similarly, the while
loop can be used to execute a block of code repeatedly as long as a certain condition is met.
In addition to these mechanisms, Python also provides try
/except
blocks, which are used for error handling. These blocks are particularly useful when a program encounters an error or an exception that it cannot handle, as they allow the program to gracefully recover from the error and continue executing its code.
It is important for programmers to have a solid understanding of control flow and the different mechanisms that can be used to control it in Python. By using these mechanisms effectively, programmers can create programs that are both efficient and robust, and that can handle a wide range of different scenarios and situations.
If Statements
If
statements are used for conditional execution, allowing a program to make decisions based on certain conditions. This is a powerful tool that gives developers more control over the flow of their code by allowing them to specify what should happen under certain circumstances.
For example, an if
statement could be used to check if a user has entered the correct password, and if so, grant them access to a restricted area of a website. Alternatively, it could be used to check if a user has entered an invalid input, and prompt them to try again.
By using if
statements, developers can create more dynamic and versatile programs that can respond to a wider range of user inputs and conditions.
Example:
x = 10
if x > 0:
print('x is positive')
elif x < 0:
print('x is negative')
else:
print('x is zero')
For Loops
For
loops are an essential tool in programming. They are used for iterating over a sequence (like a list or a string), making it possible to perform operations on each element of the sequence. This is incredibly useful in a wide variety of contexts.
For example, imagine you have a list of items and you want to perform the same calculation on each item. A for
loop makes this task simple and efficient. By iterating over the list, the loop performs the calculation on each item in turn, saving you from having to write the same code over and over again.
In addition to lists and strings, for
loops can also be used with other types of sequences, such as dictionaries and sets, making them an incredibly versatile tool for any programmer to have in their toolkit.
Example:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
While Loops
While
loops are used for repeated execution as long as a condition is true. This means that if the condition is false from the outset, the loop will never execute. It is important to ensure that the condition will eventually become false, otherwise the loop will continue indefinitely.
In practice, while
loops can be useful for situations where you do not know how many iterations will be required. For example, if you are writing a program to calculate the square root of a number, you may not know how many iterations will be required until you get the desired level of precision.
Another use of while
loops is to repeatedly ask the user for input until they provide valid input. This can be useful to ensure that the program does not crash or behave unexpectedly due to invalid user input.
Overall, while
loops are a powerful programming construct that can be used to solve a wide variety of problems. By using them effectively, you can write code that is more efficient, easier to read, and easier to maintain.
Example:
x = 0
while x < 5:
print(x)
x += 1
2.1.3 Try/Except Blocks
Try
/except
blocks are a key part of Python programming for error handling. These blocks allow the programmer to anticipate and handle errors that may occur during the execution of a Python script. By using try/except blocks, a programmer can create a more robust and error-resistant program that can handle unexpected input or other errors without crashing. In fact, try/except blocks are so commonly used in Python programming that they are often considered a fundamental aspect of the language's syntax and functionality.
Example:
try:
x = 1 / 0 # This will raise a ZeroDivisionError
except ZeroDivisionError:
print('You cannot divide by zero!')
2.1.4 Functions
Functions are a key concept in programming that allow for the creation of reusable pieces of code. When defining a function in Python, the def
keyword is used. Functions can contain any number of statements, including loops, conditional statements, and other functions.
Additionally, functions can have parameters and return values, which allows for greater flexibility in how they are used. By breaking down a problem into smaller functions, code can be made more readable and easier to maintain.
Functions are an essential tool for any programmer looking to improve the efficiency and effectiveness of their code.
Example:
def greet(name):
return f'Hello, {name}!'
print(greet('Alice')) # 'Hello, Alice!'
2.1.5 Classes
Object-oriented programming is centered around the concept of classes. A class is essentially a blueprint that describes the properties, methods, and behaviors of objects that are created from it.
These objects can be thought of as instances of the class, each with their own unique set of values for the properties defined by the class. When you create an object from a class, you are essentially instantiating it, meaning that you are creating a new instance of the class with its own set of values.
Once an object has been created, you can then call its methods to perform various tasks. By defining these methods within the class, you can ensure that the same functionality is available to all instances of the class.
Classes provide a powerful mechanism for organizing and managing complex code, allowing you to create reusable, modular code that can be shared across multiple projects.
Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f'Hello, my name is {self.name} and I am {self.age} years old.'
alice = Person('Alice', 25)
print(alice.greet()) # 'Hello, my name is Alice and I am 25 years old.'
2.1.6 Built-in Functions and Modules
Python offers an extensive range of built-in functions and modules that provide additional functionality to the language. These modules are designed to be used in a variety of applications, from data analysis to web development and beyond.
For example, the math module provides access to mathematical functions such as trigonometry, while the os module enables interaction with the operating system. Python's standard library includes modules for working with regular expressions, file I/O, and network communication, to name just a few.
With such a wide array of tools at your disposal, Python is a powerful language that can handle a vast range of tasks and applications.
Here are some of the most commonly used ones:
Built-in Functions
Python has many built-in functions that perform a variety of tasks. Here are a few examples:
print()
: Prints the specified message to the screen.len()
: Returns the number of items in an object.type()
: Returns the type of an object.str()
,int()
,float()
: Convert an object to a string, integer, or float, respectively.input()
: Reads a line from input (keyboard), converts it to a string, and returns it.
Here's how you can use these functions:
print(len('Hello, world!')) # 13
print(type(10)) # <class 'int'>
print(int('10')) # 10
name = input('What is your name? ')
Built-in Modules
Python also comes with a set of built-in modules that you can import into your program to use. Here are a few examples:
math
: Provides mathematical functions.random
: Provides functions for generating random numbers.datetime
: Provides functions for manipulating dates and times.os
: Provides functions for interacting with the operating system.
Here's how you can use these modules:
import math
print(math.sqrt(16)) # 4.0
import random
print(random.randint(1, 10)) # a random integer between 1 and 10
import datetime
print(datetime.datetime.now()) # current date and time
import os
print(os.getcwd()) # current working directory
This concludes our Python crash course. While this section only scratches the surface of what Python can do, it should give you a good foundation to build upon. In the next sections, we will explore some of the key Python libraries used in Machine Learning.
If you want to gain a better and deeper understanding of Python basics, this book may be of interest to you:
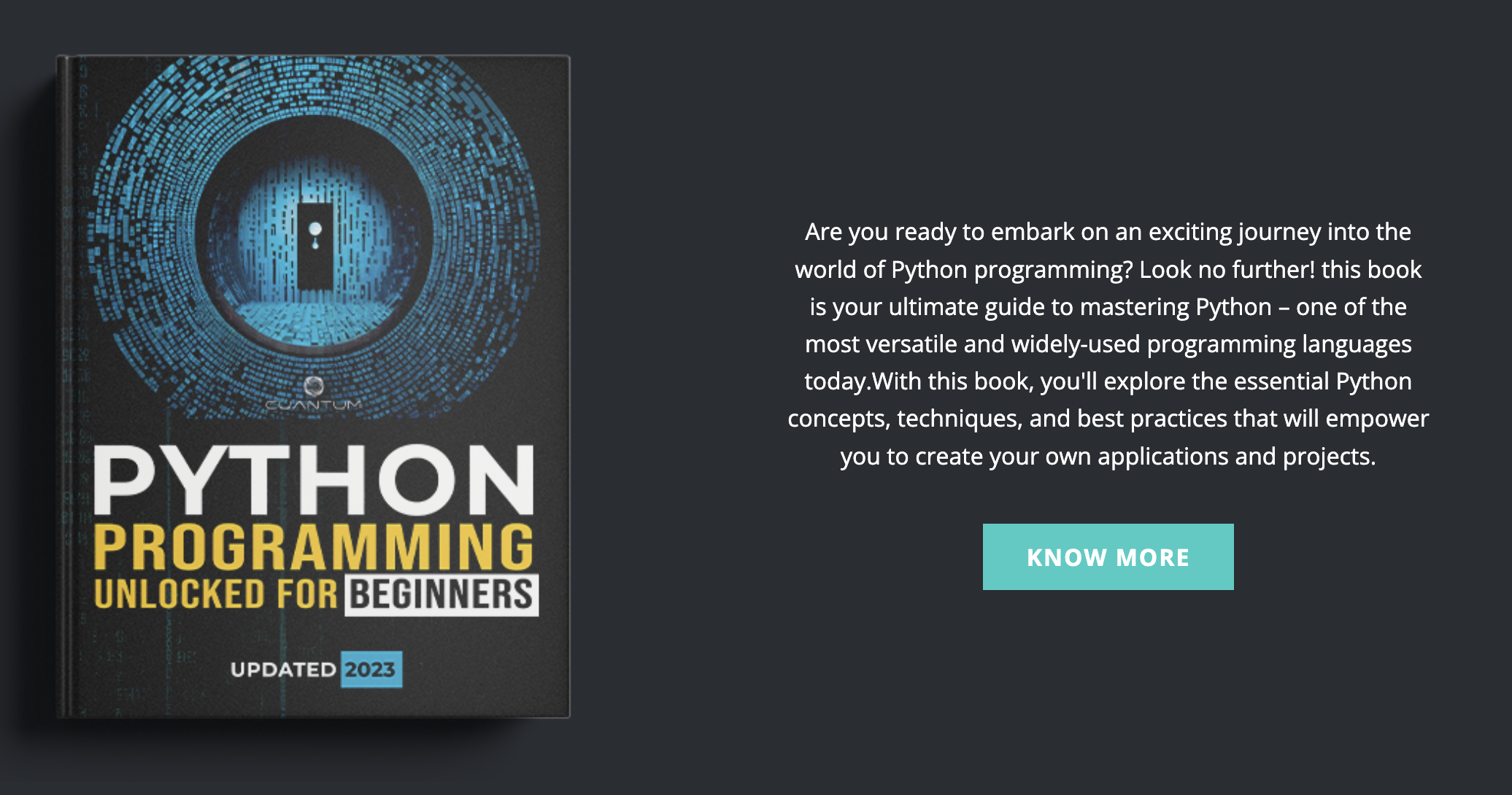
2.1 Python Crash Course
Welcome to Chapter 2! Here, we will take a deeper dive into the world of Python and its essential libraries that are widely used in the field of Machine Learning. As you may already know, Python has become the go-to language for many data scientists and machine learning engineers due to its simplicity and extensive libraries. Python's popularity is largely due to the fact that it is an open-source programming language that is easy to learn and use.
Throughout this chapter, we will cover a range of topics, from the basics of Python programming to the most essential libraries used in Machine Learning. We will start by discussing Python syntax and data types, including variables, loops, conditions, and functions. Next, we will explore some of the most essential libraries used in Machine Learning, such as NumPy, Pandas, and Matplotlib, and how they can be used to process, analyze, and visualize data.
We will also discuss the basics of Machine Learning, including supervised and unsupervised learning, and how to implement them using Python. By the end of this chapter, you will have a solid understanding of Python and its key libraries, setting a strong foundation for the rest of the book.
Let's start with a crash course on Python.
Python is a powerful and versatile high-level programming language that is widely used in various fields, including web development, data analysis, artificial intelligence, and more. Its simplicity and readability make it a great language for beginners, but it can also handle complex tasks and large-scale projects.
In this section, we will delve into the basics of Python, discussing data types, control flow, functions, and classes in detail. We will also explore some of the practical applications of Python in various industries, including finance, healthcare, and education. By the end of this section, you will have a solid understanding of the fundamentals of Python and be able to build simple programs on your own.
It's important to note that this section is not meant to be a comprehensive guide to Python, but rather a quick overview to get you started. If you're already familiar with Python, feel free to skim through this section or move on to the next one, where we will explore more advanced topics in Python programming.
2.1.1 Data Types
Python has several built-in data types, including integers, floats, strings, lists, tuples, sets, and dictionaries. Here's a quick overview:
Integers and Floats
Integers are a type of number that represent whole quantities, while floats, or floating point numbers, are a type of number that represent decimal quantities. Both types of numbers can be used in basic arithmetic operations, such as addition, subtraction, multiplication, and division.
Integers can be used in modulo operations, which find the remainder of a division operation. Floats can also be used in more complex mathematical operations, such as trigonometric functions and logarithms. It is important to note that when performing arithmetic operations with both integers and floats, the result will be a float.
Example:
# Integers
x = 10
y = 2
print(x + y) # Addition
print(x - y) # Subtraction
print(x * y) # Multiplication
print(x / y) # Division
# Floats
a = 1.5
b = 0.5
print(a + b)
print(a - b)
print(a * b)
print(a / b)
Code Purpose:
This code snippet demonstrates performing basic arithmetic operations on integer and floating-point numbers in Python. It showcases the behavior of the addition, subtraction, multiplication, and division operators for both data types.
Step-by-Step Breakdown:
- Integer Arithmetic:
- The code assigns the integer value
10
to the variablex
and2
to the variabley
. - It then performs the following operations using the arithmetic operators:
print(x + y)
: This calculates the sum ofx
andy
(which is 12) and prints the result.print(x - y)
: This calculates the difference ofx
andy
(which is 8) and prints the result.print(x * y)
: This calculates the product ofx
andy
(which is 20) and prints the result.print(x / y)
: This performs integer division ofx
byy
. Since both operands are integers, Python performs integer division by default, resulting in5.0
. Note that the result is a float, even though the operands are integers.
- The code assigns the integer value
- Float Arithmetic:
- The code assigns the floating-point value
1.5
to the variablea
and0.5
to the variableb
. - Similar to integer arithmetic, it performs operations using the same operators and prints the results:
print(a + b)
: This calculates the sum ofa
andb
(which is 2.0) and prints the result.print(a - b)
: This calculates the difference ofa
andb
(which is 1.0) and prints the result.print(a * b)
: This calculates the product ofa
andb
(which is 0.75) and prints the result.print(a / b)
: This performs floating-point division ofa
byb
. Since at least one operand is a float, Python performs division with floating-point precision, resulting in3.0
.
- The code assigns the floating-point value
Key Points:
- Python supports arithmetic operations on both integers (whole numbers) and floats (decimal numbers).
- The behavior of the division operator (
/
) differs for integers and floats.- For integers, it performs integer division, discarding any remainder (resulting in a float if necessary).
- For floats or mixed operand types (one integer and one float), it performs floating-point division, preserving decimal precision.
Strings
Strings are sequences of characters that are used to represent text in programming. They can be created by enclosing characters in single quotes ('') or double quotes (""). Once created, strings can be manipulated in various ways, such as by appending new characters to them or by extracting specific characters from them.
Strings can be formatted to include values that change dynamically, such as dates or user input. This allows for more dynamic and interactive programs that can respond to user input in real time. Overall, strings are a fundamental concept in programming that allow for the representation and manipulation of text-based data.
Example:
s = 'Hello, world!'
print(s)
Lists
Lists are an essential part of programming as they allow ordered collections of items. Lists are mutable, allowing you to make modifications to their content. One example of a list could be a to-do list that you might use to keep track of tasks you need to complete. You could add or remove items from this list as you complete tasks or think of new ones.
In addition to to-do lists, lists can be used for many other purposes, such as storing employee names and phone numbers, a list of your favorite books, or even a list of countries you'd like to visit someday. Lists can also be nested within each other to create more complex structures. For example, a list of to-do lists could be used to categorize your tasks by subject or priority.
Lists are a versatile and integral part of programming that can be used in a variety of ways to help organize and manage data.
Example:
# Create a list
fruits = ['apple', 'banana', 'cherry']
# Add an item to the list
fruits.append('date')
# Remove an item from the list
fruits.remove('banana')
# Access an item in the list
print(fruits[0]) # 'apple'
Code Purpose:
This code snippet demonstrates how to create a list in Python and perform common list manipulation operations, including adding, removing, and accessing elements.
Step-by-Step Breakdown:
- Creating a List:
- The line
fruits = ['apple', 'banana', 'cherry']
creates a list namedfruits
. Lists are used to store ordered collections of items in Python. In this case, the listfruits
contains three string elements: "apple", "banana", and "cherry".
- The line
- Adding an Item to the List (Append):
- The line
fruits.append('date')
adds the string element "date" to the end of thefruits
list using the.append
method. Lists are mutable, meaning their contents can be changed after creation. The.append
method is a convenient way to add new items to the end of a list.
- The line
- Removing an Item from the List (Remove):
- The line
fruits.remove('banana')
removes the first occurrence of the string element "banana" from thefruits
list using the.remove
method. It's important to note that the.remove
method removes the element based on its value, not its position in the list. If the element is not found, it will raise aValueError
.
- The line
- Accessing an Item in the List:
- The line
print(fruits[0])
prints the first element of thefruits
list. Lists are indexed starting from 0, sofruits[0]
refers to the element at index 0, which is "apple" in this case.
- The line
Key Points:
- Lists are a fundamental data structure in Python for storing collections of items.
- You can create lists using square brackets
[]
and enclosing elements separated by commas. - The
.append
method provides a way to add items to the end of a list. - The
.remove
method removes the first occurrence of a specified element from the list. - Lists are ordered, and you can access elements by their index within square brackets.
Tuples
Tuples and lists are both data structures in Python. While they share similarities, such as the ability to store multiple values in a single variable, there are also key differences. One of the main differences is that tuples are immutable, meaning that once a tuple is created, you cannot change its content. In contrast, lists are mutable, which means you can add, remove or modify elements after the list is created.
Despite their immutability, tuples are still useful in a number of scenarios. For example, they are commonly used to store related values together, such as the coordinates of a point in space. Tuples can also be used as keys in dictionaries, since they are hashable. Tuples can be used to return multiple values from a function, making them a useful tool for handling complex data.
While tuples may seem limited due to their immutability, they offer a number of advantages and use cases that make them a valuable tool in any Python programmer's toolkit.
Example:
# Create a tuple
coordinates = (10.0, 20.0)
# Access an item in the tuple
print(coordinates[0]) # 10.0
Code Purpose:
This code snippet demonstrates how to create a tuple in Python and access elements by their index.
Step-by-Step Breakdown:
- Creating a Tuple:
- The line
coordinates = (10.0, 20.0)
creates a tuple namedcoordinates
. Tuples are another fundamental data structure in Python used to store ordered collections of elements. However, unlike lists, tuples are immutable, meaning their contents cannot be changed after creation. In this case, the tuplecoordinates
contains two elements:10.0
(a float) and20.0
(a float).
- The line
- Accessing an Item in the Tuple:
- The line
print(coordinates[0])
prints the first element of thecoordinates
tuple. Similar to lists, tuples are indexed starting from 0. So,coordinates[0]
refers to the element at index 0, which is10.0
in this example.
- The line
Key Points:
- Tuples are ordered collections of elements like lists, but they are immutable (unchangeable after creation).
- Tuples are created using parentheses
()
, enclosing elements separated by commas. - You can access elements in tuples by their index within square brackets, similar to lists.
Sets
Sets are unordered collections of unique items. They are useful in various programming scenarios, such as counting unique items in a list or testing for membership. Sets can also be combined using mathematical operations such as union, intersection, and difference. In Python, sets are represented using curly braces and items are separated by commas.
One important characteristic of sets is that they do not allow duplicates. This means that if an item is added to a set that already exists, it will not change the set. Furthermore, sets are mutable, which means that items can be added or removed from the set after it has been created. Overall, sets are a versatile and important tool in programming.
Example:
# Create a set
fruits = {'apple', 'banana', 'cherry', 'apple'}
# Add an item to the set
fruits.add('date')
# Remove an item from the set
fruits.remove('banana')
# Check if an item is in the set
print('apple' in fruits) # True
Code Purpose:
This code snippet demonstrates how to create a set in Python and perform common set operations, including adding, removing, and checking for element membership.
Step-by-Step Breakdown:
- Creating a Set:
- The line
fruits = {'apple', 'banana', 'cherry', 'apple'}
creates a set namedfruits
. Sets are used to store collections of unique elements in Python. Unlike lists, sets do not allow duplicate elements. In this case, the setfruits
is created with four elements: "apple", "banana", "cherry", and "apple". However, since sets don't allow duplicates, the second occurrence of "apple" is ignored.
- The line
- Adding an Item to the Set (Add):
- The line
fruits.add('date')
adds the string element "date" to thefruits
set using the.add
method. Sets are mutable, meaning their contents can be changed after creation. The.add
method is a way to add new, unique elements to a set.
- The line
- Removing an Item from the Set (Remove):
- The line
fruits.remove('banana')
removes the element "banana" from thefruits
set using the.remove
method. Similar to lists, it removes the first occurrence of the specified element. It's important to note that.remove
will raise aKeyError
if the element is not found in the set.
- The line
- Checking for Membership:
- The line
print('apple' in fruits)
checks if the element "apple" exists in thefruits
set using thein
operator. Thein
operator returnsTrue
if the element is found in the set, andFalse
otherwise. In this case, it printsTrue
because "apple" is indeed in the set.
- The line
Key Points:
- Sets are collections of unique elements, ideal for storing unordered collections where duplicates are not allowed.
- You create sets using curly braces
{}
and enclosing elements separated by commas. - The
.add
method adds unique elements to a set. - The
.remove
method removes an element from the set, raising an error if not found. - The
in
operator checks if an element exists within a set.
Dictionaries
Dictionaries are data structures that contain key-value pairs, allowing for efficient lookups and storage of information. These pairs can represent any type of data, such as strings, numbers, or even other dictionaries. In addition to lookup and storage, dictionaries can also be used for data manipulation and analysis.
With the flexibility and versatility of dictionaries, they have become a staple in many programming languages, used in applications ranging from database management to natural language processing.
Example:
# Create a dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Access a value in the dictionary
print(person['name']) # 'Alice'
# Change a value in the dictionary
person['age'] = 26
# Add a new key-value pair to the dictionary
person['job'] = 'Engineer'
# Remove a key-value pair from the dictionary
del person['city']
# Print the modified dictionary
print(person)
Code Purpose:
This code snippet demonstrates how to create a dictionary in Python, access and modify its elements, and perform common operations like adding and removing key-value pairs.
Step-by-Step Breakdown:
- Creating a Dictionary:
- The line
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
creates a dictionary namedperson
. Dictionaries are fundamental data structures in Python for storing collections of data in a key-value format. In this case, theperson
dictionary stores three key-value pairs:- Key: 'name', Value: 'Alice' (associates the name "Alice" with the key 'name')
- Key: 'age', Value: 25 (associates the age 25 with the key 'age')
- Key: 'city', Value: 'New York' (associates the city "New York" with the key 'city')
- The line
- Accessing a Value in the Dictionary:
- The line
print(person['name'])
retrieves the value associated with the key 'name' from theperson
dictionary and prints it. Since 'name' is a key, it retrieves the corresponding value, which is "Alice" in this case.
- The line
- Changing a Value in the Dictionary:
- The line
person['age'] = 26
modifies the value associated with the key 'age' in theperson
dictionary. Dictionaries are mutable, meaning you can change their contents after creation. Here, we update the age to 26.
- The line
- Adding a New Key-Value Pair:
- The line
person['job'] = 'Engineer'
adds a new key-value pair to theperson
dictionary. The key is 'job', and the value is 'Engineer'. This extends the dictionary with new information.
- The line
- Removing a Key-Value Pair:
- The line
del person['city']
removes the key-value pair with the key 'city' from theperson
dictionary. Thedel
keyword is used for deletion.
- The line
- Printing the Modified Dictionary:
- The line
print(person)
prints the contents of the modifiedperson
dictionary. This will display the updated dictionary without the 'city' key-value pair and the changed age.
- The line
Key Points:
- Dictionaries are collections of key-value pairs, providing a flexible way to store and access data.
- You create dictionaries using curly braces
{}
with keys and values separated by colons (:
). Keys must be unique and immutable (e.g., strings, numbers). - Accessing elements is done using the key within square brackets
[]
. - You can modify existing values or add new key-value pairs using assignment.
- The
del
keyword removes key-value pairs from the dictionary.
2.1.2 Control Flow
Control flow is a crucial concept in programming. It refers to the order in which the code of a program is executed. In Python, there are several mechanisms that can be used to control the flow of a program.
One of these is the if
statement, which allows the program to make decisions based on certain conditions. Another important mechanism is the for
loop, which is used to iterate over a sequence of values or elements. Similarly, the while
loop can be used to execute a block of code repeatedly as long as a certain condition is met.
In addition to these mechanisms, Python also provides try
/except
blocks, which are used for error handling. These blocks are particularly useful when a program encounters an error or an exception that it cannot handle, as they allow the program to gracefully recover from the error and continue executing its code.
It is important for programmers to have a solid understanding of control flow and the different mechanisms that can be used to control it in Python. By using these mechanisms effectively, programmers can create programs that are both efficient and robust, and that can handle a wide range of different scenarios and situations.
If Statements
If
statements are used for conditional execution, allowing a program to make decisions based on certain conditions. This is a powerful tool that gives developers more control over the flow of their code by allowing them to specify what should happen under certain circumstances.
For example, an if
statement could be used to check if a user has entered the correct password, and if so, grant them access to a restricted area of a website. Alternatively, it could be used to check if a user has entered an invalid input, and prompt them to try again.
By using if
statements, developers can create more dynamic and versatile programs that can respond to a wider range of user inputs and conditions.
Example:
x = 10
if x > 0:
print('x is positive')
elif x < 0:
print('x is negative')
else:
print('x is zero')
For Loops
For
loops are an essential tool in programming. They are used for iterating over a sequence (like a list or a string), making it possible to perform operations on each element of the sequence. This is incredibly useful in a wide variety of contexts.
For example, imagine you have a list of items and you want to perform the same calculation on each item. A for
loop makes this task simple and efficient. By iterating over the list, the loop performs the calculation on each item in turn, saving you from having to write the same code over and over again.
In addition to lists and strings, for
loops can also be used with other types of sequences, such as dictionaries and sets, making them an incredibly versatile tool for any programmer to have in their toolkit.
Example:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
While Loops
While
loops are used for repeated execution as long as a condition is true. This means that if the condition is false from the outset, the loop will never execute. It is important to ensure that the condition will eventually become false, otherwise the loop will continue indefinitely.
In practice, while
loops can be useful for situations where you do not know how many iterations will be required. For example, if you are writing a program to calculate the square root of a number, you may not know how many iterations will be required until you get the desired level of precision.
Another use of while
loops is to repeatedly ask the user for input until they provide valid input. This can be useful to ensure that the program does not crash or behave unexpectedly due to invalid user input.
Overall, while
loops are a powerful programming construct that can be used to solve a wide variety of problems. By using them effectively, you can write code that is more efficient, easier to read, and easier to maintain.
Example:
x = 0
while x < 5:
print(x)
x += 1
2.1.3 Try/Except Blocks
Try
/except
blocks are a key part of Python programming for error handling. These blocks allow the programmer to anticipate and handle errors that may occur during the execution of a Python script. By using try/except blocks, a programmer can create a more robust and error-resistant program that can handle unexpected input or other errors without crashing. In fact, try/except blocks are so commonly used in Python programming that they are often considered a fundamental aspect of the language's syntax and functionality.
Example:
try:
x = 1 / 0 # This will raise a ZeroDivisionError
except ZeroDivisionError:
print('You cannot divide by zero!')
2.1.4 Functions
Functions are a key concept in programming that allow for the creation of reusable pieces of code. When defining a function in Python, the def
keyword is used. Functions can contain any number of statements, including loops, conditional statements, and other functions.
Additionally, functions can have parameters and return values, which allows for greater flexibility in how they are used. By breaking down a problem into smaller functions, code can be made more readable and easier to maintain.
Functions are an essential tool for any programmer looking to improve the efficiency and effectiveness of their code.
Example:
def greet(name):
return f'Hello, {name}!'
print(greet('Alice')) # 'Hello, Alice!'
2.1.5 Classes
Object-oriented programming is centered around the concept of classes. A class is essentially a blueprint that describes the properties, methods, and behaviors of objects that are created from it.
These objects can be thought of as instances of the class, each with their own unique set of values for the properties defined by the class. When you create an object from a class, you are essentially instantiating it, meaning that you are creating a new instance of the class with its own set of values.
Once an object has been created, you can then call its methods to perform various tasks. By defining these methods within the class, you can ensure that the same functionality is available to all instances of the class.
Classes provide a powerful mechanism for organizing and managing complex code, allowing you to create reusable, modular code that can be shared across multiple projects.
Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f'Hello, my name is {self.name} and I am {self.age} years old.'
alice = Person('Alice', 25)
print(alice.greet()) # 'Hello, my name is Alice and I am 25 years old.'
2.1.6 Built-in Functions and Modules
Python offers an extensive range of built-in functions and modules that provide additional functionality to the language. These modules are designed to be used in a variety of applications, from data analysis to web development and beyond.
For example, the math module provides access to mathematical functions such as trigonometry, while the os module enables interaction with the operating system. Python's standard library includes modules for working with regular expressions, file I/O, and network communication, to name just a few.
With such a wide array of tools at your disposal, Python is a powerful language that can handle a vast range of tasks and applications.
Here are some of the most commonly used ones:
Built-in Functions
Python has many built-in functions that perform a variety of tasks. Here are a few examples:
print()
: Prints the specified message to the screen.len()
: Returns the number of items in an object.type()
: Returns the type of an object.str()
,int()
,float()
: Convert an object to a string, integer, or float, respectively.input()
: Reads a line from input (keyboard), converts it to a string, and returns it.
Here's how you can use these functions:
print(len('Hello, world!')) # 13
print(type(10)) # <class 'int'>
print(int('10')) # 10
name = input('What is your name? ')
Built-in Modules
Python also comes with a set of built-in modules that you can import into your program to use. Here are a few examples:
math
: Provides mathematical functions.random
: Provides functions for generating random numbers.datetime
: Provides functions for manipulating dates and times.os
: Provides functions for interacting with the operating system.
Here's how you can use these modules:
import math
print(math.sqrt(16)) # 4.0
import random
print(random.randint(1, 10)) # a random integer between 1 and 10
import datetime
print(datetime.datetime.now()) # current date and time
import os
print(os.getcwd()) # current working directory
This concludes our Python crash course. While this section only scratches the surface of what Python can do, it should give you a good foundation to build upon. In the next sections, we will explore some of the key Python libraries used in Machine Learning.
If you want to gain a better and deeper understanding of Python basics, this book may be of interest to you:
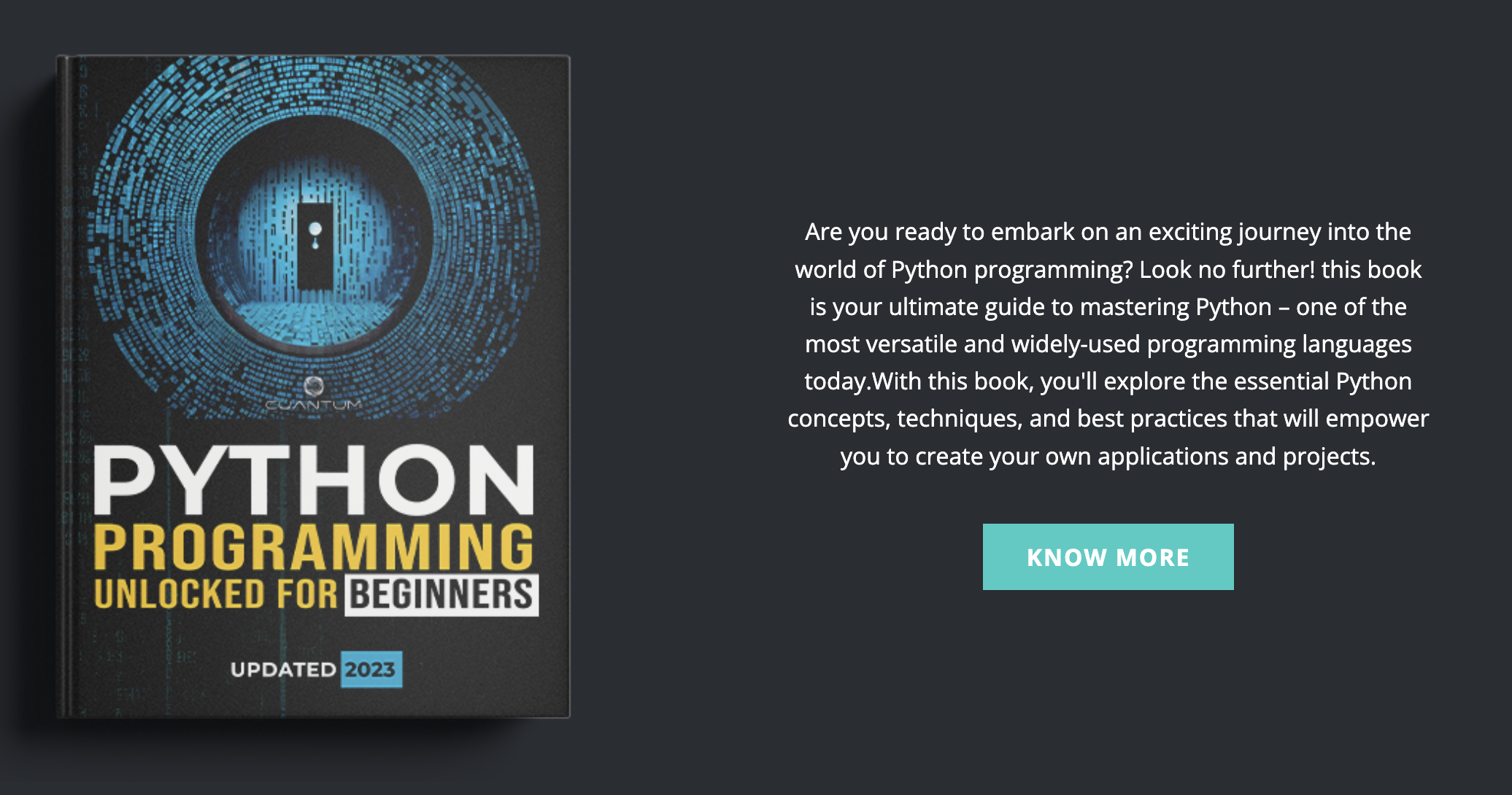