Chapter 2: Python and Essential Libraries
2.3 Pandas for Data Manipulation
Pandas is a powerful data manipulation library in Python. It provides data structures and functions needed to manipulate and analyze structured data. The name Pandas is derived from the term "Panel Data", an econometrics term for datasets that include observations over multiple time periods for the same individuals.
Pandas is built on top of NumPy and provides two key data structures: Series and DataFrame. A Series is a one-dimensional array-like object that can hold any data type, and a DataFrame is a two-dimensional table of data with rows and columns.
In this section, we will cover the basics of Pandas, including creating DataFrames, data selection, data cleaning, and basic data analysis.
2.3.1 Installation
Before we start, make sure you have Pandas installed. If you haven't installed it yet, you can do so using pip:
pip install pandas
2.3.2 Importing Pandas
To use Pandas in your Python program, you first need to import it. It's common to import Pandas with the alias pd
:
import pandas as pd
2.3.3 Creating DataFrames
There are several ways to create a DataFrame in Pandas. One way is to use a dictionary, where the keys represent the column names and the values represent the data. Another way is to use a list of dictionaries, where each dictionary represents a row of data.
Finally, you can also create a DataFrame from a 2D NumPy array, where each row represents an observation and each column represents a variable. In this case, you can specify the column names using the columns
parameter.
As you can see, pandas provides several options for creating a DataFrame, which makes it a versatile tool for data analysis and manipulation.
Example:
import pandas as pd
import numpy as np
# From a dictionary
df = pd.DataFrame({
'A': [1, 2, 3],
'B': ['a', 'b', 'c'],
})
print(df)
# From a list of dictionaries
df = pd.DataFrame([
{'A': 1, 'B': 'a'},
{'A': 2, 'B': 'b'},
{'A': 3, 'B': 'c'},
])
print(df)
# From a 2D NumPy array
array = np.array([[1, 'a'], [2, 'b'], [3, 'c']])
df = pd.DataFrame(array, columns=['A', 'B'])
print(df)
2.3.4 Data Selection
When working with a DataFrame, there are several ways to select data. The most common methods include selecting data using column names, row labels, or row numbers. In addition, you can also filter data by specifying conditions using boolean indexing.
It is important to keep in mind that the method you choose will depend on the specific task at hand and the structure of your data. Furthermore, it is often helpful to combine multiple selection methods to efficiently extract the data you need.
Example:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': ['a', 'b', 'c'],
})
# Select a column
print(df['A'])
# Select multiple columns
print(df[['A', 'B']])
# Select a row by label
print(df.loc[0])
# Select a row by number
print(df.iloc[0])
# Select a specific value
print(df.loc[0, 'A'])
print(df.iloc[0, 0])
2.3.5 Data Cleaning
Pandas is a powerful tool for working with data. One of the many benefits of using Pandas is that it provides a wide range of functions that can help you clean your data quickly and easily. For example, if your data has missing values, you can use Pandas to fill those missing values with a variety of options, such as the mean, median, or mode of the data. In addition, if you need to replace certain values in your data, Pandas makes it easy to do so by allowing you to specify what values you want to replace and what you want to replace them with. These are just a few examples of the many ways that Pandas can help you clean your data and make it more useful for your analysis.
Pandas provides many functions for cleaning data, such as filling missing values and replacing values.
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': [1, 2, np.nan],
'B': ['a', 'b', 'c'],
})
# Fill missing values
df_filled = df.fillna(0)
print(df_filled)
# Replace values
df_replaced = df.replace(np.nan, 0)
print(df_replaced)
2.3.6 Basic Data Analysis
Pandas provides many functions for basic data analysis, such as calculating the mean, sum, max, min, and more:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
})
# Calculate the mean of a column
print(df['A'].mean())
# Calculate the sum of a column
print(df['A'].sum())
# Calculate the maximum value of a column
print(df['A'].max())
# Calculate the minimum value of a column
print(df['A'].min())
Pandas also provides the describe
function, which computes a variety of summary statistics about a column:
print(df['A'].describe())
2.3. Advanced Pandas Features
Grouping Data
Pandas is a powerful library for data manipulation in Python. One of its most useful functions is the groupby
function, which makes it easy to split a DataFrame into groups based on some criteria. For example, you can group a DataFrame by the values in a particular column, or by the result of a custom function that you define.
Once you have created these groups, you can apply a function to each group independently. This is incredibly useful for performing calculations or transformations on subsets of your data. For example, you could calculate summary statistics for each group, such as the mean or median value of a particular column.
After applying the function to each group, you can then combine the results back into a new DataFrame. This allows you to easily compare the results of your calculations across different groups, and to identify any patterns or trends that may be present.
Overall, the groupby
function in Pandas is a powerful tool for data analysis and exploration. Whether you are working with small or large datasets, it can help you to quickly and easily extract insights from your data, and to make more informed decisions based on those insights.
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'three', 'two', 'two', 'one', 'three'],
'C': np.random.randn(8),
'D': np.random.randn(8)
})
# Group by column 'A' and calculate the sum of 'C' and 'D' for each group
grouped = df.groupby('A').sum()
print(grouped)
Merging Data
Pandas is a powerful tool for data analysis that allows users to manipulate and analyze data in various ways. One of the most useful features of Pandas is its ability to combine and merge DataFrames.
In addition to the merge
, join
, and concatenate
functions, Pandas also provides various other methods for combining and manipulating data. For example, users can perform operations such as filtering, grouping, and sorting data to gain insights and make informed decisions.
Pandas supports a wide range of data formats, making it easy to work with data from different sources. With its versatility and robust set of features, Pandas is an essential tool for anyone working with data in Python.
Example:
import pandas as pd
df1 = pd.DataFrame({
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'key': ['K0', 'K1', 'K0', 'K1']
})
df2 = pd.DataFrame({
'C': ['C0', 'C1'],
'D': ['D0', 'D1']},
index=['K0', 'K1']
)
# Merge df1 and df2 on the 'key' column
merged = pd.merge(df1, df2, left_on='key', right_index=True)
print(merged)
This concludes our introduction to Pandas. While this section only scratches the surface of what Pandas can do, it should give you a good foundation to build upon. In the next sections, we will explore other key Python libraries used in Machine Learning.
If you want gain more deep understanding of Pandas we recommend our following book:
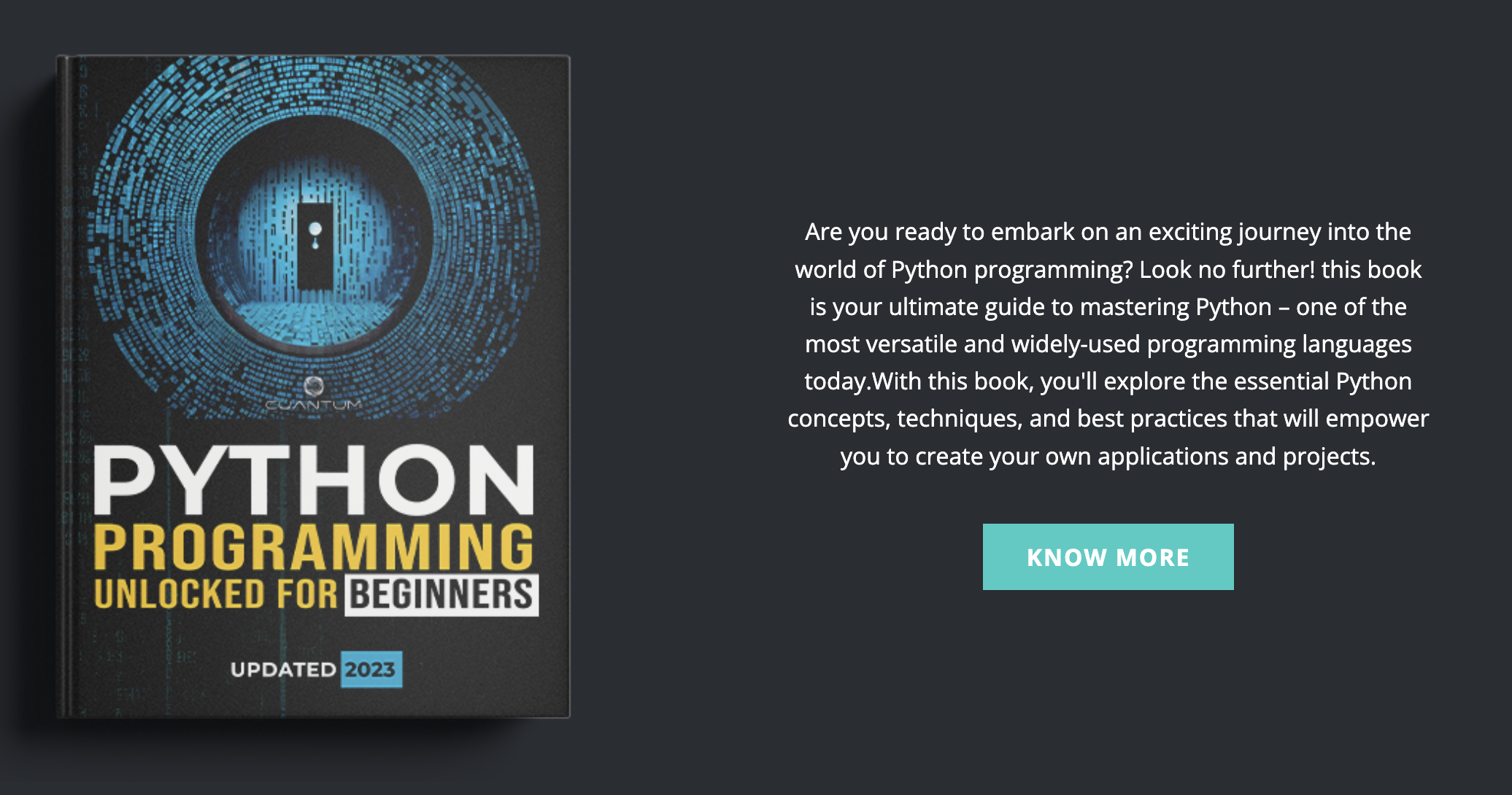
2.3 Pandas for Data Manipulation
Pandas is a powerful data manipulation library in Python. It provides data structures and functions needed to manipulate and analyze structured data. The name Pandas is derived from the term "Panel Data", an econometrics term for datasets that include observations over multiple time periods for the same individuals.
Pandas is built on top of NumPy and provides two key data structures: Series and DataFrame. A Series is a one-dimensional array-like object that can hold any data type, and a DataFrame is a two-dimensional table of data with rows and columns.
In this section, we will cover the basics of Pandas, including creating DataFrames, data selection, data cleaning, and basic data analysis.
2.3.1 Installation
Before we start, make sure you have Pandas installed. If you haven't installed it yet, you can do so using pip:
pip install pandas
2.3.2 Importing Pandas
To use Pandas in your Python program, you first need to import it. It's common to import Pandas with the alias pd
:
import pandas as pd
2.3.3 Creating DataFrames
There are several ways to create a DataFrame in Pandas. One way is to use a dictionary, where the keys represent the column names and the values represent the data. Another way is to use a list of dictionaries, where each dictionary represents a row of data.
Finally, you can also create a DataFrame from a 2D NumPy array, where each row represents an observation and each column represents a variable. In this case, you can specify the column names using the columns
parameter.
As you can see, pandas provides several options for creating a DataFrame, which makes it a versatile tool for data analysis and manipulation.
Example:
import pandas as pd
import numpy as np
# From a dictionary
df = pd.DataFrame({
'A': [1, 2, 3],
'B': ['a', 'b', 'c'],
})
print(df)
# From a list of dictionaries
df = pd.DataFrame([
{'A': 1, 'B': 'a'},
{'A': 2, 'B': 'b'},
{'A': 3, 'B': 'c'},
])
print(df)
# From a 2D NumPy array
array = np.array([[1, 'a'], [2, 'b'], [3, 'c']])
df = pd.DataFrame(array, columns=['A', 'B'])
print(df)
2.3.4 Data Selection
When working with a DataFrame, there are several ways to select data. The most common methods include selecting data using column names, row labels, or row numbers. In addition, you can also filter data by specifying conditions using boolean indexing.
It is important to keep in mind that the method you choose will depend on the specific task at hand and the structure of your data. Furthermore, it is often helpful to combine multiple selection methods to efficiently extract the data you need.
Example:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': ['a', 'b', 'c'],
})
# Select a column
print(df['A'])
# Select multiple columns
print(df[['A', 'B']])
# Select a row by label
print(df.loc[0])
# Select a row by number
print(df.iloc[0])
# Select a specific value
print(df.loc[0, 'A'])
print(df.iloc[0, 0])
2.3.5 Data Cleaning
Pandas is a powerful tool for working with data. One of the many benefits of using Pandas is that it provides a wide range of functions that can help you clean your data quickly and easily. For example, if your data has missing values, you can use Pandas to fill those missing values with a variety of options, such as the mean, median, or mode of the data. In addition, if you need to replace certain values in your data, Pandas makes it easy to do so by allowing you to specify what values you want to replace and what you want to replace them with. These are just a few examples of the many ways that Pandas can help you clean your data and make it more useful for your analysis.
Pandas provides many functions for cleaning data, such as filling missing values and replacing values.
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': [1, 2, np.nan],
'B': ['a', 'b', 'c'],
})
# Fill missing values
df_filled = df.fillna(0)
print(df_filled)
# Replace values
df_replaced = df.replace(np.nan, 0)
print(df_replaced)
2.3.6 Basic Data Analysis
Pandas provides many functions for basic data analysis, such as calculating the mean, sum, max, min, and more:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
})
# Calculate the mean of a column
print(df['A'].mean())
# Calculate the sum of a column
print(df['A'].sum())
# Calculate the maximum value of a column
print(df['A'].max())
# Calculate the minimum value of a column
print(df['A'].min())
Pandas also provides the describe
function, which computes a variety of summary statistics about a column:
print(df['A'].describe())
2.3. Advanced Pandas Features
Grouping Data
Pandas is a powerful library for data manipulation in Python. One of its most useful functions is the groupby
function, which makes it easy to split a DataFrame into groups based on some criteria. For example, you can group a DataFrame by the values in a particular column, or by the result of a custom function that you define.
Once you have created these groups, you can apply a function to each group independently. This is incredibly useful for performing calculations or transformations on subsets of your data. For example, you could calculate summary statistics for each group, such as the mean or median value of a particular column.
After applying the function to each group, you can then combine the results back into a new DataFrame. This allows you to easily compare the results of your calculations across different groups, and to identify any patterns or trends that may be present.
Overall, the groupby
function in Pandas is a powerful tool for data analysis and exploration. Whether you are working with small or large datasets, it can help you to quickly and easily extract insights from your data, and to make more informed decisions based on those insights.
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'three', 'two', 'two', 'one', 'three'],
'C': np.random.randn(8),
'D': np.random.randn(8)
})
# Group by column 'A' and calculate the sum of 'C' and 'D' for each group
grouped = df.groupby('A').sum()
print(grouped)
Merging Data
Pandas is a powerful tool for data analysis that allows users to manipulate and analyze data in various ways. One of the most useful features of Pandas is its ability to combine and merge DataFrames.
In addition to the merge
, join
, and concatenate
functions, Pandas also provides various other methods for combining and manipulating data. For example, users can perform operations such as filtering, grouping, and sorting data to gain insights and make informed decisions.
Pandas supports a wide range of data formats, making it easy to work with data from different sources. With its versatility and robust set of features, Pandas is an essential tool for anyone working with data in Python.
Example:
import pandas as pd
df1 = pd.DataFrame({
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'key': ['K0', 'K1', 'K0', 'K1']
})
df2 = pd.DataFrame({
'C': ['C0', 'C1'],
'D': ['D0', 'D1']},
index=['K0', 'K1']
)
# Merge df1 and df2 on the 'key' column
merged = pd.merge(df1, df2, left_on='key', right_index=True)
print(merged)
This concludes our introduction to Pandas. While this section only scratches the surface of what Pandas can do, it should give you a good foundation to build upon. In the next sections, we will explore other key Python libraries used in Machine Learning.
If you want gain more deep understanding of Pandas we recommend our following book:
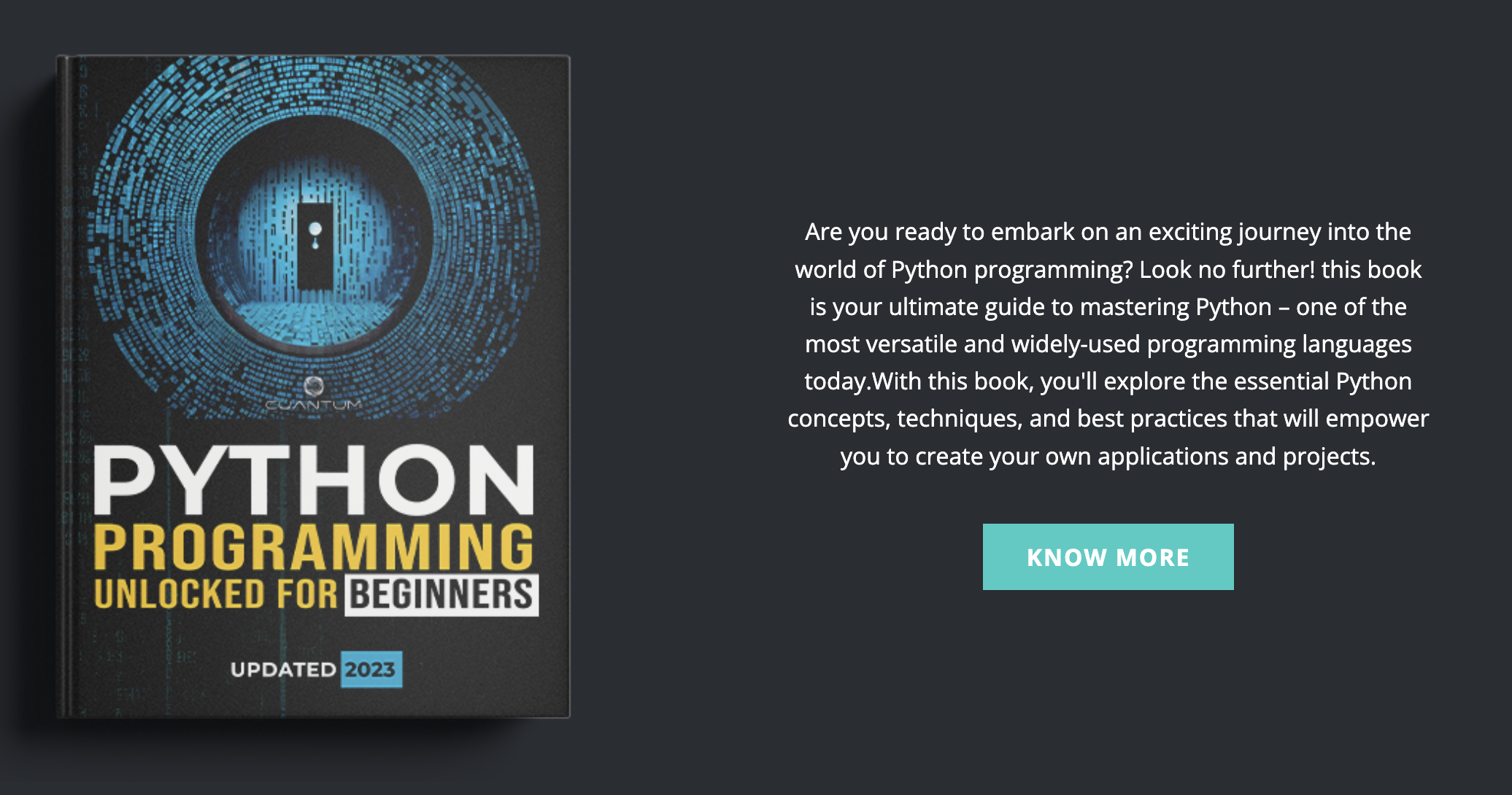
2.3 Pandas for Data Manipulation
Pandas is a powerful data manipulation library in Python. It provides data structures and functions needed to manipulate and analyze structured data. The name Pandas is derived from the term "Panel Data", an econometrics term for datasets that include observations over multiple time periods for the same individuals.
Pandas is built on top of NumPy and provides two key data structures: Series and DataFrame. A Series is a one-dimensional array-like object that can hold any data type, and a DataFrame is a two-dimensional table of data with rows and columns.
In this section, we will cover the basics of Pandas, including creating DataFrames, data selection, data cleaning, and basic data analysis.
2.3.1 Installation
Before we start, make sure you have Pandas installed. If you haven't installed it yet, you can do so using pip:
pip install pandas
2.3.2 Importing Pandas
To use Pandas in your Python program, you first need to import it. It's common to import Pandas with the alias pd
:
import pandas as pd
2.3.3 Creating DataFrames
There are several ways to create a DataFrame in Pandas. One way is to use a dictionary, where the keys represent the column names and the values represent the data. Another way is to use a list of dictionaries, where each dictionary represents a row of data.
Finally, you can also create a DataFrame from a 2D NumPy array, where each row represents an observation and each column represents a variable. In this case, you can specify the column names using the columns
parameter.
As you can see, pandas provides several options for creating a DataFrame, which makes it a versatile tool for data analysis and manipulation.
Example:
import pandas as pd
import numpy as np
# From a dictionary
df = pd.DataFrame({
'A': [1, 2, 3],
'B': ['a', 'b', 'c'],
})
print(df)
# From a list of dictionaries
df = pd.DataFrame([
{'A': 1, 'B': 'a'},
{'A': 2, 'B': 'b'},
{'A': 3, 'B': 'c'},
])
print(df)
# From a 2D NumPy array
array = np.array([[1, 'a'], [2, 'b'], [3, 'c']])
df = pd.DataFrame(array, columns=['A', 'B'])
print(df)
2.3.4 Data Selection
When working with a DataFrame, there are several ways to select data. The most common methods include selecting data using column names, row labels, or row numbers. In addition, you can also filter data by specifying conditions using boolean indexing.
It is important to keep in mind that the method you choose will depend on the specific task at hand and the structure of your data. Furthermore, it is often helpful to combine multiple selection methods to efficiently extract the data you need.
Example:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': ['a', 'b', 'c'],
})
# Select a column
print(df['A'])
# Select multiple columns
print(df[['A', 'B']])
# Select a row by label
print(df.loc[0])
# Select a row by number
print(df.iloc[0])
# Select a specific value
print(df.loc[0, 'A'])
print(df.iloc[0, 0])
2.3.5 Data Cleaning
Pandas is a powerful tool for working with data. One of the many benefits of using Pandas is that it provides a wide range of functions that can help you clean your data quickly and easily. For example, if your data has missing values, you can use Pandas to fill those missing values with a variety of options, such as the mean, median, or mode of the data. In addition, if you need to replace certain values in your data, Pandas makes it easy to do so by allowing you to specify what values you want to replace and what you want to replace them with. These are just a few examples of the many ways that Pandas can help you clean your data and make it more useful for your analysis.
Pandas provides many functions for cleaning data, such as filling missing values and replacing values.
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': [1, 2, np.nan],
'B': ['a', 'b', 'c'],
})
# Fill missing values
df_filled = df.fillna(0)
print(df_filled)
# Replace values
df_replaced = df.replace(np.nan, 0)
print(df_replaced)
2.3.6 Basic Data Analysis
Pandas provides many functions for basic data analysis, such as calculating the mean, sum, max, min, and more:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
})
# Calculate the mean of a column
print(df['A'].mean())
# Calculate the sum of a column
print(df['A'].sum())
# Calculate the maximum value of a column
print(df['A'].max())
# Calculate the minimum value of a column
print(df['A'].min())
Pandas also provides the describe
function, which computes a variety of summary statistics about a column:
print(df['A'].describe())
2.3. Advanced Pandas Features
Grouping Data
Pandas is a powerful library for data manipulation in Python. One of its most useful functions is the groupby
function, which makes it easy to split a DataFrame into groups based on some criteria. For example, you can group a DataFrame by the values in a particular column, or by the result of a custom function that you define.
Once you have created these groups, you can apply a function to each group independently. This is incredibly useful for performing calculations or transformations on subsets of your data. For example, you could calculate summary statistics for each group, such as the mean or median value of a particular column.
After applying the function to each group, you can then combine the results back into a new DataFrame. This allows you to easily compare the results of your calculations across different groups, and to identify any patterns or trends that may be present.
Overall, the groupby
function in Pandas is a powerful tool for data analysis and exploration. Whether you are working with small or large datasets, it can help you to quickly and easily extract insights from your data, and to make more informed decisions based on those insights.
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'three', 'two', 'two', 'one', 'three'],
'C': np.random.randn(8),
'D': np.random.randn(8)
})
# Group by column 'A' and calculate the sum of 'C' and 'D' for each group
grouped = df.groupby('A').sum()
print(grouped)
Merging Data
Pandas is a powerful tool for data analysis that allows users to manipulate and analyze data in various ways. One of the most useful features of Pandas is its ability to combine and merge DataFrames.
In addition to the merge
, join
, and concatenate
functions, Pandas also provides various other methods for combining and manipulating data. For example, users can perform operations such as filtering, grouping, and sorting data to gain insights and make informed decisions.
Pandas supports a wide range of data formats, making it easy to work with data from different sources. With its versatility and robust set of features, Pandas is an essential tool for anyone working with data in Python.
Example:
import pandas as pd
df1 = pd.DataFrame({
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'key': ['K0', 'K1', 'K0', 'K1']
})
df2 = pd.DataFrame({
'C': ['C0', 'C1'],
'D': ['D0', 'D1']},
index=['K0', 'K1']
)
# Merge df1 and df2 on the 'key' column
merged = pd.merge(df1, df2, left_on='key', right_index=True)
print(merged)
This concludes our introduction to Pandas. While this section only scratches the surface of what Pandas can do, it should give you a good foundation to build upon. In the next sections, we will explore other key Python libraries used in Machine Learning.
If you want gain more deep understanding of Pandas we recommend our following book:
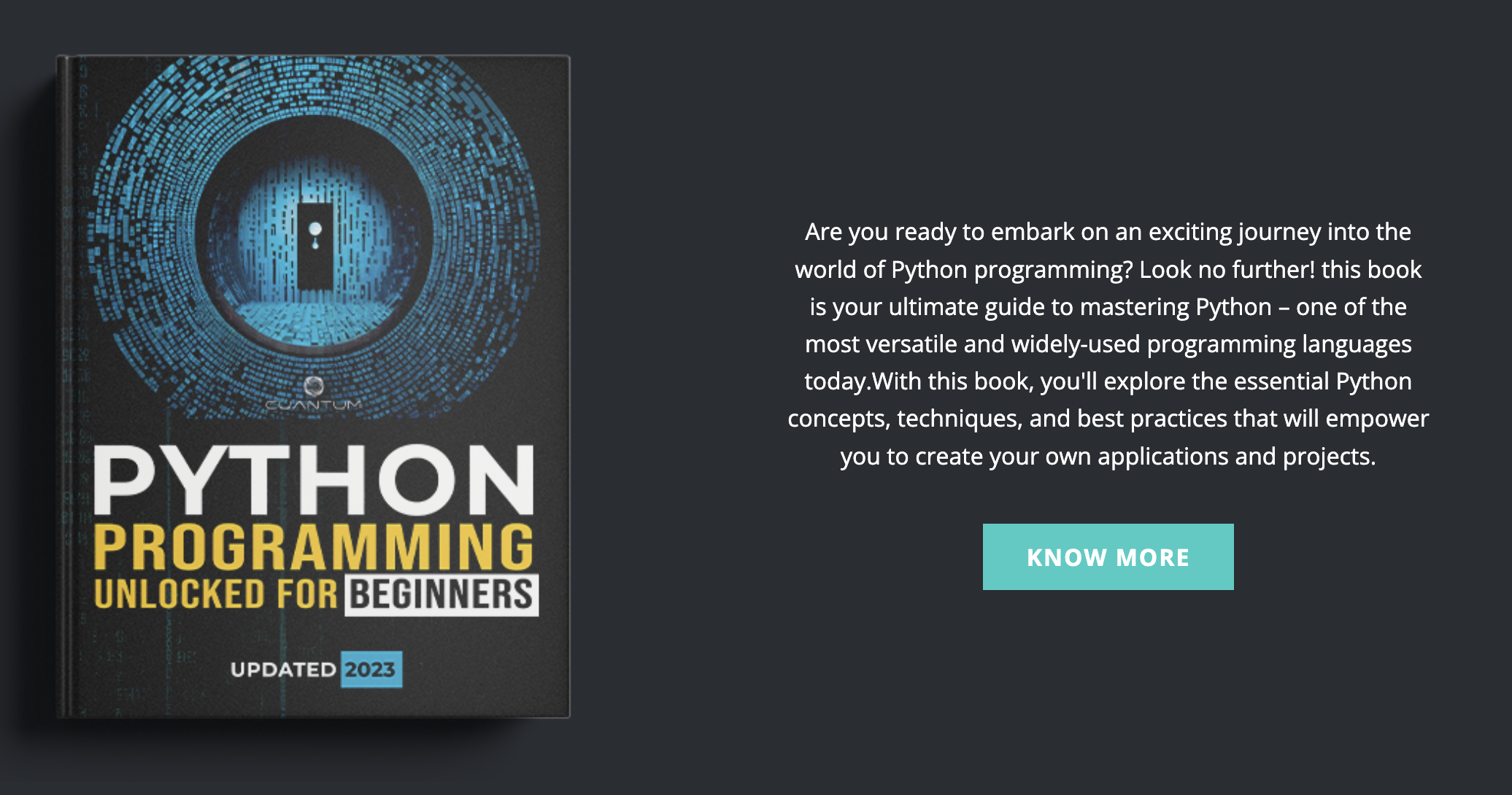
2.3 Pandas for Data Manipulation
Pandas is a powerful data manipulation library in Python. It provides data structures and functions needed to manipulate and analyze structured data. The name Pandas is derived from the term "Panel Data", an econometrics term for datasets that include observations over multiple time periods for the same individuals.
Pandas is built on top of NumPy and provides two key data structures: Series and DataFrame. A Series is a one-dimensional array-like object that can hold any data type, and a DataFrame is a two-dimensional table of data with rows and columns.
In this section, we will cover the basics of Pandas, including creating DataFrames, data selection, data cleaning, and basic data analysis.
2.3.1 Installation
Before we start, make sure you have Pandas installed. If you haven't installed it yet, you can do so using pip:
pip install pandas
2.3.2 Importing Pandas
To use Pandas in your Python program, you first need to import it. It's common to import Pandas with the alias pd
:
import pandas as pd
2.3.3 Creating DataFrames
There are several ways to create a DataFrame in Pandas. One way is to use a dictionary, where the keys represent the column names and the values represent the data. Another way is to use a list of dictionaries, where each dictionary represents a row of data.
Finally, you can also create a DataFrame from a 2D NumPy array, where each row represents an observation and each column represents a variable. In this case, you can specify the column names using the columns
parameter.
As you can see, pandas provides several options for creating a DataFrame, which makes it a versatile tool for data analysis and manipulation.
Example:
import pandas as pd
import numpy as np
# From a dictionary
df = pd.DataFrame({
'A': [1, 2, 3],
'B': ['a', 'b', 'c'],
})
print(df)
# From a list of dictionaries
df = pd.DataFrame([
{'A': 1, 'B': 'a'},
{'A': 2, 'B': 'b'},
{'A': 3, 'B': 'c'},
])
print(df)
# From a 2D NumPy array
array = np.array([[1, 'a'], [2, 'b'], [3, 'c']])
df = pd.DataFrame(array, columns=['A', 'B'])
print(df)
2.3.4 Data Selection
When working with a DataFrame, there are several ways to select data. The most common methods include selecting data using column names, row labels, or row numbers. In addition, you can also filter data by specifying conditions using boolean indexing.
It is important to keep in mind that the method you choose will depend on the specific task at hand and the structure of your data. Furthermore, it is often helpful to combine multiple selection methods to efficiently extract the data you need.
Example:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': ['a', 'b', 'c'],
})
# Select a column
print(df['A'])
# Select multiple columns
print(df[['A', 'B']])
# Select a row by label
print(df.loc[0])
# Select a row by number
print(df.iloc[0])
# Select a specific value
print(df.loc[0, 'A'])
print(df.iloc[0, 0])
2.3.5 Data Cleaning
Pandas is a powerful tool for working with data. One of the many benefits of using Pandas is that it provides a wide range of functions that can help you clean your data quickly and easily. For example, if your data has missing values, you can use Pandas to fill those missing values with a variety of options, such as the mean, median, or mode of the data. In addition, if you need to replace certain values in your data, Pandas makes it easy to do so by allowing you to specify what values you want to replace and what you want to replace them with. These are just a few examples of the many ways that Pandas can help you clean your data and make it more useful for your analysis.
Pandas provides many functions for cleaning data, such as filling missing values and replacing values.
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': [1, 2, np.nan],
'B': ['a', 'b', 'c'],
})
# Fill missing values
df_filled = df.fillna(0)
print(df_filled)
# Replace values
df_replaced = df.replace(np.nan, 0)
print(df_replaced)
2.3.6 Basic Data Analysis
Pandas provides many functions for basic data analysis, such as calculating the mean, sum, max, min, and more:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
})
# Calculate the mean of a column
print(df['A'].mean())
# Calculate the sum of a column
print(df['A'].sum())
# Calculate the maximum value of a column
print(df['A'].max())
# Calculate the minimum value of a column
print(df['A'].min())
Pandas also provides the describe
function, which computes a variety of summary statistics about a column:
print(df['A'].describe())
2.3. Advanced Pandas Features
Grouping Data
Pandas is a powerful library for data manipulation in Python. One of its most useful functions is the groupby
function, which makes it easy to split a DataFrame into groups based on some criteria. For example, you can group a DataFrame by the values in a particular column, or by the result of a custom function that you define.
Once you have created these groups, you can apply a function to each group independently. This is incredibly useful for performing calculations or transformations on subsets of your data. For example, you could calculate summary statistics for each group, such as the mean or median value of a particular column.
After applying the function to each group, you can then combine the results back into a new DataFrame. This allows you to easily compare the results of your calculations across different groups, and to identify any patterns or trends that may be present.
Overall, the groupby
function in Pandas is a powerful tool for data analysis and exploration. Whether you are working with small or large datasets, it can help you to quickly and easily extract insights from your data, and to make more informed decisions based on those insights.
Example:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'three', 'two', 'two', 'one', 'three'],
'C': np.random.randn(8),
'D': np.random.randn(8)
})
# Group by column 'A' and calculate the sum of 'C' and 'D' for each group
grouped = df.groupby('A').sum()
print(grouped)
Merging Data
Pandas is a powerful tool for data analysis that allows users to manipulate and analyze data in various ways. One of the most useful features of Pandas is its ability to combine and merge DataFrames.
In addition to the merge
, join
, and concatenate
functions, Pandas also provides various other methods for combining and manipulating data. For example, users can perform operations such as filtering, grouping, and sorting data to gain insights and make informed decisions.
Pandas supports a wide range of data formats, making it easy to work with data from different sources. With its versatility and robust set of features, Pandas is an essential tool for anyone working with data in Python.
Example:
import pandas as pd
df1 = pd.DataFrame({
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'key': ['K0', 'K1', 'K0', 'K1']
})
df2 = pd.DataFrame({
'C': ['C0', 'C1'],
'D': ['D0', 'D1']},
index=['K0', 'K1']
)
# Merge df1 and df2 on the 'key' column
merged = pd.merge(df1, df2, left_on='key', right_index=True)
print(merged)
This concludes our introduction to Pandas. While this section only scratches the surface of what Pandas can do, it should give you a good foundation to build upon. In the next sections, we will explore other key Python libraries used in Machine Learning.
If you want gain more deep understanding of Pandas we recommend our following book:
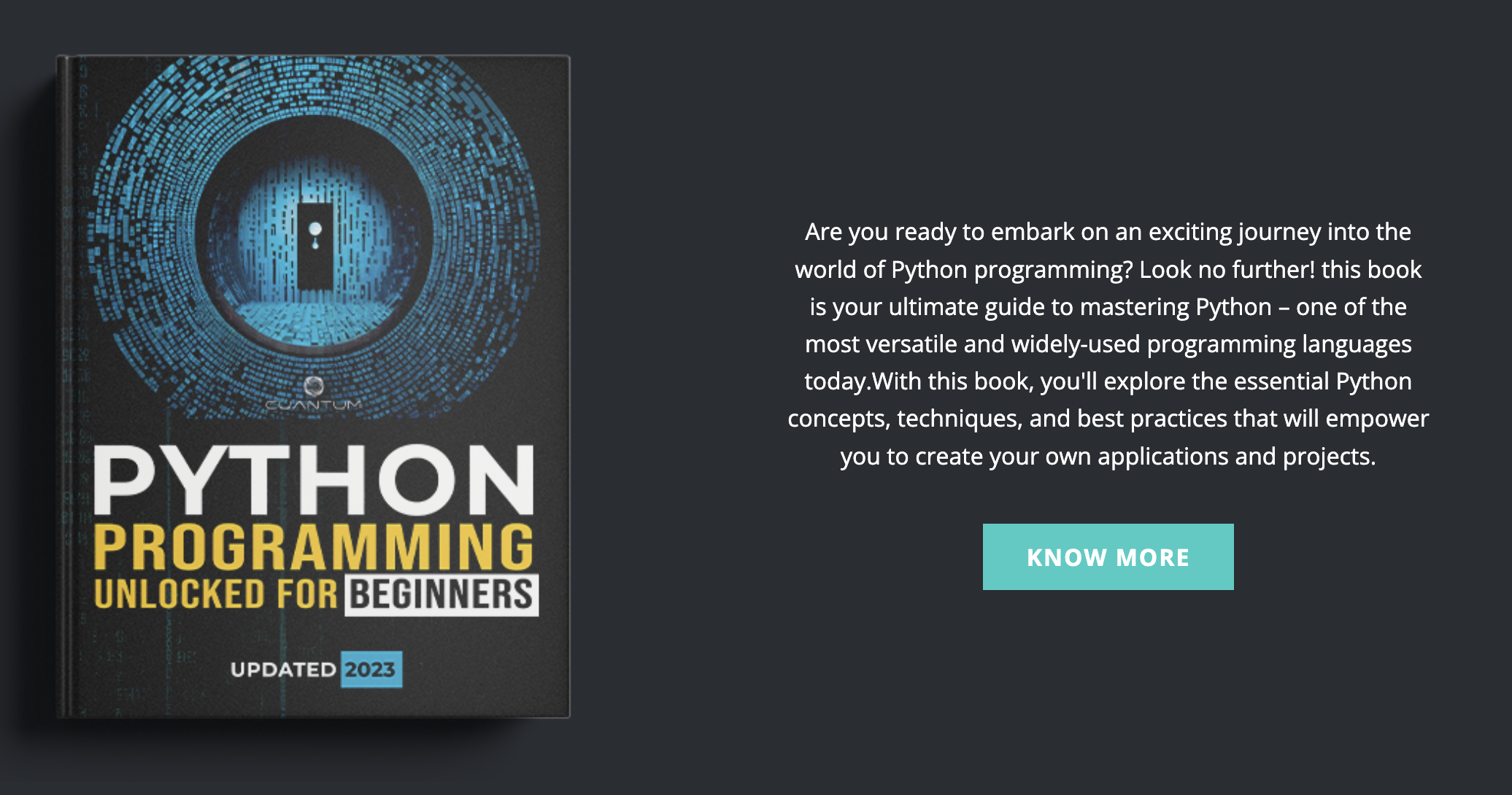