Chapter 3 - Basic Usage of ChatGPT API
3.2. Controlling the Output
To achieve the best possible results with ChatGPT, it's important to have fine-grained control over the generated output. This allows you to not only adjust the response length, creativity, and sampling strategies, but also to tailor the generated text to your specific needs. By using a combination of various techniques and parameters, you can achieve a wide range of outputs, from short and to-the-point responses to more verbose, elaborated ones that provide more context and detail.
One of the key aspects to consider is the response length. By default, ChatGPT generates responses that are between one and three sentences long, which can be useful for quick, simple interactions. However, in certain contexts, you might need longer responses that provide more information and context. In these cases, you can adjust the length of the generated text by specifying a minimum and maximum length for the output.
Another important parameter to consider is creativity. By default, ChatGPT generates responses that are relatively conservative and safe, in order to avoid generating inappropriate or offensive content. However, in some cases, you might want to increase the creativity of the generated text, to produce more surprising or unexpected outputs. This can be done by adjusting the temperature parameter, which controls the randomness and diversity of the generated text.
Sampling strategies can also play an important role in determining the quality and relevance of the generated text. ChatGPT supports several sampling strategies, including top-k sampling, nucleus sampling, and beam search, each with its own advantages and drawbacks. By experimenting with different sampling techniques, you can find the one that best suits your needs and preferences, and generates the most accurate and relevant responses.
3.2.1. Adjusting Response Length and Creativity
ChatGPT's response length and creativity can be controlled by modifying the max_tokens
, temperature
, and top_p
parameters.
max_tokens
: This parameter sets the maximum number of tokens in the generated response. By increasing or decreasing this value, you can control the length of the output. The max_tokens
parameter determines the maximum number of tokens that the model can generate in its response. A higher value will result in longer responses, while a lower value will result in shorter responses.
temperature
: This parameter influences the randomness of the generated text. A higher temperature value (e.g., 1.0) will make the output more creative and diverse, while a lower value (e.g., 0.1) will make it more focused and deterministic. The temperature
parameter controls the "creativity" of the model. A higher value will result in more surprising and diverse responses, while a lower value will result in more predictable responses.
The top_p
parameter is used to control the diversity of the generated responses by restricting the probability mass to the top p
tokens. A lower value of p
will result in more conservative responses, while a higher value of p
will result in more varied responses.
Here's an explanation of each parameter and an example of how to use them:
Example:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="Write a short introduction to artificial intelligence.",
max_tokens=100, # Adjust the max_tokens value to control response length
n=1,
stop=None,
temperature=0.5, # Adjust the temperature value to control creativity
)
3.2.2. Temperature and Top-k Sampling
There are two primary sampling strategies for controlling the randomness of ChatGPT's output: temperature sampling and top-k sampling.
temperature
: As mentioned earlier, this parameter influences the randomness of the generated text. A higher temperature value will produce more diverse and creative output, while a lower value will generate more focused and deterministic text.
top_k
: This parameter controls the top-k sampling strategy that is used during the text generation process. As you may know, the model selects the next token from the top-k most likely tokens. However, by adjusting the value of top_k
, you can control the diversity of the generated output. In other words, a lower top_k
value will result in a more conservative and predictable output, while a higher top_k
value will result in a more diverse and surprising output.
Therefore, it is important to experiment with different values of top_k
to find the one that best suits your needs. Additionally, keep in mind that other parameters, such as temperature
and length_penalty
, may also affect the quality and diversity of the generated output, so it is important to consider them as well when fine-tuning your model.
Here's an example of using both the temperature
and top_k
parameters:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="Describe the process of photosynthesis.",
max_tokens=150,
n=1,
stop=None,
temperature=0.7, # Adjust the temperature value
top_k=50, # Add the top_k parameter for top-k sampling
)
3.2.3. Using the stop
Parameter
The stop
parameter is a useful feature that enables you to specify a list of tokens at which the API should stop generating text. This can be especially helpful when you want to customize the output structure and ensure that the response ends at a logical point. By using the stop
parameter, you can create more complex and nuanced outputs that better reflect the context and purpose of your text.
Furthermore, the stop
parameter allows you to refine your text generation process by providing more detailed control over the content and structure of your responses. With this powerful tool at your disposal, you can create more engaging, informative, and compelling content that resonates with your audience and achieves your goals.
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="List the benefits of exercise:",
max_tokens=50,
n=1,
stop=["\n"], # Stop generating text at the first newline character
temperature=0.5,
)
3.2.4. Generating Multiple Responses with n
The n
parameter is an extremely useful tool that enables you to generate multiple responses for a single prompt. This is particularly helpful when you want to explore different ideas or provide users with a wide variety of options.
With the n
parameter, you can easily fine-tune the output to generate exactly the kind of content you need. Whether you're looking to brainstorm new ideas or provide users with a range of choices, the n
parameter is an essential tool in your arsenal.
By leveraging this powerful feature, you'll be able to take your content creation to the next level and achieve remarkable results with your audience.
To generate multiple responses, simply set the n
parameter to the desired number of responses:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="What are some creative ways to reuse plastic bottles?",
max_tokens=100,
n=3, # Generate 3 different responses
stop=None,
temperature=0.7,
)
for i, choice in enumerate(response.choices):
print(f"Response {i + 1}:")
print(choice.text.strip())
print()
3.2.5. Prompt Engineering
Prompt engineering involves crafting the input text in such a way that it encourages the model to generate the desired output. One way to do this is by asking the model to think step-by-step. For example, you can break down a complex question into smaller sub-questions, and ask the model to answer each one in sequence.
Alternatively, you could ask the model to debate pros and cons, where it is required to weigh the advantages and disadvantages of a given topic. You could also ask the model to provide a summary before giving a detailed answer, which can help it to focus on the key points and avoid meandering. By using these techniques, you can obtain more focused and relevant responses from ChatGPT, which can be useful in a variety of settings, such as customer service, research, or entertainment.
Example:
import openai
openai.api_key = "your_api_key"
# Craft the prompt to encourage a more structured response
prompt = ("Imagine you are an AI tutor. First, briefly explain the concept of "
"machine learning. Then, describe three common types of machine learning.")
response = openai.Completion.create(
engine="text-davinci-002",
prompt=prompt,
max_tokens=200,
n=1,
stop=None,
temperature=0.5,
)
print(response.choices[0].text.strip())
One way to improve the effectiveness of ChatGPT is to experiment with different prompt structures and incorporate control techniques. By doing this, you can gain greater control over the output of ChatGPT and guide it to match specific use cases and requirements.
For example, you might try using more complex prompts that include multiple questions or directions. Another approach could be to use more nuanced control techniques, such as adjusting the response length or using keyword prompts. With these strategies, you can optimize ChatGPT's output to better meet your needs and achieve your desired outcomes.
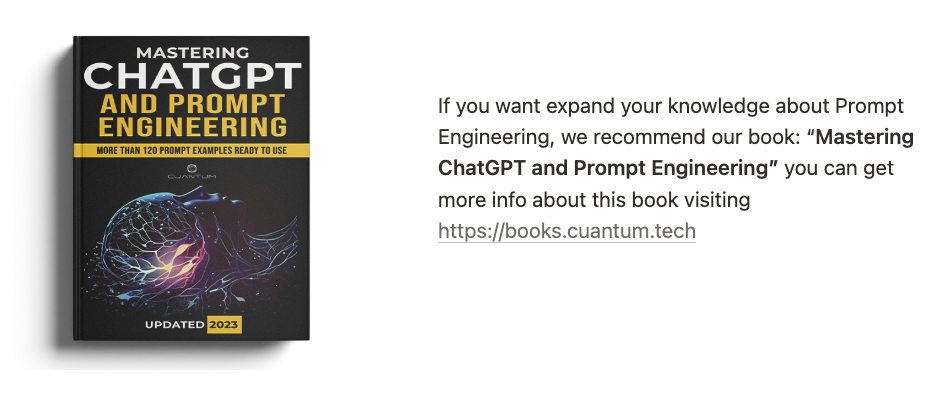
3.2. Controlling the Output
To achieve the best possible results with ChatGPT, it's important to have fine-grained control over the generated output. This allows you to not only adjust the response length, creativity, and sampling strategies, but also to tailor the generated text to your specific needs. By using a combination of various techniques and parameters, you can achieve a wide range of outputs, from short and to-the-point responses to more verbose, elaborated ones that provide more context and detail.
One of the key aspects to consider is the response length. By default, ChatGPT generates responses that are between one and three sentences long, which can be useful for quick, simple interactions. However, in certain contexts, you might need longer responses that provide more information and context. In these cases, you can adjust the length of the generated text by specifying a minimum and maximum length for the output.
Another important parameter to consider is creativity. By default, ChatGPT generates responses that are relatively conservative and safe, in order to avoid generating inappropriate or offensive content. However, in some cases, you might want to increase the creativity of the generated text, to produce more surprising or unexpected outputs. This can be done by adjusting the temperature parameter, which controls the randomness and diversity of the generated text.
Sampling strategies can also play an important role in determining the quality and relevance of the generated text. ChatGPT supports several sampling strategies, including top-k sampling, nucleus sampling, and beam search, each with its own advantages and drawbacks. By experimenting with different sampling techniques, you can find the one that best suits your needs and preferences, and generates the most accurate and relevant responses.
3.2.1. Adjusting Response Length and Creativity
ChatGPT's response length and creativity can be controlled by modifying the max_tokens
, temperature
, and top_p
parameters.
max_tokens
: This parameter sets the maximum number of tokens in the generated response. By increasing or decreasing this value, you can control the length of the output. The max_tokens
parameter determines the maximum number of tokens that the model can generate in its response. A higher value will result in longer responses, while a lower value will result in shorter responses.
temperature
: This parameter influences the randomness of the generated text. A higher temperature value (e.g., 1.0) will make the output more creative and diverse, while a lower value (e.g., 0.1) will make it more focused and deterministic. The temperature
parameter controls the "creativity" of the model. A higher value will result in more surprising and diverse responses, while a lower value will result in more predictable responses.
The top_p
parameter is used to control the diversity of the generated responses by restricting the probability mass to the top p
tokens. A lower value of p
will result in more conservative responses, while a higher value of p
will result in more varied responses.
Here's an explanation of each parameter and an example of how to use them:
Example:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="Write a short introduction to artificial intelligence.",
max_tokens=100, # Adjust the max_tokens value to control response length
n=1,
stop=None,
temperature=0.5, # Adjust the temperature value to control creativity
)
3.2.2. Temperature and Top-k Sampling
There are two primary sampling strategies for controlling the randomness of ChatGPT's output: temperature sampling and top-k sampling.
temperature
: As mentioned earlier, this parameter influences the randomness of the generated text. A higher temperature value will produce more diverse and creative output, while a lower value will generate more focused and deterministic text.
top_k
: This parameter controls the top-k sampling strategy that is used during the text generation process. As you may know, the model selects the next token from the top-k most likely tokens. However, by adjusting the value of top_k
, you can control the diversity of the generated output. In other words, a lower top_k
value will result in a more conservative and predictable output, while a higher top_k
value will result in a more diverse and surprising output.
Therefore, it is important to experiment with different values of top_k
to find the one that best suits your needs. Additionally, keep in mind that other parameters, such as temperature
and length_penalty
, may also affect the quality and diversity of the generated output, so it is important to consider them as well when fine-tuning your model.
Here's an example of using both the temperature
and top_k
parameters:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="Describe the process of photosynthesis.",
max_tokens=150,
n=1,
stop=None,
temperature=0.7, # Adjust the temperature value
top_k=50, # Add the top_k parameter for top-k sampling
)
3.2.3. Using the stop
Parameter
The stop
parameter is a useful feature that enables you to specify a list of tokens at which the API should stop generating text. This can be especially helpful when you want to customize the output structure and ensure that the response ends at a logical point. By using the stop
parameter, you can create more complex and nuanced outputs that better reflect the context and purpose of your text.
Furthermore, the stop
parameter allows you to refine your text generation process by providing more detailed control over the content and structure of your responses. With this powerful tool at your disposal, you can create more engaging, informative, and compelling content that resonates with your audience and achieves your goals.
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="List the benefits of exercise:",
max_tokens=50,
n=1,
stop=["\n"], # Stop generating text at the first newline character
temperature=0.5,
)
3.2.4. Generating Multiple Responses with n
The n
parameter is an extremely useful tool that enables you to generate multiple responses for a single prompt. This is particularly helpful when you want to explore different ideas or provide users with a wide variety of options.
With the n
parameter, you can easily fine-tune the output to generate exactly the kind of content you need. Whether you're looking to brainstorm new ideas or provide users with a range of choices, the n
parameter is an essential tool in your arsenal.
By leveraging this powerful feature, you'll be able to take your content creation to the next level and achieve remarkable results with your audience.
To generate multiple responses, simply set the n
parameter to the desired number of responses:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="What are some creative ways to reuse plastic bottles?",
max_tokens=100,
n=3, # Generate 3 different responses
stop=None,
temperature=0.7,
)
for i, choice in enumerate(response.choices):
print(f"Response {i + 1}:")
print(choice.text.strip())
print()
3.2.5. Prompt Engineering
Prompt engineering involves crafting the input text in such a way that it encourages the model to generate the desired output. One way to do this is by asking the model to think step-by-step. For example, you can break down a complex question into smaller sub-questions, and ask the model to answer each one in sequence.
Alternatively, you could ask the model to debate pros and cons, where it is required to weigh the advantages and disadvantages of a given topic. You could also ask the model to provide a summary before giving a detailed answer, which can help it to focus on the key points and avoid meandering. By using these techniques, you can obtain more focused and relevant responses from ChatGPT, which can be useful in a variety of settings, such as customer service, research, or entertainment.
Example:
import openai
openai.api_key = "your_api_key"
# Craft the prompt to encourage a more structured response
prompt = ("Imagine you are an AI tutor. First, briefly explain the concept of "
"machine learning. Then, describe three common types of machine learning.")
response = openai.Completion.create(
engine="text-davinci-002",
prompt=prompt,
max_tokens=200,
n=1,
stop=None,
temperature=0.5,
)
print(response.choices[0].text.strip())
One way to improve the effectiveness of ChatGPT is to experiment with different prompt structures and incorporate control techniques. By doing this, you can gain greater control over the output of ChatGPT and guide it to match specific use cases and requirements.
For example, you might try using more complex prompts that include multiple questions or directions. Another approach could be to use more nuanced control techniques, such as adjusting the response length or using keyword prompts. With these strategies, you can optimize ChatGPT's output to better meet your needs and achieve your desired outcomes.
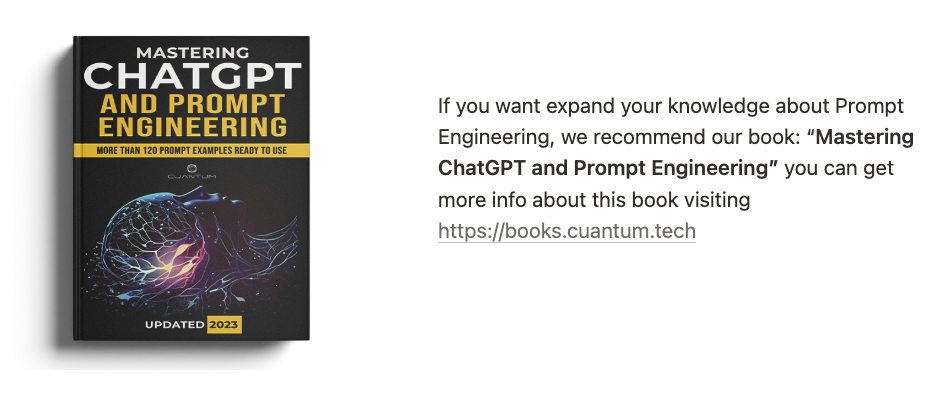
3.2. Controlling the Output
To achieve the best possible results with ChatGPT, it's important to have fine-grained control over the generated output. This allows you to not only adjust the response length, creativity, and sampling strategies, but also to tailor the generated text to your specific needs. By using a combination of various techniques and parameters, you can achieve a wide range of outputs, from short and to-the-point responses to more verbose, elaborated ones that provide more context and detail.
One of the key aspects to consider is the response length. By default, ChatGPT generates responses that are between one and three sentences long, which can be useful for quick, simple interactions. However, in certain contexts, you might need longer responses that provide more information and context. In these cases, you can adjust the length of the generated text by specifying a minimum and maximum length for the output.
Another important parameter to consider is creativity. By default, ChatGPT generates responses that are relatively conservative and safe, in order to avoid generating inappropriate or offensive content. However, in some cases, you might want to increase the creativity of the generated text, to produce more surprising or unexpected outputs. This can be done by adjusting the temperature parameter, which controls the randomness and diversity of the generated text.
Sampling strategies can also play an important role in determining the quality and relevance of the generated text. ChatGPT supports several sampling strategies, including top-k sampling, nucleus sampling, and beam search, each with its own advantages and drawbacks. By experimenting with different sampling techniques, you can find the one that best suits your needs and preferences, and generates the most accurate and relevant responses.
3.2.1. Adjusting Response Length and Creativity
ChatGPT's response length and creativity can be controlled by modifying the max_tokens
, temperature
, and top_p
parameters.
max_tokens
: This parameter sets the maximum number of tokens in the generated response. By increasing or decreasing this value, you can control the length of the output. The max_tokens
parameter determines the maximum number of tokens that the model can generate in its response. A higher value will result in longer responses, while a lower value will result in shorter responses.
temperature
: This parameter influences the randomness of the generated text. A higher temperature value (e.g., 1.0) will make the output more creative and diverse, while a lower value (e.g., 0.1) will make it more focused and deterministic. The temperature
parameter controls the "creativity" of the model. A higher value will result in more surprising and diverse responses, while a lower value will result in more predictable responses.
The top_p
parameter is used to control the diversity of the generated responses by restricting the probability mass to the top p
tokens. A lower value of p
will result in more conservative responses, while a higher value of p
will result in more varied responses.
Here's an explanation of each parameter and an example of how to use them:
Example:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="Write a short introduction to artificial intelligence.",
max_tokens=100, # Adjust the max_tokens value to control response length
n=1,
stop=None,
temperature=0.5, # Adjust the temperature value to control creativity
)
3.2.2. Temperature and Top-k Sampling
There are two primary sampling strategies for controlling the randomness of ChatGPT's output: temperature sampling and top-k sampling.
temperature
: As mentioned earlier, this parameter influences the randomness of the generated text. A higher temperature value will produce more diverse and creative output, while a lower value will generate more focused and deterministic text.
top_k
: This parameter controls the top-k sampling strategy that is used during the text generation process. As you may know, the model selects the next token from the top-k most likely tokens. However, by adjusting the value of top_k
, you can control the diversity of the generated output. In other words, a lower top_k
value will result in a more conservative and predictable output, while a higher top_k
value will result in a more diverse and surprising output.
Therefore, it is important to experiment with different values of top_k
to find the one that best suits your needs. Additionally, keep in mind that other parameters, such as temperature
and length_penalty
, may also affect the quality and diversity of the generated output, so it is important to consider them as well when fine-tuning your model.
Here's an example of using both the temperature
and top_k
parameters:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="Describe the process of photosynthesis.",
max_tokens=150,
n=1,
stop=None,
temperature=0.7, # Adjust the temperature value
top_k=50, # Add the top_k parameter for top-k sampling
)
3.2.3. Using the stop
Parameter
The stop
parameter is a useful feature that enables you to specify a list of tokens at which the API should stop generating text. This can be especially helpful when you want to customize the output structure and ensure that the response ends at a logical point. By using the stop
parameter, you can create more complex and nuanced outputs that better reflect the context and purpose of your text.
Furthermore, the stop
parameter allows you to refine your text generation process by providing more detailed control over the content and structure of your responses. With this powerful tool at your disposal, you can create more engaging, informative, and compelling content that resonates with your audience and achieves your goals.
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="List the benefits of exercise:",
max_tokens=50,
n=1,
stop=["\n"], # Stop generating text at the first newline character
temperature=0.5,
)
3.2.4. Generating Multiple Responses with n
The n
parameter is an extremely useful tool that enables you to generate multiple responses for a single prompt. This is particularly helpful when you want to explore different ideas or provide users with a wide variety of options.
With the n
parameter, you can easily fine-tune the output to generate exactly the kind of content you need. Whether you're looking to brainstorm new ideas or provide users with a range of choices, the n
parameter is an essential tool in your arsenal.
By leveraging this powerful feature, you'll be able to take your content creation to the next level and achieve remarkable results with your audience.
To generate multiple responses, simply set the n
parameter to the desired number of responses:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="What are some creative ways to reuse plastic bottles?",
max_tokens=100,
n=3, # Generate 3 different responses
stop=None,
temperature=0.7,
)
for i, choice in enumerate(response.choices):
print(f"Response {i + 1}:")
print(choice.text.strip())
print()
3.2.5. Prompt Engineering
Prompt engineering involves crafting the input text in such a way that it encourages the model to generate the desired output. One way to do this is by asking the model to think step-by-step. For example, you can break down a complex question into smaller sub-questions, and ask the model to answer each one in sequence.
Alternatively, you could ask the model to debate pros and cons, where it is required to weigh the advantages and disadvantages of a given topic. You could also ask the model to provide a summary before giving a detailed answer, which can help it to focus on the key points and avoid meandering. By using these techniques, you can obtain more focused and relevant responses from ChatGPT, which can be useful in a variety of settings, such as customer service, research, or entertainment.
Example:
import openai
openai.api_key = "your_api_key"
# Craft the prompt to encourage a more structured response
prompt = ("Imagine you are an AI tutor. First, briefly explain the concept of "
"machine learning. Then, describe three common types of machine learning.")
response = openai.Completion.create(
engine="text-davinci-002",
prompt=prompt,
max_tokens=200,
n=1,
stop=None,
temperature=0.5,
)
print(response.choices[0].text.strip())
One way to improve the effectiveness of ChatGPT is to experiment with different prompt structures and incorporate control techniques. By doing this, you can gain greater control over the output of ChatGPT and guide it to match specific use cases and requirements.
For example, you might try using more complex prompts that include multiple questions or directions. Another approach could be to use more nuanced control techniques, such as adjusting the response length or using keyword prompts. With these strategies, you can optimize ChatGPT's output to better meet your needs and achieve your desired outcomes.
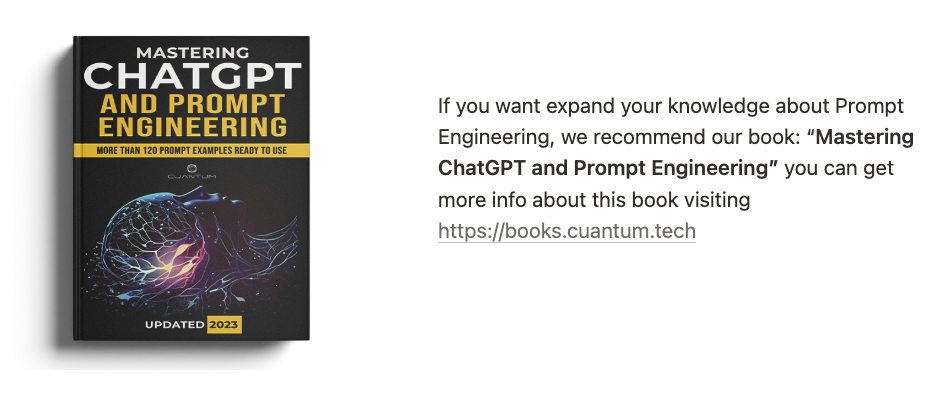
3.2. Controlling the Output
To achieve the best possible results with ChatGPT, it's important to have fine-grained control over the generated output. This allows you to not only adjust the response length, creativity, and sampling strategies, but also to tailor the generated text to your specific needs. By using a combination of various techniques and parameters, you can achieve a wide range of outputs, from short and to-the-point responses to more verbose, elaborated ones that provide more context and detail.
One of the key aspects to consider is the response length. By default, ChatGPT generates responses that are between one and three sentences long, which can be useful for quick, simple interactions. However, in certain contexts, you might need longer responses that provide more information and context. In these cases, you can adjust the length of the generated text by specifying a minimum and maximum length for the output.
Another important parameter to consider is creativity. By default, ChatGPT generates responses that are relatively conservative and safe, in order to avoid generating inappropriate or offensive content. However, in some cases, you might want to increase the creativity of the generated text, to produce more surprising or unexpected outputs. This can be done by adjusting the temperature parameter, which controls the randomness and diversity of the generated text.
Sampling strategies can also play an important role in determining the quality and relevance of the generated text. ChatGPT supports several sampling strategies, including top-k sampling, nucleus sampling, and beam search, each with its own advantages and drawbacks. By experimenting with different sampling techniques, you can find the one that best suits your needs and preferences, and generates the most accurate and relevant responses.
3.2.1. Adjusting Response Length and Creativity
ChatGPT's response length and creativity can be controlled by modifying the max_tokens
, temperature
, and top_p
parameters.
max_tokens
: This parameter sets the maximum number of tokens in the generated response. By increasing or decreasing this value, you can control the length of the output. The max_tokens
parameter determines the maximum number of tokens that the model can generate in its response. A higher value will result in longer responses, while a lower value will result in shorter responses.
temperature
: This parameter influences the randomness of the generated text. A higher temperature value (e.g., 1.0) will make the output more creative and diverse, while a lower value (e.g., 0.1) will make it more focused and deterministic. The temperature
parameter controls the "creativity" of the model. A higher value will result in more surprising and diverse responses, while a lower value will result in more predictable responses.
The top_p
parameter is used to control the diversity of the generated responses by restricting the probability mass to the top p
tokens. A lower value of p
will result in more conservative responses, while a higher value of p
will result in more varied responses.
Here's an explanation of each parameter and an example of how to use them:
Example:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="Write a short introduction to artificial intelligence.",
max_tokens=100, # Adjust the max_tokens value to control response length
n=1,
stop=None,
temperature=0.5, # Adjust the temperature value to control creativity
)
3.2.2. Temperature and Top-k Sampling
There are two primary sampling strategies for controlling the randomness of ChatGPT's output: temperature sampling and top-k sampling.
temperature
: As mentioned earlier, this parameter influences the randomness of the generated text. A higher temperature value will produce more diverse and creative output, while a lower value will generate more focused and deterministic text.
top_k
: This parameter controls the top-k sampling strategy that is used during the text generation process. As you may know, the model selects the next token from the top-k most likely tokens. However, by adjusting the value of top_k
, you can control the diversity of the generated output. In other words, a lower top_k
value will result in a more conservative and predictable output, while a higher top_k
value will result in a more diverse and surprising output.
Therefore, it is important to experiment with different values of top_k
to find the one that best suits your needs. Additionally, keep in mind that other parameters, such as temperature
and length_penalty
, may also affect the quality and diversity of the generated output, so it is important to consider them as well when fine-tuning your model.
Here's an example of using both the temperature
and top_k
parameters:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="Describe the process of photosynthesis.",
max_tokens=150,
n=1,
stop=None,
temperature=0.7, # Adjust the temperature value
top_k=50, # Add the top_k parameter for top-k sampling
)
3.2.3. Using the stop
Parameter
The stop
parameter is a useful feature that enables you to specify a list of tokens at which the API should stop generating text. This can be especially helpful when you want to customize the output structure and ensure that the response ends at a logical point. By using the stop
parameter, you can create more complex and nuanced outputs that better reflect the context and purpose of your text.
Furthermore, the stop
parameter allows you to refine your text generation process by providing more detailed control over the content and structure of your responses. With this powerful tool at your disposal, you can create more engaging, informative, and compelling content that resonates with your audience and achieves your goals.
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="List the benefits of exercise:",
max_tokens=50,
n=1,
stop=["\n"], # Stop generating text at the first newline character
temperature=0.5,
)
3.2.4. Generating Multiple Responses with n
The n
parameter is an extremely useful tool that enables you to generate multiple responses for a single prompt. This is particularly helpful when you want to explore different ideas or provide users with a wide variety of options.
With the n
parameter, you can easily fine-tune the output to generate exactly the kind of content you need. Whether you're looking to brainstorm new ideas or provide users with a range of choices, the n
parameter is an essential tool in your arsenal.
By leveraging this powerful feature, you'll be able to take your content creation to the next level and achieve remarkable results with your audience.
To generate multiple responses, simply set the n
parameter to the desired number of responses:
import openai
openai.api_key = "your_api_key"
response = openai.Completion.create(
engine="text-davinci-002",
prompt="What are some creative ways to reuse plastic bottles?",
max_tokens=100,
n=3, # Generate 3 different responses
stop=None,
temperature=0.7,
)
for i, choice in enumerate(response.choices):
print(f"Response {i + 1}:")
print(choice.text.strip())
print()
3.2.5. Prompt Engineering
Prompt engineering involves crafting the input text in such a way that it encourages the model to generate the desired output. One way to do this is by asking the model to think step-by-step. For example, you can break down a complex question into smaller sub-questions, and ask the model to answer each one in sequence.
Alternatively, you could ask the model to debate pros and cons, where it is required to weigh the advantages and disadvantages of a given topic. You could also ask the model to provide a summary before giving a detailed answer, which can help it to focus on the key points and avoid meandering. By using these techniques, you can obtain more focused and relevant responses from ChatGPT, which can be useful in a variety of settings, such as customer service, research, or entertainment.
Example:
import openai
openai.api_key = "your_api_key"
# Craft the prompt to encourage a more structured response
prompt = ("Imagine you are an AI tutor. First, briefly explain the concept of "
"machine learning. Then, describe three common types of machine learning.")
response = openai.Completion.create(
engine="text-davinci-002",
prompt=prompt,
max_tokens=200,
n=1,
stop=None,
temperature=0.5,
)
print(response.choices[0].text.strip())
One way to improve the effectiveness of ChatGPT is to experiment with different prompt structures and incorporate control techniques. By doing this, you can gain greater control over the output of ChatGPT and guide it to match specific use cases and requirements.
For example, you might try using more complex prompts that include multiple questions or directions. Another approach could be to use more nuanced control techniques, such as adjusting the response length or using keyword prompts. With these strategies, you can optimize ChatGPT's output to better meet your needs and achieve your desired outcomes.
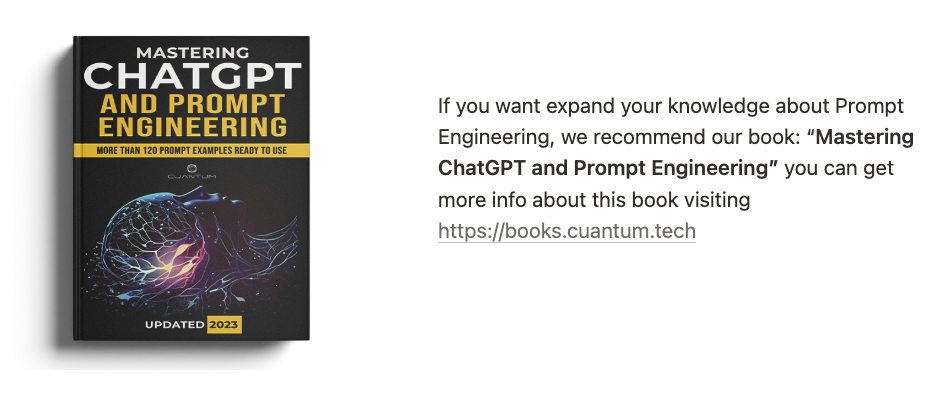