Database automation is a powerful tool that can substantially elevate work efficiency and greatly reduce the burden of manual labor involved in managing databases. It achieves this by automating a number of repetitive tasks that are otherwise time-consuming. These tasks include but are not limited to, data migration, creating backups of data, and the generation of reports.
When Python, a high-level, interpreted programming language, is combined with SQL, a standard language for managing data held in a relational database management system, it results in a robust toolkit. This toolkit is capable of automating a wide range of database operations, making the maintenance of databases a much smoother process.
In this comprehensive guide, we will delve into the details of setting up your automation environment. This involves configuring your system to support the automation of database tasks using Python and SQL. Following this, we will delve into the specifics of writing Python scripts that can handle a variety of common database tasks. These tasks include creating, reading, updating, and deleting records in your database.
Once the scripts have been written, the next part of the process involves scheduling these scripts to run at specific intervals. This ensures that your database remains up-to-date and that any changes to the data are reflected in a timely manner.
The mastery of these techniques will significantly streamline your database management tasks. In addition to making the process more efficient, these skills will also enhance your productivity by freeing up time that would otherwise be spent on manual database management.
Understanding Database Automation
Database automation is the process of utilizing scripts and tools to carry out routine database operations with little to no manual intervention. The operations that can be automated span a wide range of tasks, some of which include:
- Data migration, which involves the seamless transfer of data from one storage type, format, or computer system to another.
- Data backups, which are crucial for data integrity and disaster recovery and can be easily scheduled and performed automatically.
- Report generation, which allows for timely and accurate reporting without the need for manual compilation of data.
- Data cleaning, a process that ensures the accuracy and consistency of data by removing or correcting erroneous entries.
- Scheduled maintenance, which includes tasks such as performance tuning, database updates, and other preventive measures.
The implementation of automation in database management brings about several benefits. It promotes consistency by ensuring that tasks are performed the same way every time, leading to more reliable results. It minimizes the potential for human errors that can occur in manual operations. Furthermore, it liberates database administrators from repetitive tasks, allowing them to focus their time and efforts on more complex and strategic tasks that require human judgment.
Setting Up Your Automation Environment
To automate database operations, you need to set up your Python and SQL environment with the necessary libraries and tools.
Step 1: Install Python
If you don’t have Python installed, download and install it from the official Python website.
Step 2: Install SQL
Choose an SQL database system such as PostgreSQL or MySQL. Follow the installation instructions on their respective websites:
Step 3: Install Necessary Libraries
To make sure that your Python environment has all the necessary libraries, one common method is to use pip
, which is a package management system that is used to install and manage software packages written in Python. So, to get all your needed libraries, you can simply use pip
to install them. This not only saves you a lot of time but also ensures that you have the latest versions of the libraries.
pip install pandas sqlalchemy psycopg2-binary pymysql schedule
This example code uses pip, the package installer for Python, to install several Python packages. These include 'pandas' (for data manipulation and analysis), 'sqlalchemy' (a SQL toolkit and Object-Relational Mapping system), 'psycopg2-binary' (a PostgreSQL database adapter), 'pymysql' (a MySQL database connector), and 'schedule' (a job scheduling library).
Writing Python Scripts for Common Database Tasks
We should endeavour to write Python scripts that can handle a variety of common database tasks. This includes tasks such as data migration, where we would need to safely and efficiently move data from one storage type, format, or computer system to another.
In addition, we should also consider writing scripts for the essential task of data backups, thus ensuring that our important data is duplicated and securely stored to prevent data loss in case of accidental deletions or system failures. Another important task that we can automate with Python scripts is report generation. This would involve writing scripts that can automatically collate, organize, and present data in a human-readable format, making it easier for stakeholders to understand the data and make informed decisions.
Data Migration
Data migration is a key process that involves the movement or transfer of data from one database or repository to another. It is a critical operation that can be required for a variety of reasons, such as upgrading or replacing servers, shifting to a new platform, or just consolidating, archiving, or otherwise managing your data.In essence, it involves the extraction of data from the source system, transforming it into a format that can be understood by the destination system, and then loading it into the destination system. To better illustrate this concept, here's an example script that specifically migrates data from a PostgreSQL database, which is an object-relational database system that uses and extends the SQL language combined with many features that safely store and scale complicated data workloads, to a MySQL database, a renowned open-source relational database management system.
Example: Data Migration Script
from sqlalchemy import create_engine
import pandas as pd
# PostgreSQL database connection
pg_engine = create_engine('postgresql+psycopg2://user:password@localhost:5432/source_db')
# MySQL database connection
mysql_engine = create_engine('mysql+pymysql://user:password@localhost:3306/target_db')
# Read data from PostgreSQL
data = pd.read_sql('SELECT * FROM source_table', pg_engine)
# Write data to MySQL
data.to_sql('target_table', mysql_engine, if_exists='replace', index=False)
print("Data migration completed successfully.")
This example is a Python-based solution for performing data migration tasks, particularly from a PostgreSQL database to a MySQL database. To achieve this functionality, it leverages two powerful Python libraries: SQLAlchemy and pandas.
SQLAlchemy is a SQL toolkit and Object-Relational Mapping (ORM) system for Python, which provides a full suite of well known enterprise-level persistence patterns, designed for efficient and high-performing database access. pandas is a fast, powerful, and flexible open source data analysis and manipulation library for Python, built on top of the Python programming language.
The script begins by importing the necessary libraries. It then establishes connections to both the source PostgreSQL database and the target MySQL database. This is done using the 'create_engine' function from SQLAlchemy, which sets up a standard DBAPI connection.
The connection strings for both databases are specified in the format: dialect+driver://username:password@host:port/database. In this case, 'postgresql+psycopg2' and 'mysql+pymysql' are the dialect and driver for the PostgreSQL and MySQL databases, respectively.
Once the connections to the databases have been established, the script proceeds to read data from a specified table in the PostgreSQL database. This is done using the 'read_sql' function from the pandas library, which executes a SQL query and returns a DataFrame. The query 'SELECT * FROM source_table' is used to select all records from the source table in the PostgreSQL database.
The data read from the PostgreSQL database is then written to a specified table in the MySQL database. This is done using the 'to_sql' function from pandas, which writes records stored in a DataFrame to a SQL database. The 'if_exists' parameter is set to 'replace', which means that if the table already exists, it will be replaced with the new data. The 'index' parameter is set to False, meaning that the DataFrame index will not be written to the MySQL table.
Finally, the script prints a message "Data migration completed successfully." to the console, indicating that the data migration has been completed.
This script offers a simple and efficient method for data migration between PostgreSQL and MySQL databases, and it can be easily modified or extended to accommodate different databases and additional functionalities as needed.
Data Backups
Automating data backups is a strategic measure that provides a robust solution for regularly saving and protecting your database information. This automation process eliminates the need for manual intervention, thereby reducing the risk of human error and ensuring a consistent backup schedule. By automating this critical task, you can ensure that your data is always secure and readily available, providing peace of mind and allowing you to focus on other important aspects of your operations.
Example: Data Backup Script
import datetime
import subprocess
# Define the backup file name with timestamp
backup_file = f"backup_{datetime.datetime.now().strftime('%Y%m%d%H%M%S')}.sql"
# PostgreSQL backup command
backup_command = f"pg_dump -U user -h localhost -d source_db -F c -b -v -f {backup_file}"
# Run the backup command
subprocess.run(backup_command, shell=True)
print(f"Database backup completed: {backup_file}")
This Python script is used to create a backup of a PostgreSQL database. It first defines the name of the backup file, which includes the current timestamp. It then constructs a PostgreSQL backup command using the 'pg_dump' utility, which is used to backup the database. The 'subprocess.run()' function is used to execute this command. After the backup process is completed, it prints a message confirming the completion of the database backup along with the name of the backup file.
Report Generation
The process of automating report generation plays a pivotal role in extracting and compiling regular reports from your database without the need for manual intervention. This approach not only streamlines the process of report creation, but it also eliminates the possibility of human error, thereby enhancing accuracy.
The ability to automatically generate these reports allows businesses to access crucial information in a timely manner, which can then be used to make informed decisions. Hence, the automation of report generation is an essential tool for efficient business management.
Example: Report Generation Script
import pandas as pd
# PostgreSQL database connection
engine = create_engine('postgresql+psycopg2://user:password@localhost:5432/source_db')
# Read data and generate report
data = pd.read_sql('SELECT column1, column2, COUNT(*) as count FROM source_table GROUP BY column1, column2', engine)
# Save report to CSV
report_file = f"report_{datetime.datetime.now().strftime('%Y%m%d%H%M%S')}.csv"
data.to_csv(report_file, index=False)
print(f"Report generated successfully: {report_file}")
This Python script connects to a PostgreSQL database using a connection string. It reads data from a specific table ('source_table') with a SQL query that selects two columns ('column1', 'column2') and counts the number of rows for each unique combination of these columns.
The result is stored in a pandas DataFrame. The script then saves the DataFrame as a CSV file, with a filename that includes the current date and time. Finally, it prints a message to the console indicating that the report has been generated successfully and displays the name of the report file.
Scheduling and Running Automation Scripts
In order to automate the execution of these scripts, there are several scheduling libraries available that you can take advantage of. Two notable examples are schedule
and APScheduler
. These libraries allow you to run your scripts at predetermined intervals, making it easier to manage tasks that need to be executed regularly. This can greatly enhance the efficiency of your programs, and free up your time for other important tasks.
Example: Scheduling with Schedule
The schedule
library allows you to schedule your scripts to run at specific intervals.
Example: Scheduling a Daily Backup
import schedule
import time
import subprocess
def backup_database():
backup_file = f"backup_{datetime.datetime.now().strftime('%Y%m%d%H%M%S')}.sql"
backup_command = f"pg_dump -U user -h localhost -d source_db -F c -b -v -f {backup_file}"
subprocess.run(backup_command, shell=True)
print(f"Database backup completed: {backup_file}")
# Schedule the backup to run daily at 2am
schedule.every().day.at("02:00").do(backup_database)
while True:
schedule.run_pending()
time.sleep(1)
This Python script is designed to automate the process of backing up a database. A function named backup_database
is defined which creates a backup of a PostgreSQL database named 'source_db', hosted on 'localhost' with the username 'user'.
The backup file is named with the current date and time to ensure uniqueness, and the pg_dump
command is used to create the backup. This function is then scheduled to run every day at 2 a.m.
The script enters an infinite loop where it continuously checks and runs any pending scheduled tasks, sleeping for one second between each check.
Example: Scheduling with APScheduler
APScheduler
is a more advanced scheduling library that offers more flexibility and features.
Example: Scheduling a Weekly Report
from apscheduler.schedulers.blocking import BlockingScheduler
import datetime
import pandas as pd
from sqlalchemy import create_engine
def generate_report():
engine = create_engine('postgresql+psycopg2://user:password@localhost:5432/source_db')
data = pd.read_sql('SELECT column1, column2, COUNT(*) as count FROM source_table GROUP BY column1, column2', engine)
report_file = f"report_{datetime.datetime.now().strftime('%Y%m%d%H%M%S')}.csv"
data.to_csv(report_file, index=False)
print(f"Report generated successfully: {report_file}")
scheduler = BlockingScheduler()
scheduler.add_job(generate_report, 'cron', day_of_week='sun', hour=0)
scheduler.start()
The example Python code is a script for scheduling a task that generates a report from a database.
The task involves connecting to a PostgreSQL database using SQLAlchemy and psycopg2, executing a SQL query to retrieve specific data, and then saving the data to a CSV file. The filename is timestamped with the current date and time.
The APScheduler is used here to schedule the task. It is set to run the 'generate_report' function once a week on Sunday at midnight.
Conclusion
The automation of database operations utilizing the capabilities of Python and SQL has the potential to significantly improve efficiency, decrease the volume of manual work, and guarantee uniformity in the execution of your database management tasks.
This is accomplished by properly setting up your working environment, scripting Python for routine tasks, and establishing a schedule for these scripts to be executed automatically. In doing so, the process of managing your database becomes significantly more streamlined and error-free. This not only saves time but also ensures that your database is always up-to-date and accurate, which can have a significant impact on your organization's overall efficiency.
For those interested in expanding their knowledge and skills in this area, we recommend exploring our in-depth guide, "Python and SQL Bible." This resource provides a comprehensive overview of more advanced techniques and a broader understanding of the subject matter.
FAQs
What is database automation?
Database automation involves using scripts and tools to perform routine database operations without manual intervention, such as data migration, backups, and report generation.
Why should I automate database operations?
Automation helps ensure consistency, reduces errors, improves efficiency, and frees up time for more complex tasks.
How do I set up Python and SQL for automation?
Install Python, choose an SQL database system (e.g., PostgreSQL or MySQL), and install necessary Python libraries like pandas
, SQLAlchemy
, psycopg2-binary
, pymysql
, and schedule
.
What are some common database tasks that can be automated?
Common tasks include data migration, data backups, report generation, data cleaning, and scheduled maintenance.
How can I schedule my automation scripts to run automatically?
You can use scheduling libraries like schedule
or APScheduler
to schedule your scripts to run at specific intervals.
Discover "Python and SQL Bible”
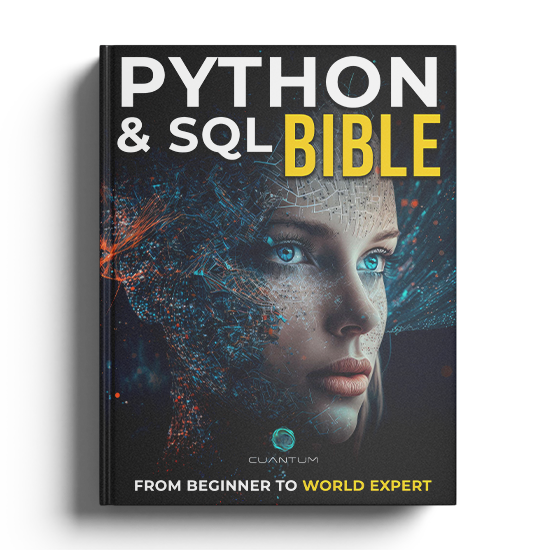
Why Choose This Book?
- Comprehensive Coverage: Covers everything from the basics to advanced techniques, providing a thorough understanding of Python and SQL integration.
- Practical Examples: Includes real-world examples and practical exercises to help you apply what you've learned.
- Detailed Explanations: Breaks down complex topics into easy-to-understand sections, making it accessible for all skill levels.
- Hands-On Exercises: Engage in hands-on exercises at the end of each chapter to reinforce your learning and build confidence.
- Structured Learning Path: Follows a structured learning path that gradually builds your knowledge and skills.
- Automation Techniques: Learn how to automate database operations, reducing manual workload and improving efficiency.
Don't miss out on the opportunity to master Python and SQL. Get your copy of "Python and SQL Bible" today and start transforming your data management and analysis processes!