Chapter 2: Python Building Blocks
2.4 Practice Exercises of Chapter 2: Python Building Blocks
To reinforce the concepts we've discussed in this chapter, try out the following exercises. The solutions are provided after each question, but try to complete the exercises on your own before looking at the answers.
Exercise 1: Create a Python program that takes two numbers as inputs from the user, performs all basic arithmetic operations on these numbers, and prints the results.
Solution:
# taking two numbers as inputs from the user
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
# performing arithmetic operations
add = num1 + num2
subtract = num1 - num2
multiply = num1 * num2
divide = num1 / num2
# printing the results
print(f"The sum of {num1} and {num2} is: {add}")
print(f"The difference between {num1} and {num2} is: {subtract}")
print(f"The product of {num1} and {num2} is: {multiply}")
print(f"The quotient of {num1} and {num2} is: {divide}")
Exercise 2: Create a Python program that asks the user for a number and then prints whether the number is even or odd.
Solution:
# asking the user for a number
num = int(input("Enter a number: "))
# checking whether the number is even or odd
if num % 2 == 0:
print(f"{num} is an even number.")
else:
print(f"{num} is an odd number.")
Exercise 3: Create a Python program that uses comparison operators to compare two numbers provided by the user and prints whether they are equal, and if not, which one is larger.
Solution:
# taking two numbers as inputs from the user
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
# using comparison operators to compare the numbers
if num1 == num2:
print("The numbers are equal.")
elif num1 > num2:
print(f"The number {num1} is larger.")
else:
print(f"The number {num2} is larger.")
Exercise 4: Create a Python program that uses logical operators to determine whether a number input by the user is within a certain range.
Solution:
# taking a number as input from the user
num = float(input("Enter a number: "))
# specifying the range
lower_bound = 10
upper_bound = 20
# checking whether the number is within the range
if num >= lower_bound and num <= upper_bound:
print(f"The number {num} is within the range of {lower_bound} and {upper_bound}.")
else:
print(f"The number {num} is outside the range of {lower_bound} and {upper_bound}.")
These exercises will help you to practice Python syntax, working with variables and different data types, and using Python's basic operators. Always remember that practice is key when learning a new programming language.

If you want to practice Python and eventually become an expert, we recommend our book, "Python Practice Makes a Master: 120 'Real World' Python Exercises with more than 220 Concepts Explained.” It will be a great addition to your journey. You can find it on Amazon.
https://www.amazon.com/dp/B0BZF8R4BZ
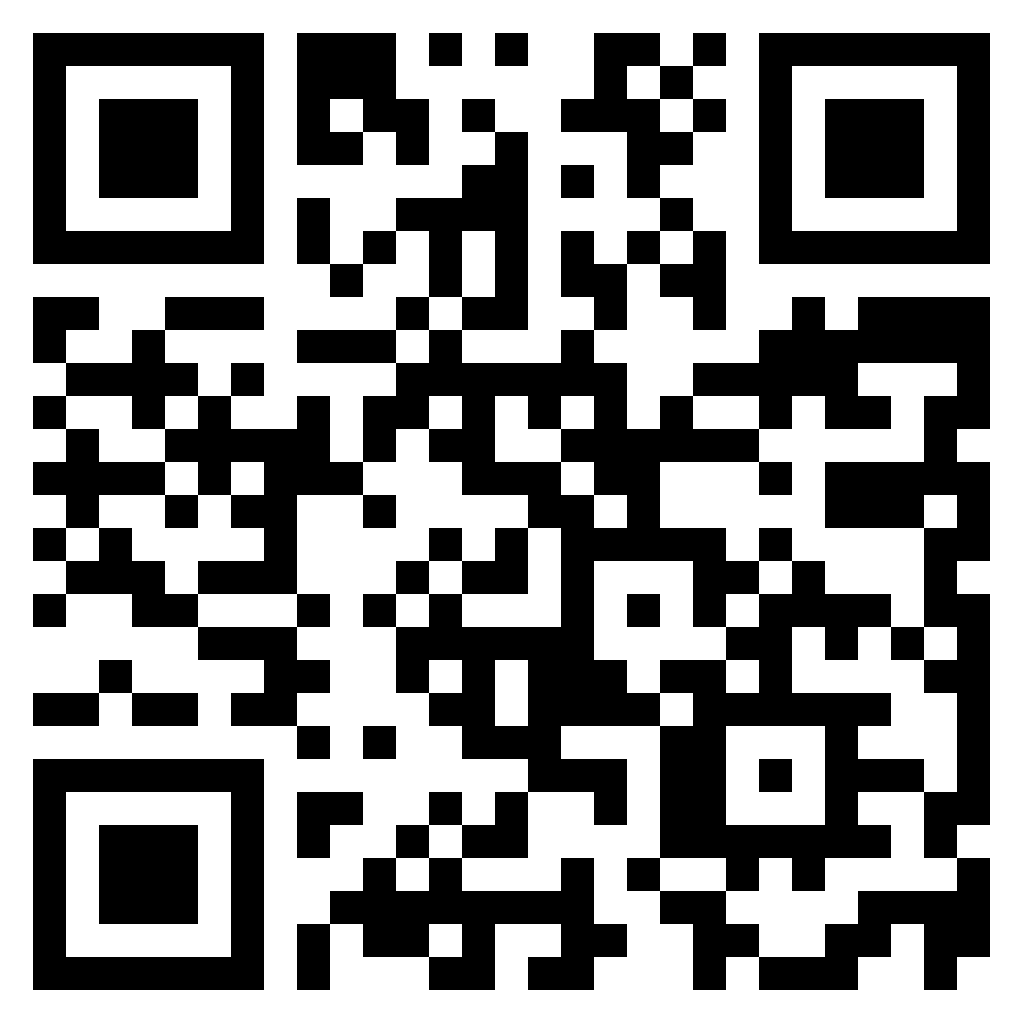
Chapter 2 Conclusion
In this chapter, we have delved deep into the core building blocks of Python, including the language's syntax, semantics, and key programming constructs. We have examined Python's intuitiveness and user-friendly design, both of which have contributed to its rapid rise in popularity among beginners and professionals alike.
Beginning with an exploration of Python's syntax and semantics, we observed the fundamental rules that guide the structure of Python programs. Python's clear, readable syntax makes it a highly expressive language, enabling developers to accomplish complex tasks with comparatively minimal lines of code. Understanding the concepts of indentation and the importance of whitespace, the use of comments, and the correct placement of colons is crucial to writing correct, working Python code. Moreover, diving into Python's semantics, we learned about how Python executes commands and the nuances of the dynamic typing system, demonstrating Python's flexibility.
We then explored the different types of variables and data types in Python. Variables are fundamental to any programming language, and Python offers a variety of data types to suit different needs. We examined several basic data types, including numbers (integers and floats), Booleans, strings, and None, and also looked at more complex types like lists, tuples, dictionaries, and sets. Each type has its unique properties and uses, emphasizing Python's ability to handle a broad range of data manipulation tasks.
In the following sections, we deepened our understanding of Python by exploring the various operators it offers. We covered the basic arithmetic operators for performing mathematical calculations and the comparison operators for making comparisons between values. We also examined the logical operators that allow us to create complex logical conditions. Furthermore, we delved into more advanced concepts like bitwise, membership, and identity operators. Understanding these operators is key to leveraging Python's full potential in data manipulation and decision-making processes.
Finally, we finished the chapter with a series of practical exercises designed to apply and solidify your understanding of the concepts learned. By experimenting and trying out different code snippets, you undoubtedly experienced firsthand the power and simplicity of Python. This hands-on approach is vital for learning and becoming comfortable with a new programming language.
As we conclude this chapter, it's important to remember that while we've covered many fundamental aspects of Python, there is always more to learn. Python is a dynamic, continually evolving language with a rich ecosystem of libraries and frameworks that extend its capabilities. As you continue your journey with Python, you'll discover new features and techniques that will make your code more efficient and effective.
In the upcoming chapters, we will build upon this foundation, exploring more advanced topics like control structures, functions, and object-oriented programming in Python. We'll also delve into Python's powerful libraries for tasks such as data analysis, machine learning, and web development. So, keep practicing, stay curious, and enjoy your journey of mastering Python.
2.4 Practice Exercises of Chapter 2: Python Building Blocks
To reinforce the concepts we've discussed in this chapter, try out the following exercises. The solutions are provided after each question, but try to complete the exercises on your own before looking at the answers.
Exercise 1: Create a Python program that takes two numbers as inputs from the user, performs all basic arithmetic operations on these numbers, and prints the results.
Solution:
# taking two numbers as inputs from the user
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
# performing arithmetic operations
add = num1 + num2
subtract = num1 - num2
multiply = num1 * num2
divide = num1 / num2
# printing the results
print(f"The sum of {num1} and {num2} is: {add}")
print(f"The difference between {num1} and {num2} is: {subtract}")
print(f"The product of {num1} and {num2} is: {multiply}")
print(f"The quotient of {num1} and {num2} is: {divide}")
Exercise 2: Create a Python program that asks the user for a number and then prints whether the number is even or odd.
Solution:
# asking the user for a number
num = int(input("Enter a number: "))
# checking whether the number is even or odd
if num % 2 == 0:
print(f"{num} is an even number.")
else:
print(f"{num} is an odd number.")
Exercise 3: Create a Python program that uses comparison operators to compare two numbers provided by the user and prints whether they are equal, and if not, which one is larger.
Solution:
# taking two numbers as inputs from the user
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
# using comparison operators to compare the numbers
if num1 == num2:
print("The numbers are equal.")
elif num1 > num2:
print(f"The number {num1} is larger.")
else:
print(f"The number {num2} is larger.")
Exercise 4: Create a Python program that uses logical operators to determine whether a number input by the user is within a certain range.
Solution:
# taking a number as input from the user
num = float(input("Enter a number: "))
# specifying the range
lower_bound = 10
upper_bound = 20
# checking whether the number is within the range
if num >= lower_bound and num <= upper_bound:
print(f"The number {num} is within the range of {lower_bound} and {upper_bound}.")
else:
print(f"The number {num} is outside the range of {lower_bound} and {upper_bound}.")
These exercises will help you to practice Python syntax, working with variables and different data types, and using Python's basic operators. Always remember that practice is key when learning a new programming language.

If you want to practice Python and eventually become an expert, we recommend our book, "Python Practice Makes a Master: 120 'Real World' Python Exercises with more than 220 Concepts Explained.” It will be a great addition to your journey. You can find it on Amazon.
https://www.amazon.com/dp/B0BZF8R4BZ
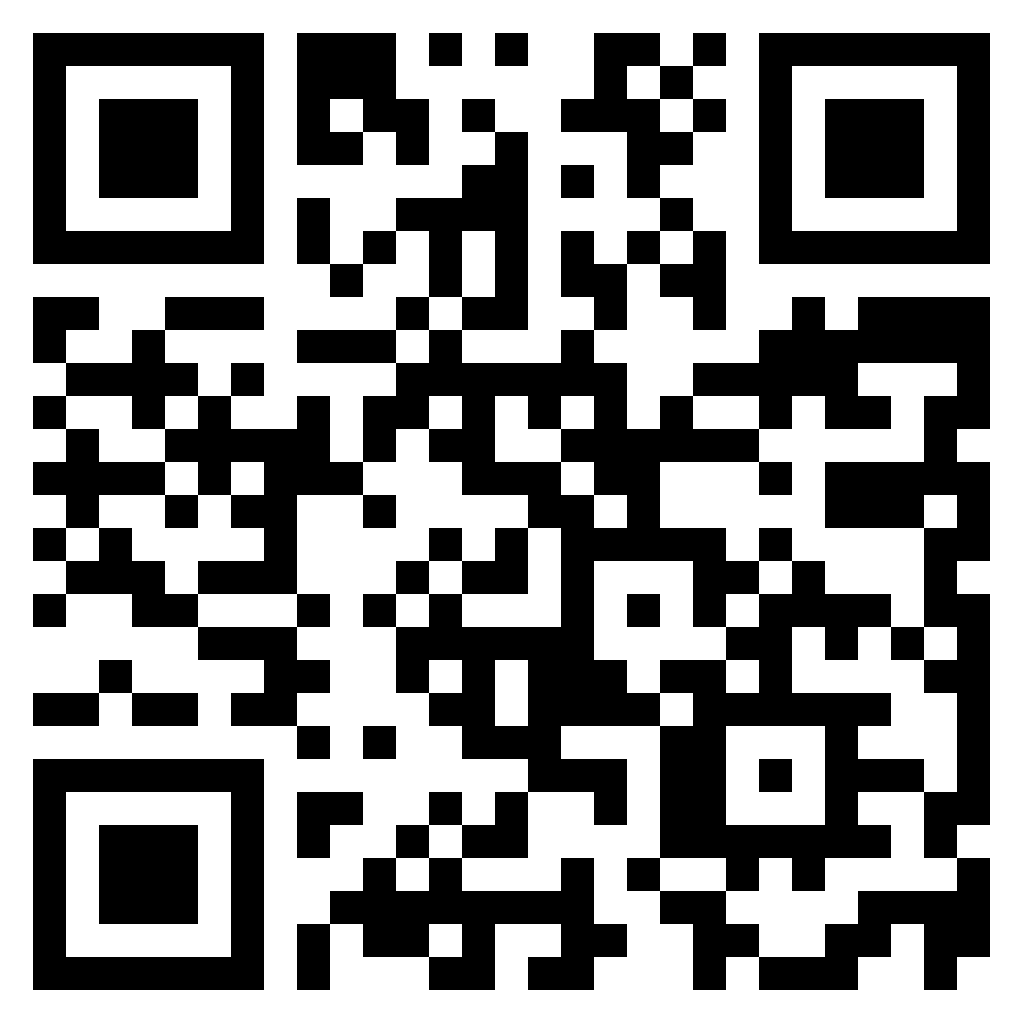
Chapter 2 Conclusion
In this chapter, we have delved deep into the core building blocks of Python, including the language's syntax, semantics, and key programming constructs. We have examined Python's intuitiveness and user-friendly design, both of which have contributed to its rapid rise in popularity among beginners and professionals alike.
Beginning with an exploration of Python's syntax and semantics, we observed the fundamental rules that guide the structure of Python programs. Python's clear, readable syntax makes it a highly expressive language, enabling developers to accomplish complex tasks with comparatively minimal lines of code. Understanding the concepts of indentation and the importance of whitespace, the use of comments, and the correct placement of colons is crucial to writing correct, working Python code. Moreover, diving into Python's semantics, we learned about how Python executes commands and the nuances of the dynamic typing system, demonstrating Python's flexibility.
We then explored the different types of variables and data types in Python. Variables are fundamental to any programming language, and Python offers a variety of data types to suit different needs. We examined several basic data types, including numbers (integers and floats), Booleans, strings, and None, and also looked at more complex types like lists, tuples, dictionaries, and sets. Each type has its unique properties and uses, emphasizing Python's ability to handle a broad range of data manipulation tasks.
In the following sections, we deepened our understanding of Python by exploring the various operators it offers. We covered the basic arithmetic operators for performing mathematical calculations and the comparison operators for making comparisons between values. We also examined the logical operators that allow us to create complex logical conditions. Furthermore, we delved into more advanced concepts like bitwise, membership, and identity operators. Understanding these operators is key to leveraging Python's full potential in data manipulation and decision-making processes.
Finally, we finished the chapter with a series of practical exercises designed to apply and solidify your understanding of the concepts learned. By experimenting and trying out different code snippets, you undoubtedly experienced firsthand the power and simplicity of Python. This hands-on approach is vital for learning and becoming comfortable with a new programming language.
As we conclude this chapter, it's important to remember that while we've covered many fundamental aspects of Python, there is always more to learn. Python is a dynamic, continually evolving language with a rich ecosystem of libraries and frameworks that extend its capabilities. As you continue your journey with Python, you'll discover new features and techniques that will make your code more efficient and effective.
In the upcoming chapters, we will build upon this foundation, exploring more advanced topics like control structures, functions, and object-oriented programming in Python. We'll also delve into Python's powerful libraries for tasks such as data analysis, machine learning, and web development. So, keep practicing, stay curious, and enjoy your journey of mastering Python.
2.4 Practice Exercises of Chapter 2: Python Building Blocks
To reinforce the concepts we've discussed in this chapter, try out the following exercises. The solutions are provided after each question, but try to complete the exercises on your own before looking at the answers.
Exercise 1: Create a Python program that takes two numbers as inputs from the user, performs all basic arithmetic operations on these numbers, and prints the results.
Solution:
# taking two numbers as inputs from the user
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
# performing arithmetic operations
add = num1 + num2
subtract = num1 - num2
multiply = num1 * num2
divide = num1 / num2
# printing the results
print(f"The sum of {num1} and {num2} is: {add}")
print(f"The difference between {num1} and {num2} is: {subtract}")
print(f"The product of {num1} and {num2} is: {multiply}")
print(f"The quotient of {num1} and {num2} is: {divide}")
Exercise 2: Create a Python program that asks the user for a number and then prints whether the number is even or odd.
Solution:
# asking the user for a number
num = int(input("Enter a number: "))
# checking whether the number is even or odd
if num % 2 == 0:
print(f"{num} is an even number.")
else:
print(f"{num} is an odd number.")
Exercise 3: Create a Python program that uses comparison operators to compare two numbers provided by the user and prints whether they are equal, and if not, which one is larger.
Solution:
# taking two numbers as inputs from the user
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
# using comparison operators to compare the numbers
if num1 == num2:
print("The numbers are equal.")
elif num1 > num2:
print(f"The number {num1} is larger.")
else:
print(f"The number {num2} is larger.")
Exercise 4: Create a Python program that uses logical operators to determine whether a number input by the user is within a certain range.
Solution:
# taking a number as input from the user
num = float(input("Enter a number: "))
# specifying the range
lower_bound = 10
upper_bound = 20
# checking whether the number is within the range
if num >= lower_bound and num <= upper_bound:
print(f"The number {num} is within the range of {lower_bound} and {upper_bound}.")
else:
print(f"The number {num} is outside the range of {lower_bound} and {upper_bound}.")
These exercises will help you to practice Python syntax, working with variables and different data types, and using Python's basic operators. Always remember that practice is key when learning a new programming language.

If you want to practice Python and eventually become an expert, we recommend our book, "Python Practice Makes a Master: 120 'Real World' Python Exercises with more than 220 Concepts Explained.” It will be a great addition to your journey. You can find it on Amazon.
https://www.amazon.com/dp/B0BZF8R4BZ
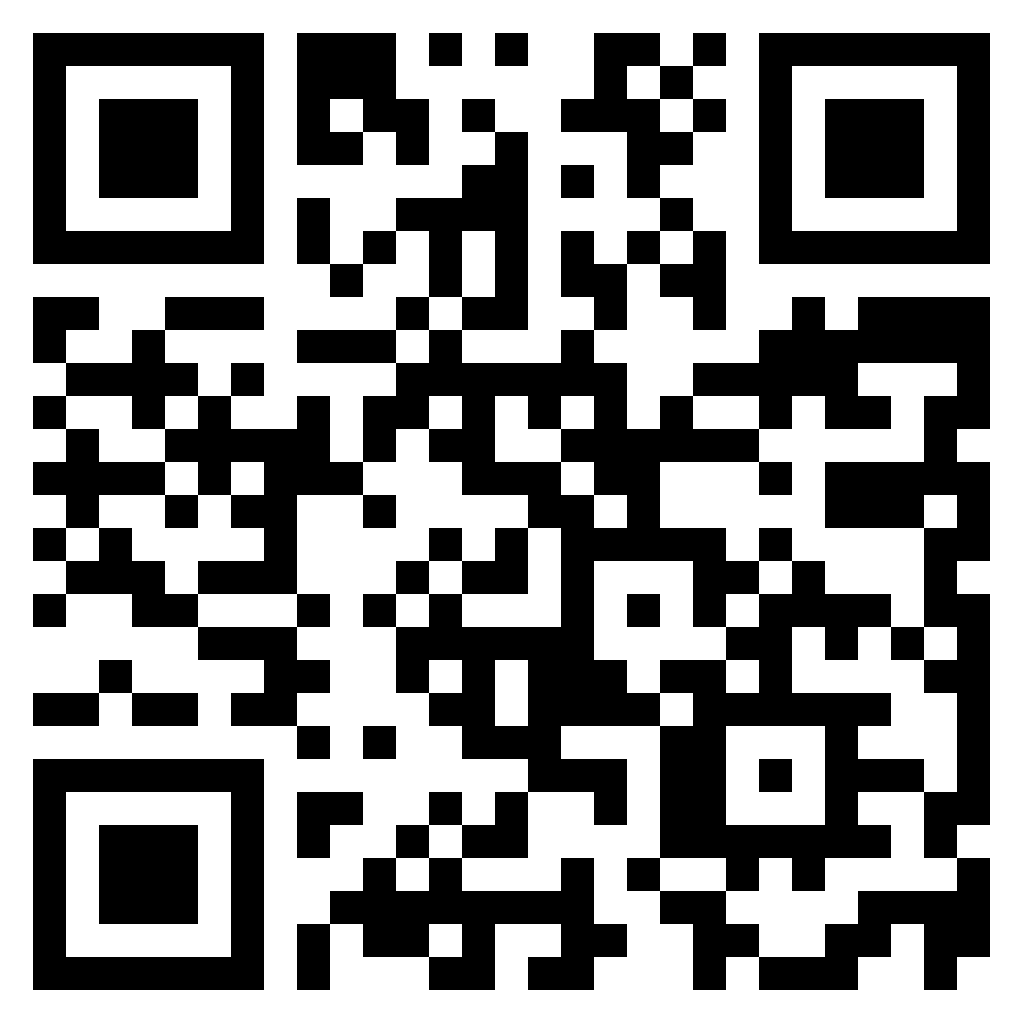
Chapter 2 Conclusion
In this chapter, we have delved deep into the core building blocks of Python, including the language's syntax, semantics, and key programming constructs. We have examined Python's intuitiveness and user-friendly design, both of which have contributed to its rapid rise in popularity among beginners and professionals alike.
Beginning with an exploration of Python's syntax and semantics, we observed the fundamental rules that guide the structure of Python programs. Python's clear, readable syntax makes it a highly expressive language, enabling developers to accomplish complex tasks with comparatively minimal lines of code. Understanding the concepts of indentation and the importance of whitespace, the use of comments, and the correct placement of colons is crucial to writing correct, working Python code. Moreover, diving into Python's semantics, we learned about how Python executes commands and the nuances of the dynamic typing system, demonstrating Python's flexibility.
We then explored the different types of variables and data types in Python. Variables are fundamental to any programming language, and Python offers a variety of data types to suit different needs. We examined several basic data types, including numbers (integers and floats), Booleans, strings, and None, and also looked at more complex types like lists, tuples, dictionaries, and sets. Each type has its unique properties and uses, emphasizing Python's ability to handle a broad range of data manipulation tasks.
In the following sections, we deepened our understanding of Python by exploring the various operators it offers. We covered the basic arithmetic operators for performing mathematical calculations and the comparison operators for making comparisons between values. We also examined the logical operators that allow us to create complex logical conditions. Furthermore, we delved into more advanced concepts like bitwise, membership, and identity operators. Understanding these operators is key to leveraging Python's full potential in data manipulation and decision-making processes.
Finally, we finished the chapter with a series of practical exercises designed to apply and solidify your understanding of the concepts learned. By experimenting and trying out different code snippets, you undoubtedly experienced firsthand the power and simplicity of Python. This hands-on approach is vital for learning and becoming comfortable with a new programming language.
As we conclude this chapter, it's important to remember that while we've covered many fundamental aspects of Python, there is always more to learn. Python is a dynamic, continually evolving language with a rich ecosystem of libraries and frameworks that extend its capabilities. As you continue your journey with Python, you'll discover new features and techniques that will make your code more efficient and effective.
In the upcoming chapters, we will build upon this foundation, exploring more advanced topics like control structures, functions, and object-oriented programming in Python. We'll also delve into Python's powerful libraries for tasks such as data analysis, machine learning, and web development. So, keep practicing, stay curious, and enjoy your journey of mastering Python.
2.4 Practice Exercises of Chapter 2: Python Building Blocks
To reinforce the concepts we've discussed in this chapter, try out the following exercises. The solutions are provided after each question, but try to complete the exercises on your own before looking at the answers.
Exercise 1: Create a Python program that takes two numbers as inputs from the user, performs all basic arithmetic operations on these numbers, and prints the results.
Solution:
# taking two numbers as inputs from the user
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
# performing arithmetic operations
add = num1 + num2
subtract = num1 - num2
multiply = num1 * num2
divide = num1 / num2
# printing the results
print(f"The sum of {num1} and {num2} is: {add}")
print(f"The difference between {num1} and {num2} is: {subtract}")
print(f"The product of {num1} and {num2} is: {multiply}")
print(f"The quotient of {num1} and {num2} is: {divide}")
Exercise 2: Create a Python program that asks the user for a number and then prints whether the number is even or odd.
Solution:
# asking the user for a number
num = int(input("Enter a number: "))
# checking whether the number is even or odd
if num % 2 == 0:
print(f"{num} is an even number.")
else:
print(f"{num} is an odd number.")
Exercise 3: Create a Python program that uses comparison operators to compare two numbers provided by the user and prints whether they are equal, and if not, which one is larger.
Solution:
# taking two numbers as inputs from the user
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
# using comparison operators to compare the numbers
if num1 == num2:
print("The numbers are equal.")
elif num1 > num2:
print(f"The number {num1} is larger.")
else:
print(f"The number {num2} is larger.")
Exercise 4: Create a Python program that uses logical operators to determine whether a number input by the user is within a certain range.
Solution:
# taking a number as input from the user
num = float(input("Enter a number: "))
# specifying the range
lower_bound = 10
upper_bound = 20
# checking whether the number is within the range
if num >= lower_bound and num <= upper_bound:
print(f"The number {num} is within the range of {lower_bound} and {upper_bound}.")
else:
print(f"The number {num} is outside the range of {lower_bound} and {upper_bound}.")
These exercises will help you to practice Python syntax, working with variables and different data types, and using Python's basic operators. Always remember that practice is key when learning a new programming language.

If you want to practice Python and eventually become an expert, we recommend our book, "Python Practice Makes a Master: 120 'Real World' Python Exercises with more than 220 Concepts Explained.” It will be a great addition to your journey. You can find it on Amazon.
https://www.amazon.com/dp/B0BZF8R4BZ
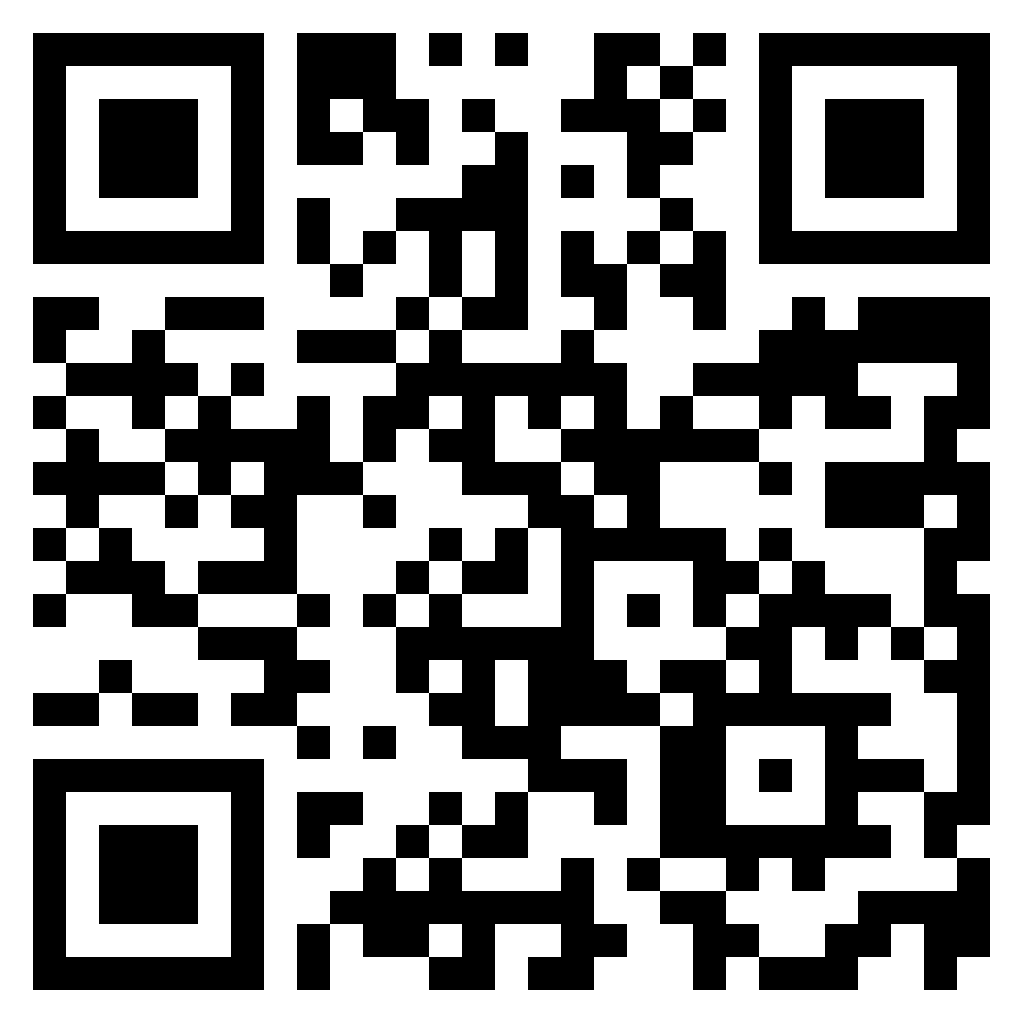
Chapter 2 Conclusion
In this chapter, we have delved deep into the core building blocks of Python, including the language's syntax, semantics, and key programming constructs. We have examined Python's intuitiveness and user-friendly design, both of which have contributed to its rapid rise in popularity among beginners and professionals alike.
Beginning with an exploration of Python's syntax and semantics, we observed the fundamental rules that guide the structure of Python programs. Python's clear, readable syntax makes it a highly expressive language, enabling developers to accomplish complex tasks with comparatively minimal lines of code. Understanding the concepts of indentation and the importance of whitespace, the use of comments, and the correct placement of colons is crucial to writing correct, working Python code. Moreover, diving into Python's semantics, we learned about how Python executes commands and the nuances of the dynamic typing system, demonstrating Python's flexibility.
We then explored the different types of variables and data types in Python. Variables are fundamental to any programming language, and Python offers a variety of data types to suit different needs. We examined several basic data types, including numbers (integers and floats), Booleans, strings, and None, and also looked at more complex types like lists, tuples, dictionaries, and sets. Each type has its unique properties and uses, emphasizing Python's ability to handle a broad range of data manipulation tasks.
In the following sections, we deepened our understanding of Python by exploring the various operators it offers. We covered the basic arithmetic operators for performing mathematical calculations and the comparison operators for making comparisons between values. We also examined the logical operators that allow us to create complex logical conditions. Furthermore, we delved into more advanced concepts like bitwise, membership, and identity operators. Understanding these operators is key to leveraging Python's full potential in data manipulation and decision-making processes.
Finally, we finished the chapter with a series of practical exercises designed to apply and solidify your understanding of the concepts learned. By experimenting and trying out different code snippets, you undoubtedly experienced firsthand the power and simplicity of Python. This hands-on approach is vital for learning and becoming comfortable with a new programming language.
As we conclude this chapter, it's important to remember that while we've covered many fundamental aspects of Python, there is always more to learn. Python is a dynamic, continually evolving language with a rich ecosystem of libraries and frameworks that extend its capabilities. As you continue your journey with Python, you'll discover new features and techniques that will make your code more efficient and effective.
In the upcoming chapters, we will build upon this foundation, exploring more advanced topics like control structures, functions, and object-oriented programming in Python. We'll also delve into Python's powerful libraries for tasks such as data analysis, machine learning, and web development. So, keep practicing, stay curious, and enjoy your journey of mastering Python.