Algorithms and Data Structures with Python
Unleash Python's power! Master efficient code with algorithms & data structures like lists, trees, & searches. This book is your guide to Python programming proficiency.
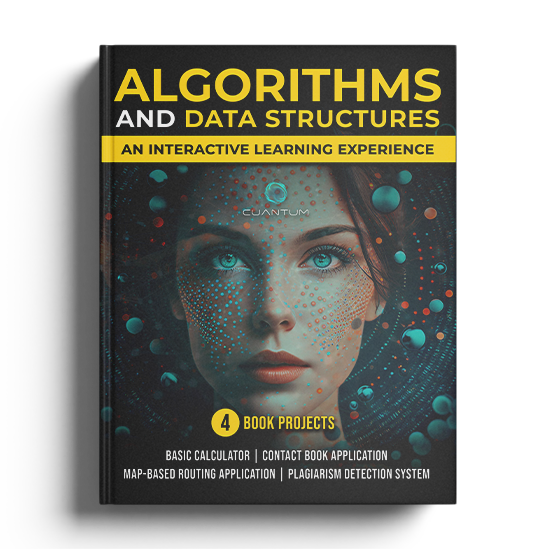
Why you should have this book
Level up your coding skills
Build strong coding abilities & tackle projects with confidence.
Become a confident programmer
Grasp key concepts & avoid common pitfalls. Be unstoppable.
Solid foundation
Learn once, code anywhere. Unlock your programming potential.
Master Algorithms and Data Structures with Python

Unlock the power of problem-solving and data manipulation with "Algorithms and Data Structures with Python." This comprehensive guide equips you with the essential knowledge and skills to design efficient algorithms and implement powerful data structures using Python, a versatile and widely used programming language.
Through engaging explanations, practical exercises, and real-world applications, you'll gain a thorough understanding of fundamental concepts like:
- Searching and Sorting Algorithms: Explore efficient techniques for finding and organizing data, from basic linear search to sophisticated sorting algorithms like Merge Sort and Quick Sort.
- Data Structures: Delve into the world of different data structures like stacks, queues, linked lists, and trees, each tailored for specific tasks and optimizing storage and retrieval of information.
- Graph Algorithms: Understand how to represent and manipulate relationships between data points using graph structures, opening doors to solving problems like shortest path finding and network analysis.
Build Efficient Algorithms and Data Structures in Python
This practical guide takes a hands-on approach, focusing on building your skills through engaging exercises and real-world projects. You'll explore:
- Designing algorithms: Learn how to break down complex problems into smaller, manageable steps, formulating efficient algorithms that minimize execution time and resource usage.
- Implementing data structures: Discover how to choose the right data structure for the job, from simple arrays to complex trees and graphs, ensuring optimal storage, retrieval, and manipulation of your data.
- Optimizing code performance: Understand how to analyze and optimize your code for efficiency, minimizing unnecessary calculations and maximizing resource utilization.

In today's data-driven world, efficiency and organization are key. "Build Efficient Algorithms and Data Structures in Python" empowers you to become a master of data manipulation by equipping you with the skills to design and implement efficient algorithms and powerful data structures using Python.
By mastering these crucial components, you'll be well-equipped to tackle complex programming challenges, optimize code performance, and design efficient solutions for various real-world applications. This book empowers you to not only understand these concepts but also confidently apply them to real-world scenarios, setting you apart as a programmer with a strong foundation in problem-solving and data manipulation.
By the end of this journey, you'll be fully prepared to build efficient and well-structured code, confidently addressing complex problems. Whether you're a data scientist, software developer, or simply curious about efficient data manipulation, this book offers the essential skills and knowledge needed to excel in today's data-focused world.
Table of contents
Chapter 1: Python & Algorithms: An Introduction
1.1 Why algorithms and data structures?
1.2 The Evolution of Programming
1.3 The Synergy Between Python and Algorithms
1.4 Python's Role in Algorithm Development
Chapter 1: Practical Exercises of Python & Algorithms: An Introduction
Chapter 2: Diving into Python
2.1 Python Syntax Essentials
2.2 Data Types and Operators
2.3 Control Structures and Functions
Chapter 2: Practical Exercises of Diving into Python
Chapter 2 Summary of Diving into Python
Chapter 3: Elementary Data Containers
3.1 Lists, Tuples, Sets, and Dictionaries
3.2 OOP: Classes, Objects, and Encapsulation
3.3 Stacks, Queues, and their Applications
3.4 Linked Lists: Understanding Pointers and Nodes, and Their Applications
Chapter 3: Practical Exercises of Elementary Data Containers
Quiz Part I: Python Foundations and Basic Data Structures
Questions of Quiz Part I: Python Foundations and Basic Data Structures
Answers of Quiz Part I: Python Foundations and Basic Data Structures
Project 1: Basic Calculator
1. Setting Up the Main Framework
2. Implementing Arithmetic Functions
3. Integrating Arithmetic Functions with Main Framework
4. Enhancing User Experience
5. Adding Advanced Arithmetic Functions
Chapter 4: The Art of Sorting
4.1 Basic Sorting Algorithms: Bubble, Selection, Insertion
4.2 Advanced Sorting: Delving Deeper
4.3 Time Complexity and Performance Analysis
Chapter 4: Practical Exercises of The Art of Sorting
Chapter 4 Summary of The Art of Sorting
Chapter 5: Search Operations & Efficiency
5.1 Linear vs. Binary Search
5.2 Introduction to Hashing and Its Efficiency
5.3 Time Complexity and Big O Notation
Chapter 5: Practical Exercises of Search Operations & Efficiency
Chapter 5 Summary of Search Operations & Efficiency
Chapter 6: Trees and Graphs: Hierarchical Data Structures
6.1 Trees: Types and Traversal Techniques
6.2 Graphs: Representation and Basic Algorithms
6.3 Hash Tables: Implementation and Collision Resolution
Chapter 6: Practical Exercises of Trees and Graphs: Hierarchical Data Structures
Chapter 6 Summary of Trees and Graphs: Hierarchical Data Structures
Quiz Part II: Sorting, Searching, and Hierarchical Structures
Questions of Quiz Part II: Sorting, Searching, and Hierarchical Structures
Answers of Quiz Part II: Sorting, Searching, and Hierarchical Structures
Project 2: Contact Book Application
Implementing the Basic Structure
Adding Search Functionality
Adding Delete Functionality
Listing All Contacts
Conclusion and Future Enhancements of Project 2: Contact Book Application
Chapter 7: Mastering Algorithmic Techniques
7.1 The Philosophy of Divide and Conquer
7.2 Saving Time with Dynamic Programming
7.3 The Greedy Approach and Backtracking
Chapter 7: Practical Exercises of Mastering Algorithmic Techniques
Chapter 7 Summary of Mastering Algorithmic Techniques
Chapter 8: Networks and Paths: Advanced Graph Algorithms
8.1 Diving Deeper into Graph Theory
8.2 Algorithms for Shortest Paths, Flows, and Connectivity
8.3 Network Optimization and Advanced Graph Techniques
Chapter 8: Practical Exercises of Networks and Paths: Advanced Graph Algorithms
Chapter 8 Summary of Networks and Paths: Advanced Graph Algorithms
Quiz Part III: Advanced Algorithmic Techniques and Network Structures
Questions of Quiz Part III: Advanced Algorithmic Techniques and Network Structures
Answers of Quiz Part III: Advanced Algorithmic Techniques and Network Structures
Project 3: Map-based Routing Application
Setting Up the Graph for the Map
Implementing Dijkstra's Algorithm
User Interaction and Input Handling
Handling Real-World Map Data
Graphical Interface for Visualization (Optional)
Chapter 9: Deciphering Strings and Patterns
9.1 Basics of String Algorithms
9.2 Pattern Searching, Tries, and Suffix Trees
9.3 Advanced Pattern Matching and Text Analysis Techniques
Chapter 9: Practical Exercises of Deciphering Strings and Patterns
Chapter 9 Summary of Deciphering Strings and Patterns
Chapter 10: Venturing into Advanced Computational Problems
10.1 Unraveling NP-hard and NP-complete Classes
10.2 Approaches to Approximation and Randomized Algorithms
10.3 Advanced Algorithms in Graph Theory and Network Analysis
Chapter 10: Practical Exercises of Venturing into Advanced Computational Problems
Chapter 10 Summary of Venturing into Advanced Computational Problems
Chapter 11: From Theory to Practice. Case Studies & Optimizations
11.1 Case Studies: Real-world Algorithmic Solutions
11.2 Python Performance Considerations and Enhancements
Chapter 11: Practical Exercises of From Theory to Practice. Case Studies & Optimizations
Chapter 11 Summary of From Theory to Practice. Case Studies & Optimizations
Quiz Part IV: String Manipulation, Advanced Concepts, and Practical Applications
Questions of Quiz Part IV: String Manipulation, Advanced Concepts, and Practical Applications
Answers of Quiz Part IV: String Manipulation, Advanced Concepts, and Practical Applications
Project 4: Plagiarism Detection System
Building the Foundation: Text Preprocessing and Similarity Measurement
Handling Larger Documents and Paragraph-Level Analysis
Incorporating Advanced Text Analysis Techniques
Conclusion and Future Directions of Project 4: Plagiarism Detection System
What our readers are saying about this book
Explore the reviews to understand why this book is a great choice! Discover how others have gained from the knowledge and insights it provides. Get a taste of the exciting content that awaits you and see if this book is the perfect fit for your journey.
Excellent writing! As a non-tech person, I was nervous to read this book, but wanted to expand my horizons. I was pleasantly surprised how accessible it is! There is wonderful metaphorical language to help “tech muggles” like me grasp foreign concepts. This book is very well done and can help beginning and advanced coders alike. Well done!
Algorithms and Data Structures with Python" isn't your typical textbook; it's like a guide on a thrilling journey of discovery. What makes it stand out is its focus on real-world applications, turning each chapter into an interactive experience with practical exercises and projects mirroring industry challenges. You can feel the author's passion as they stress the importance of grasping algorithms in today's data-driven landscape. The incorporation of Python makes this exploration accessible whether you're a student, a professional, or just a curious mind.
Unlock Access
Is your choice, paperback, eBook, or a Full Access Pass to our entire library
- Paperback shipped from Amazon
- Free code repository access
- Premium customer support
- Digital eLearning platform
- Free additional video content
- Cost-effective
- Premium customer support
- Easy copy-paste code resources
- Learn anywhere
- Everything from Book Access
- Unlimited Book Library Access
- 50% Off on Paperback Books
- Early Access to New Launches
- Exclusive Video Content
- Monthly Book Recommendations
- Unlimited book updates
- 24/7 VIP Customer Support
- Programming Challenges